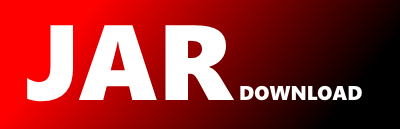
com.pulumi.azurenative.botservice.outputs.SkypeChannelPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SkypeChannelPropertiesResponse {
/**
* @return Calling web hook for Skype channel
*
*/
private @Nullable String callingWebHook;
/**
* @return Enable calling for Skype channel
*
*/
private @Nullable Boolean enableCalling;
/**
* @return Enable groups for Skype channel
*
*/
private @Nullable Boolean enableGroups;
/**
* @return Enable media cards for Skype channel
*
*/
private @Nullable Boolean enableMediaCards;
/**
* @return Enable messaging for Skype channel
*
*/
private @Nullable Boolean enableMessaging;
/**
* @return Enable screen sharing for Skype channel
*
*/
private @Nullable Boolean enableScreenSharing;
/**
* @return Enable video for Skype channel
*
*/
private @Nullable Boolean enableVideo;
/**
* @return Group mode for Skype channel
*
*/
private @Nullable String groupsMode;
/**
* @return Incoming call route for Skype channel
*
*/
private @Nullable String incomingCallRoute;
/**
* @return Whether this channel is enabled for the bot
*
*/
private Boolean isEnabled;
private SkypeChannelPropertiesResponse() {}
/**
* @return Calling web hook for Skype channel
*
*/
public Optional callingWebHook() {
return Optional.ofNullable(this.callingWebHook);
}
/**
* @return Enable calling for Skype channel
*
*/
public Optional enableCalling() {
return Optional.ofNullable(this.enableCalling);
}
/**
* @return Enable groups for Skype channel
*
*/
public Optional enableGroups() {
return Optional.ofNullable(this.enableGroups);
}
/**
* @return Enable media cards for Skype channel
*
*/
public Optional enableMediaCards() {
return Optional.ofNullable(this.enableMediaCards);
}
/**
* @return Enable messaging for Skype channel
*
*/
public Optional enableMessaging() {
return Optional.ofNullable(this.enableMessaging);
}
/**
* @return Enable screen sharing for Skype channel
*
*/
public Optional enableScreenSharing() {
return Optional.ofNullable(this.enableScreenSharing);
}
/**
* @return Enable video for Skype channel
*
*/
public Optional enableVideo() {
return Optional.ofNullable(this.enableVideo);
}
/**
* @return Group mode for Skype channel
*
*/
public Optional groupsMode() {
return Optional.ofNullable(this.groupsMode);
}
/**
* @return Incoming call route for Skype channel
*
*/
public Optional incomingCallRoute() {
return Optional.ofNullable(this.incomingCallRoute);
}
/**
* @return Whether this channel is enabled for the bot
*
*/
public Boolean isEnabled() {
return this.isEnabled;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SkypeChannelPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String callingWebHook;
private @Nullable Boolean enableCalling;
private @Nullable Boolean enableGroups;
private @Nullable Boolean enableMediaCards;
private @Nullable Boolean enableMessaging;
private @Nullable Boolean enableScreenSharing;
private @Nullable Boolean enableVideo;
private @Nullable String groupsMode;
private @Nullable String incomingCallRoute;
private Boolean isEnabled;
public Builder() {}
public Builder(SkypeChannelPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.callingWebHook = defaults.callingWebHook;
this.enableCalling = defaults.enableCalling;
this.enableGroups = defaults.enableGroups;
this.enableMediaCards = defaults.enableMediaCards;
this.enableMessaging = defaults.enableMessaging;
this.enableScreenSharing = defaults.enableScreenSharing;
this.enableVideo = defaults.enableVideo;
this.groupsMode = defaults.groupsMode;
this.incomingCallRoute = defaults.incomingCallRoute;
this.isEnabled = defaults.isEnabled;
}
@CustomType.Setter
public Builder callingWebHook(@Nullable String callingWebHook) {
this.callingWebHook = callingWebHook;
return this;
}
@CustomType.Setter
public Builder enableCalling(@Nullable Boolean enableCalling) {
this.enableCalling = enableCalling;
return this;
}
@CustomType.Setter
public Builder enableGroups(@Nullable Boolean enableGroups) {
this.enableGroups = enableGroups;
return this;
}
@CustomType.Setter
public Builder enableMediaCards(@Nullable Boolean enableMediaCards) {
this.enableMediaCards = enableMediaCards;
return this;
}
@CustomType.Setter
public Builder enableMessaging(@Nullable Boolean enableMessaging) {
this.enableMessaging = enableMessaging;
return this;
}
@CustomType.Setter
public Builder enableScreenSharing(@Nullable Boolean enableScreenSharing) {
this.enableScreenSharing = enableScreenSharing;
return this;
}
@CustomType.Setter
public Builder enableVideo(@Nullable Boolean enableVideo) {
this.enableVideo = enableVideo;
return this;
}
@CustomType.Setter
public Builder groupsMode(@Nullable String groupsMode) {
this.groupsMode = groupsMode;
return this;
}
@CustomType.Setter
public Builder incomingCallRoute(@Nullable String incomingCallRoute) {
this.incomingCallRoute = incomingCallRoute;
return this;
}
@CustomType.Setter
public Builder isEnabled(Boolean isEnabled) {
if (isEnabled == null) {
throw new MissingRequiredPropertyException("SkypeChannelPropertiesResponse", "isEnabled");
}
this.isEnabled = isEnabled;
return this;
}
public SkypeChannelPropertiesResponse build() {
final var _resultValue = new SkypeChannelPropertiesResponse();
_resultValue.callingWebHook = callingWebHook;
_resultValue.enableCalling = enableCalling;
_resultValue.enableGroups = enableGroups;
_resultValue.enableMediaCards = enableMediaCards;
_resultValue.enableMessaging = enableMessaging;
_resultValue.enableScreenSharing = enableScreenSharing;
_resultValue.enableVideo = enableVideo;
_resultValue.groupsMode = groupsMode;
_resultValue.incomingCallRoute = incomingCallRoute;
_resultValue.isEnabled = isEnabled;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy