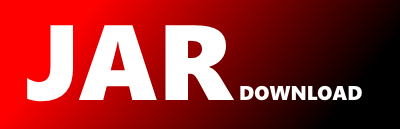
com.pulumi.azurenative.cdn.RouteArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn;
import com.pulumi.azurenative.cdn.enums.AFDEndpointProtocols;
import com.pulumi.azurenative.cdn.enums.EnabledState;
import com.pulumi.azurenative.cdn.enums.ForwardingProtocol;
import com.pulumi.azurenative.cdn.enums.HttpsRedirect;
import com.pulumi.azurenative.cdn.enums.LinkToDefaultDomain;
import com.pulumi.azurenative.cdn.inputs.ActivatedResourceReferenceArgs;
import com.pulumi.azurenative.cdn.inputs.AfdRouteCacheConfigurationArgs;
import com.pulumi.azurenative.cdn.inputs.ResourceReferenceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RouteArgs extends com.pulumi.resources.ResourceArgs {
public static final RouteArgs Empty = new RouteArgs();
/**
* The caching configuration for this route. To disable caching, do not provide a cacheConfiguration object.
*
*/
@Import(name="cacheConfiguration")
private @Nullable Output cacheConfiguration;
/**
* @return The caching configuration for this route. To disable caching, do not provide a cacheConfiguration object.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy