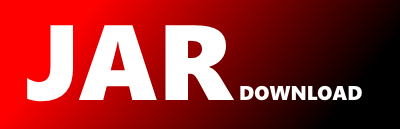
com.pulumi.azurenative.cdn.inputs.RemoteAddressMatchConditionParametersArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn.inputs;
import com.pulumi.azurenative.cdn.enums.RemoteAddressOperator;
import com.pulumi.azurenative.cdn.enums.Transform;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Defines the parameters for RemoteAddress match conditions
*
*/
public final class RemoteAddressMatchConditionParametersArgs extends com.pulumi.resources.ResourceArgs {
public static final RemoteAddressMatchConditionParametersArgs Empty = new RemoteAddressMatchConditionParametersArgs();
/**
* Match values to match against. The operator will apply to each value in here with OR semantics. If any of them match the variable with the given operator this match condition is considered a match.
*
*/
@Import(name="matchValues")
private @Nullable Output> matchValues;
/**
* @return Match values to match against. The operator will apply to each value in here with OR semantics. If any of them match the variable with the given operator this match condition is considered a match.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy