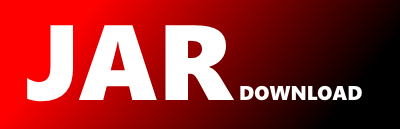
com.pulumi.azurenative.batch.outputs.VirtualMachineConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.batch.outputs;
import com.pulumi.azurenative.batch.outputs.ContainerConfigurationResponse;
import com.pulumi.azurenative.batch.outputs.DataDiskResponse;
import com.pulumi.azurenative.batch.outputs.DiskEncryptionConfigurationResponse;
import com.pulumi.azurenative.batch.outputs.ImageReferenceResponse;
import com.pulumi.azurenative.batch.outputs.NodePlacementConfigurationResponse;
import com.pulumi.azurenative.batch.outputs.OSDiskResponse;
import com.pulumi.azurenative.batch.outputs.VMExtensionResponse;
import com.pulumi.azurenative.batch.outputs.WindowsConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualMachineConfigurationResponse {
/**
* @return If specified, setup is performed on each node in the pool to allow tasks to run in containers. All regular tasks and job manager tasks run on this pool must specify the containerSettings property, and all other tasks may specify it.
*
*/
private @Nullable ContainerConfigurationResponse containerConfiguration;
/**
* @return This property must be specified if the compute nodes in the pool need to have empty data disks attached to them.
*
*/
private @Nullable List dataDisks;
/**
* @return If specified, encryption is performed on each node in the pool during node provisioning.
*
*/
private @Nullable DiskEncryptionConfigurationResponse diskEncryptionConfiguration;
/**
* @return If specified, the extensions mentioned in this configuration will be installed on each node.
*
*/
private @Nullable List extensions;
private ImageReferenceResponse imageReference;
/**
* @return This only applies to images that contain the Windows operating system, and should only be used when you hold valid on-premises licenses for the nodes which will be deployed. If omitted, no on-premises licensing discount is applied. Values are:
*
* Windows_Server - The on-premises license is for Windows Server.
* Windows_Client - The on-premises license is for Windows Client.
*
*/
private @Nullable String licenseType;
/**
* @return The Batch node agent is a program that runs on each node in the pool, and provides the command-and-control interface between the node and the Batch service. There are different implementations of the node agent, known as SKUs, for different operating systems. You must specify a node agent SKU which matches the selected image reference. To get the list of supported node agent SKUs along with their list of verified image references, see the 'List supported node agent SKUs' operation.
*
*/
private String nodeAgentSkuId;
/**
* @return This configuration will specify rules on how nodes in the pool will be physically allocated.
*
*/
private @Nullable NodePlacementConfigurationResponse nodePlacementConfiguration;
/**
* @return Contains configuration for ephemeral OSDisk settings.
*
*/
private @Nullable OSDiskResponse osDisk;
/**
* @return This property must not be specified if the imageReference specifies a Linux OS image.
*
*/
private @Nullable WindowsConfigurationResponse windowsConfiguration;
private VirtualMachineConfigurationResponse() {}
/**
* @return If specified, setup is performed on each node in the pool to allow tasks to run in containers. All regular tasks and job manager tasks run on this pool must specify the containerSettings property, and all other tasks may specify it.
*
*/
public Optional containerConfiguration() {
return Optional.ofNullable(this.containerConfiguration);
}
/**
* @return This property must be specified if the compute nodes in the pool need to have empty data disks attached to them.
*
*/
public List dataDisks() {
return this.dataDisks == null ? List.of() : this.dataDisks;
}
/**
* @return If specified, encryption is performed on each node in the pool during node provisioning.
*
*/
public Optional diskEncryptionConfiguration() {
return Optional.ofNullable(this.diskEncryptionConfiguration);
}
/**
* @return If specified, the extensions mentioned in this configuration will be installed on each node.
*
*/
public List extensions() {
return this.extensions == null ? List.of() : this.extensions;
}
public ImageReferenceResponse imageReference() {
return this.imageReference;
}
/**
* @return This only applies to images that contain the Windows operating system, and should only be used when you hold valid on-premises licenses for the nodes which will be deployed. If omitted, no on-premises licensing discount is applied. Values are:
*
* Windows_Server - The on-premises license is for Windows Server.
* Windows_Client - The on-premises license is for Windows Client.
*
*/
public Optional licenseType() {
return Optional.ofNullable(this.licenseType);
}
/**
* @return The Batch node agent is a program that runs on each node in the pool, and provides the command-and-control interface between the node and the Batch service. There are different implementations of the node agent, known as SKUs, for different operating systems. You must specify a node agent SKU which matches the selected image reference. To get the list of supported node agent SKUs along with their list of verified image references, see the 'List supported node agent SKUs' operation.
*
*/
public String nodeAgentSkuId() {
return this.nodeAgentSkuId;
}
/**
* @return This configuration will specify rules on how nodes in the pool will be physically allocated.
*
*/
public Optional nodePlacementConfiguration() {
return Optional.ofNullable(this.nodePlacementConfiguration);
}
/**
* @return Contains configuration for ephemeral OSDisk settings.
*
*/
public Optional osDisk() {
return Optional.ofNullable(this.osDisk);
}
/**
* @return This property must not be specified if the imageReference specifies a Linux OS image.
*
*/
public Optional windowsConfiguration() {
return Optional.ofNullable(this.windowsConfiguration);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualMachineConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ContainerConfigurationResponse containerConfiguration;
private @Nullable List dataDisks;
private @Nullable DiskEncryptionConfigurationResponse diskEncryptionConfiguration;
private @Nullable List extensions;
private ImageReferenceResponse imageReference;
private @Nullable String licenseType;
private String nodeAgentSkuId;
private @Nullable NodePlacementConfigurationResponse nodePlacementConfiguration;
private @Nullable OSDiskResponse osDisk;
private @Nullable WindowsConfigurationResponse windowsConfiguration;
public Builder() {}
public Builder(VirtualMachineConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.containerConfiguration = defaults.containerConfiguration;
this.dataDisks = defaults.dataDisks;
this.diskEncryptionConfiguration = defaults.diskEncryptionConfiguration;
this.extensions = defaults.extensions;
this.imageReference = defaults.imageReference;
this.licenseType = defaults.licenseType;
this.nodeAgentSkuId = defaults.nodeAgentSkuId;
this.nodePlacementConfiguration = defaults.nodePlacementConfiguration;
this.osDisk = defaults.osDisk;
this.windowsConfiguration = defaults.windowsConfiguration;
}
@CustomType.Setter
public Builder containerConfiguration(@Nullable ContainerConfigurationResponse containerConfiguration) {
this.containerConfiguration = containerConfiguration;
return this;
}
@CustomType.Setter
public Builder dataDisks(@Nullable List dataDisks) {
this.dataDisks = dataDisks;
return this;
}
public Builder dataDisks(DataDiskResponse... dataDisks) {
return dataDisks(List.of(dataDisks));
}
@CustomType.Setter
public Builder diskEncryptionConfiguration(@Nullable DiskEncryptionConfigurationResponse diskEncryptionConfiguration) {
this.diskEncryptionConfiguration = diskEncryptionConfiguration;
return this;
}
@CustomType.Setter
public Builder extensions(@Nullable List extensions) {
this.extensions = extensions;
return this;
}
public Builder extensions(VMExtensionResponse... extensions) {
return extensions(List.of(extensions));
}
@CustomType.Setter
public Builder imageReference(ImageReferenceResponse imageReference) {
if (imageReference == null) {
throw new MissingRequiredPropertyException("VirtualMachineConfigurationResponse", "imageReference");
}
this.imageReference = imageReference;
return this;
}
@CustomType.Setter
public Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@CustomType.Setter
public Builder nodeAgentSkuId(String nodeAgentSkuId) {
if (nodeAgentSkuId == null) {
throw new MissingRequiredPropertyException("VirtualMachineConfigurationResponse", "nodeAgentSkuId");
}
this.nodeAgentSkuId = nodeAgentSkuId;
return this;
}
@CustomType.Setter
public Builder nodePlacementConfiguration(@Nullable NodePlacementConfigurationResponse nodePlacementConfiguration) {
this.nodePlacementConfiguration = nodePlacementConfiguration;
return this;
}
@CustomType.Setter
public Builder osDisk(@Nullable OSDiskResponse osDisk) {
this.osDisk = osDisk;
return this;
}
@CustomType.Setter
public Builder windowsConfiguration(@Nullable WindowsConfigurationResponse windowsConfiguration) {
this.windowsConfiguration = windowsConfiguration;
return this;
}
public VirtualMachineConfigurationResponse build() {
final var _resultValue = new VirtualMachineConfigurationResponse();
_resultValue.containerConfiguration = containerConfiguration;
_resultValue.dataDisks = dataDisks;
_resultValue.diskEncryptionConfiguration = diskEncryptionConfiguration;
_resultValue.extensions = extensions;
_resultValue.imageReference = imageReference;
_resultValue.licenseType = licenseType;
_resultValue.nodeAgentSkuId = nodeAgentSkuId;
_resultValue.nodePlacementConfiguration = nodePlacementConfiguration;
_resultValue.osDisk = osDisk;
_resultValue.windowsConfiguration = windowsConfiguration;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy