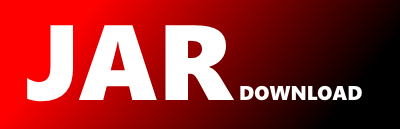
com.pulumi.azurenative.cdn.inputs.CacheConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn.inputs;
import com.pulumi.azurenative.cdn.enums.RuleCacheBehavior;
import com.pulumi.azurenative.cdn.enums.RuleIsCompressionEnabled;
import com.pulumi.azurenative.cdn.enums.RuleQueryStringCachingBehavior;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Caching settings for a caching-type route. To disable caching, do not provide a cacheConfiguration object.
*
*/
public final class CacheConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final CacheConfigurationArgs Empty = new CacheConfigurationArgs();
/**
* Caching behavior for the requests
*
*/
@Import(name="cacheBehavior")
private @Nullable Output> cacheBehavior;
/**
* @return Caching behavior for the requests
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy