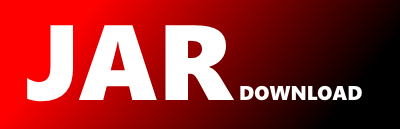
com.pulumi.azurenative.botservice.inputs.WebChatSiteArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A site for the Webchat channel
*
*/
public final class WebChatSiteArgs extends com.pulumi.resources.ResourceArgs {
public static final WebChatSiteArgs Empty = new WebChatSiteArgs();
/**
* DirectLine application id
*
*/
@Import(name="appId")
private @Nullable Output appId;
/**
* @return DirectLine application id
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy