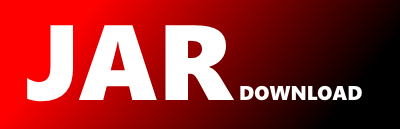
com.pulumi.azurenative.botservice.outputs.WebChatSiteResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class WebChatSiteResponse {
/**
* @return DirectLine application id
*
*/
private @Nullable String appId;
/**
* @return Entity Tag
*
*/
private @Nullable String eTag;
/**
* @return Whether this site is enabled for block user upload.
*
*/
private @Nullable Boolean isBlockUserUploadEnabled;
/**
* @return Whether this site is disabled detailed logging for
*
*/
private @Nullable Boolean isDetailedLoggingEnabled;
/**
* @return Whether this site is enabled for DirectLine channel
*
*/
private Boolean isEnabled;
/**
* @return Whether this site is EndpointParameters enabled for channel
*
*/
private @Nullable Boolean isEndpointParametersEnabled;
/**
* @return Whether this no-storage site is disabled detailed logging for
*
*/
private @Nullable Boolean isNoStorageEnabled;
/**
* @return Whether this site is enabled for authentication with Bot Framework.
*
*/
private @Nullable Boolean isSecureSiteEnabled;
/**
* @return Whether this site is token enabled for channel
*
*/
private Boolean isTokenEnabled;
/**
* @return Whether this site is enabled for Bot Framework V1 protocol.
*
*/
private @Nullable Boolean isV1Enabled;
/**
* @return Whether this site is enabled for Bot Framework V3 protocol.
*
*/
private @Nullable Boolean isV3Enabled;
/**
* @return Whether this site is enabled for Webchat Speech
*
*/
private @Nullable Boolean isWebChatSpeechEnabled;
/**
* @return Whether this site is enabled for preview versions of Webchat
*
*/
private Boolean isWebchatPreviewEnabled;
/**
* @return Primary key. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
private String key;
/**
* @return Secondary key. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
private String key2;
/**
* @return Site Id
*
*/
private String siteId;
/**
* @return Site name
*
*/
private String siteName;
/**
* @return Tenant Id
*
*/
private @Nullable String tenantId;
/**
* @return List of Trusted Origin URLs for this site. This field is applicable only if isSecureSiteEnabled is True.
*
*/
private @Nullable List trustedOrigins;
private WebChatSiteResponse() {}
/**
* @return DirectLine application id
*
*/
public Optional appId() {
return Optional.ofNullable(this.appId);
}
/**
* @return Entity Tag
*
*/
public Optional eTag() {
return Optional.ofNullable(this.eTag);
}
/**
* @return Whether this site is enabled for block user upload.
*
*/
public Optional isBlockUserUploadEnabled() {
return Optional.ofNullable(this.isBlockUserUploadEnabled);
}
/**
* @return Whether this site is disabled detailed logging for
*
*/
public Optional isDetailedLoggingEnabled() {
return Optional.ofNullable(this.isDetailedLoggingEnabled);
}
/**
* @return Whether this site is enabled for DirectLine channel
*
*/
public Boolean isEnabled() {
return this.isEnabled;
}
/**
* @return Whether this site is EndpointParameters enabled for channel
*
*/
public Optional isEndpointParametersEnabled() {
return Optional.ofNullable(this.isEndpointParametersEnabled);
}
/**
* @return Whether this no-storage site is disabled detailed logging for
*
*/
public Optional isNoStorageEnabled() {
return Optional.ofNullable(this.isNoStorageEnabled);
}
/**
* @return Whether this site is enabled for authentication with Bot Framework.
*
*/
public Optional isSecureSiteEnabled() {
return Optional.ofNullable(this.isSecureSiteEnabled);
}
/**
* @return Whether this site is token enabled for channel
*
*/
public Boolean isTokenEnabled() {
return this.isTokenEnabled;
}
/**
* @return Whether this site is enabled for Bot Framework V1 protocol.
*
*/
public Optional isV1Enabled() {
return Optional.ofNullable(this.isV1Enabled);
}
/**
* @return Whether this site is enabled for Bot Framework V3 protocol.
*
*/
public Optional isV3Enabled() {
return Optional.ofNullable(this.isV3Enabled);
}
/**
* @return Whether this site is enabled for Webchat Speech
*
*/
public Optional isWebChatSpeechEnabled() {
return Optional.ofNullable(this.isWebChatSpeechEnabled);
}
/**
* @return Whether this site is enabled for preview versions of Webchat
*
*/
public Boolean isWebchatPreviewEnabled() {
return this.isWebchatPreviewEnabled;
}
/**
* @return Primary key. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
public String key() {
return this.key;
}
/**
* @return Secondary key. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
public String key2() {
return this.key2;
}
/**
* @return Site Id
*
*/
public String siteId() {
return this.siteId;
}
/**
* @return Site name
*
*/
public String siteName() {
return this.siteName;
}
/**
* @return Tenant Id
*
*/
public Optional tenantId() {
return Optional.ofNullable(this.tenantId);
}
/**
* @return List of Trusted Origin URLs for this site. This field is applicable only if isSecureSiteEnabled is True.
*
*/
public List trustedOrigins() {
return this.trustedOrigins == null ? List.of() : this.trustedOrigins;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WebChatSiteResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String appId;
private @Nullable String eTag;
private @Nullable Boolean isBlockUserUploadEnabled;
private @Nullable Boolean isDetailedLoggingEnabled;
private Boolean isEnabled;
private @Nullable Boolean isEndpointParametersEnabled;
private @Nullable Boolean isNoStorageEnabled;
private @Nullable Boolean isSecureSiteEnabled;
private Boolean isTokenEnabled;
private @Nullable Boolean isV1Enabled;
private @Nullable Boolean isV3Enabled;
private @Nullable Boolean isWebChatSpeechEnabled;
private Boolean isWebchatPreviewEnabled;
private String key;
private String key2;
private String siteId;
private String siteName;
private @Nullable String tenantId;
private @Nullable List trustedOrigins;
public Builder() {}
public Builder(WebChatSiteResponse defaults) {
Objects.requireNonNull(defaults);
this.appId = defaults.appId;
this.eTag = defaults.eTag;
this.isBlockUserUploadEnabled = defaults.isBlockUserUploadEnabled;
this.isDetailedLoggingEnabled = defaults.isDetailedLoggingEnabled;
this.isEnabled = defaults.isEnabled;
this.isEndpointParametersEnabled = defaults.isEndpointParametersEnabled;
this.isNoStorageEnabled = defaults.isNoStorageEnabled;
this.isSecureSiteEnabled = defaults.isSecureSiteEnabled;
this.isTokenEnabled = defaults.isTokenEnabled;
this.isV1Enabled = defaults.isV1Enabled;
this.isV3Enabled = defaults.isV3Enabled;
this.isWebChatSpeechEnabled = defaults.isWebChatSpeechEnabled;
this.isWebchatPreviewEnabled = defaults.isWebchatPreviewEnabled;
this.key = defaults.key;
this.key2 = defaults.key2;
this.siteId = defaults.siteId;
this.siteName = defaults.siteName;
this.tenantId = defaults.tenantId;
this.trustedOrigins = defaults.trustedOrigins;
}
@CustomType.Setter
public Builder appId(@Nullable String appId) {
this.appId = appId;
return this;
}
@CustomType.Setter
public Builder eTag(@Nullable String eTag) {
this.eTag = eTag;
return this;
}
@CustomType.Setter
public Builder isBlockUserUploadEnabled(@Nullable Boolean isBlockUserUploadEnabled) {
this.isBlockUserUploadEnabled = isBlockUserUploadEnabled;
return this;
}
@CustomType.Setter
public Builder isDetailedLoggingEnabled(@Nullable Boolean isDetailedLoggingEnabled) {
this.isDetailedLoggingEnabled = isDetailedLoggingEnabled;
return this;
}
@CustomType.Setter
public Builder isEnabled(Boolean isEnabled) {
if (isEnabled == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "isEnabled");
}
this.isEnabled = isEnabled;
return this;
}
@CustomType.Setter
public Builder isEndpointParametersEnabled(@Nullable Boolean isEndpointParametersEnabled) {
this.isEndpointParametersEnabled = isEndpointParametersEnabled;
return this;
}
@CustomType.Setter
public Builder isNoStorageEnabled(@Nullable Boolean isNoStorageEnabled) {
this.isNoStorageEnabled = isNoStorageEnabled;
return this;
}
@CustomType.Setter
public Builder isSecureSiteEnabled(@Nullable Boolean isSecureSiteEnabled) {
this.isSecureSiteEnabled = isSecureSiteEnabled;
return this;
}
@CustomType.Setter
public Builder isTokenEnabled(Boolean isTokenEnabled) {
if (isTokenEnabled == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "isTokenEnabled");
}
this.isTokenEnabled = isTokenEnabled;
return this;
}
@CustomType.Setter
public Builder isV1Enabled(@Nullable Boolean isV1Enabled) {
this.isV1Enabled = isV1Enabled;
return this;
}
@CustomType.Setter
public Builder isV3Enabled(@Nullable Boolean isV3Enabled) {
this.isV3Enabled = isV3Enabled;
return this;
}
@CustomType.Setter
public Builder isWebChatSpeechEnabled(@Nullable Boolean isWebChatSpeechEnabled) {
this.isWebChatSpeechEnabled = isWebChatSpeechEnabled;
return this;
}
@CustomType.Setter
public Builder isWebchatPreviewEnabled(Boolean isWebchatPreviewEnabled) {
if (isWebchatPreviewEnabled == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "isWebchatPreviewEnabled");
}
this.isWebchatPreviewEnabled = isWebchatPreviewEnabled;
return this;
}
@CustomType.Setter
public Builder key(String key) {
if (key == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "key");
}
this.key = key;
return this;
}
@CustomType.Setter
public Builder key2(String key2) {
if (key2 == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "key2");
}
this.key2 = key2;
return this;
}
@CustomType.Setter
public Builder siteId(String siteId) {
if (siteId == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "siteId");
}
this.siteId = siteId;
return this;
}
@CustomType.Setter
public Builder siteName(String siteName) {
if (siteName == null) {
throw new MissingRequiredPropertyException("WebChatSiteResponse", "siteName");
}
this.siteName = siteName;
return this;
}
@CustomType.Setter
public Builder tenantId(@Nullable String tenantId) {
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder trustedOrigins(@Nullable List trustedOrigins) {
this.trustedOrigins = trustedOrigins;
return this;
}
public Builder trustedOrigins(String... trustedOrigins) {
return trustedOrigins(List.of(trustedOrigins));
}
public WebChatSiteResponse build() {
final var _resultValue = new WebChatSiteResponse();
_resultValue.appId = appId;
_resultValue.eTag = eTag;
_resultValue.isBlockUserUploadEnabled = isBlockUserUploadEnabled;
_resultValue.isDetailedLoggingEnabled = isDetailedLoggingEnabled;
_resultValue.isEnabled = isEnabled;
_resultValue.isEndpointParametersEnabled = isEndpointParametersEnabled;
_resultValue.isNoStorageEnabled = isNoStorageEnabled;
_resultValue.isSecureSiteEnabled = isSecureSiteEnabled;
_resultValue.isTokenEnabled = isTokenEnabled;
_resultValue.isV1Enabled = isV1Enabled;
_resultValue.isV3Enabled = isV3Enabled;
_resultValue.isWebChatSpeechEnabled = isWebChatSpeechEnabled;
_resultValue.isWebchatPreviewEnabled = isWebchatPreviewEnabled;
_resultValue.key = key;
_resultValue.key2 = key2;
_resultValue.siteId = siteId;
_resultValue.siteName = siteName;
_resultValue.tenantId = tenantId;
_resultValue.trustedOrigins = trustedOrigins;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy