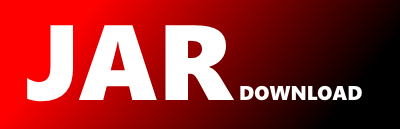
com.pulumi.azurenative.cdn.outputs.GetRouteResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn.outputs;
import com.pulumi.azurenative.cdn.outputs.ActivatedResourceReferenceResponse;
import com.pulumi.azurenative.cdn.outputs.AfdRouteCacheConfigurationResponse;
import com.pulumi.azurenative.cdn.outputs.ResourceReferenceResponse;
import com.pulumi.azurenative.cdn.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetRouteResult {
/**
* @return The caching configuration for this route. To disable caching, do not provide a cacheConfiguration object.
*
*/
private @Nullable AfdRouteCacheConfigurationResponse cacheConfiguration;
/**
* @return Domains referenced by this endpoint.
*
*/
private @Nullable List customDomains;
private String deploymentStatus;
/**
* @return Whether to enable use of this rule. Permitted values are 'Enabled' or 'Disabled'
*
*/
private @Nullable String enabledState;
/**
* @return The name of the endpoint which holds the route.
*
*/
private String endpointName;
/**
* @return Protocol this rule will use when forwarding traffic to backends.
*
*/
private @Nullable String forwardingProtocol;
/**
* @return Whether to automatically redirect HTTP traffic to HTTPS traffic. Note that this is a easy way to set up this rule and it will be the first rule that gets executed.
*
*/
private @Nullable String httpsRedirect;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return whether this route will be linked to the default endpoint domain.
*
*/
private @Nullable String linkToDefaultDomain;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return A reference to the origin group.
*
*/
private ResourceReferenceResponse originGroup;
/**
* @return A directory path on the origin that AzureFrontDoor can use to retrieve content from, e.g. contoso.cloudapp.net/originpath.
*
*/
private @Nullable String originPath;
/**
* @return The route patterns of the rule.
*
*/
private @Nullable List patternsToMatch;
/**
* @return Provisioning status
*
*/
private String provisioningState;
/**
* @return rule sets referenced by this endpoint.
*
*/
private @Nullable List ruleSets;
/**
* @return List of supported protocols for this route.
*
*/
private @Nullable List supportedProtocols;
/**
* @return Read only system data
*
*/
private SystemDataResponse systemData;
/**
* @return Resource type.
*
*/
private String type;
private GetRouteResult() {}
/**
* @return The caching configuration for this route. To disable caching, do not provide a cacheConfiguration object.
*
*/
public Optional cacheConfiguration() {
return Optional.ofNullable(this.cacheConfiguration);
}
/**
* @return Domains referenced by this endpoint.
*
*/
public List customDomains() {
return this.customDomains == null ? List.of() : this.customDomains;
}
public String deploymentStatus() {
return this.deploymentStatus;
}
/**
* @return Whether to enable use of this rule. Permitted values are 'Enabled' or 'Disabled'
*
*/
public Optional enabledState() {
return Optional.ofNullable(this.enabledState);
}
/**
* @return The name of the endpoint which holds the route.
*
*/
public String endpointName() {
return this.endpointName;
}
/**
* @return Protocol this rule will use when forwarding traffic to backends.
*
*/
public Optional forwardingProtocol() {
return Optional.ofNullable(this.forwardingProtocol);
}
/**
* @return Whether to automatically redirect HTTP traffic to HTTPS traffic. Note that this is a easy way to set up this rule and it will be the first rule that gets executed.
*
*/
public Optional httpsRedirect() {
return Optional.ofNullable(this.httpsRedirect);
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return whether this route will be linked to the default endpoint domain.
*
*/
public Optional linkToDefaultDomain() {
return Optional.ofNullable(this.linkToDefaultDomain);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return A reference to the origin group.
*
*/
public ResourceReferenceResponse originGroup() {
return this.originGroup;
}
/**
* @return A directory path on the origin that AzureFrontDoor can use to retrieve content from, e.g. contoso.cloudapp.net/originpath.
*
*/
public Optional originPath() {
return Optional.ofNullable(this.originPath);
}
/**
* @return The route patterns of the rule.
*
*/
public List patternsToMatch() {
return this.patternsToMatch == null ? List.of() : this.patternsToMatch;
}
/**
* @return Provisioning status
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return rule sets referenced by this endpoint.
*
*/
public List ruleSets() {
return this.ruleSets == null ? List.of() : this.ruleSets;
}
/**
* @return List of supported protocols for this route.
*
*/
public List supportedProtocols() {
return this.supportedProtocols == null ? List.of() : this.supportedProtocols;
}
/**
* @return Read only system data
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRouteResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AfdRouteCacheConfigurationResponse cacheConfiguration;
private @Nullable List customDomains;
private String deploymentStatus;
private @Nullable String enabledState;
private String endpointName;
private @Nullable String forwardingProtocol;
private @Nullable String httpsRedirect;
private String id;
private @Nullable String linkToDefaultDomain;
private String name;
private ResourceReferenceResponse originGroup;
private @Nullable String originPath;
private @Nullable List patternsToMatch;
private String provisioningState;
private @Nullable List ruleSets;
private @Nullable List supportedProtocols;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetRouteResult defaults) {
Objects.requireNonNull(defaults);
this.cacheConfiguration = defaults.cacheConfiguration;
this.customDomains = defaults.customDomains;
this.deploymentStatus = defaults.deploymentStatus;
this.enabledState = defaults.enabledState;
this.endpointName = defaults.endpointName;
this.forwardingProtocol = defaults.forwardingProtocol;
this.httpsRedirect = defaults.httpsRedirect;
this.id = defaults.id;
this.linkToDefaultDomain = defaults.linkToDefaultDomain;
this.name = defaults.name;
this.originGroup = defaults.originGroup;
this.originPath = defaults.originPath;
this.patternsToMatch = defaults.patternsToMatch;
this.provisioningState = defaults.provisioningState;
this.ruleSets = defaults.ruleSets;
this.supportedProtocols = defaults.supportedProtocols;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder cacheConfiguration(@Nullable AfdRouteCacheConfigurationResponse cacheConfiguration) {
this.cacheConfiguration = cacheConfiguration;
return this;
}
@CustomType.Setter
public Builder customDomains(@Nullable List customDomains) {
this.customDomains = customDomains;
return this;
}
public Builder customDomains(ActivatedResourceReferenceResponse... customDomains) {
return customDomains(List.of(customDomains));
}
@CustomType.Setter
public Builder deploymentStatus(String deploymentStatus) {
if (deploymentStatus == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "deploymentStatus");
}
this.deploymentStatus = deploymentStatus;
return this;
}
@CustomType.Setter
public Builder enabledState(@Nullable String enabledState) {
this.enabledState = enabledState;
return this;
}
@CustomType.Setter
public Builder endpointName(String endpointName) {
if (endpointName == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "endpointName");
}
this.endpointName = endpointName;
return this;
}
@CustomType.Setter
public Builder forwardingProtocol(@Nullable String forwardingProtocol) {
this.forwardingProtocol = forwardingProtocol;
return this;
}
@CustomType.Setter
public Builder httpsRedirect(@Nullable String httpsRedirect) {
this.httpsRedirect = httpsRedirect;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder linkToDefaultDomain(@Nullable String linkToDefaultDomain) {
this.linkToDefaultDomain = linkToDefaultDomain;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder originGroup(ResourceReferenceResponse originGroup) {
if (originGroup == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "originGroup");
}
this.originGroup = originGroup;
return this;
}
@CustomType.Setter
public Builder originPath(@Nullable String originPath) {
this.originPath = originPath;
return this;
}
@CustomType.Setter
public Builder patternsToMatch(@Nullable List patternsToMatch) {
this.patternsToMatch = patternsToMatch;
return this;
}
public Builder patternsToMatch(String... patternsToMatch) {
return patternsToMatch(List.of(patternsToMatch));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder ruleSets(@Nullable List ruleSets) {
this.ruleSets = ruleSets;
return this;
}
public Builder ruleSets(ResourceReferenceResponse... ruleSets) {
return ruleSets(List.of(ruleSets));
}
@CustomType.Setter
public Builder supportedProtocols(@Nullable List supportedProtocols) {
this.supportedProtocols = supportedProtocols;
return this;
}
public Builder supportedProtocols(String... supportedProtocols) {
return supportedProtocols(List.of(supportedProtocols));
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetRouteResult", "type");
}
this.type = type;
return this;
}
public GetRouteResult build() {
final var _resultValue = new GetRouteResult();
_resultValue.cacheConfiguration = cacheConfiguration;
_resultValue.customDomains = customDomains;
_resultValue.deploymentStatus = deploymentStatus;
_resultValue.enabledState = enabledState;
_resultValue.endpointName = endpointName;
_resultValue.forwardingProtocol = forwardingProtocol;
_resultValue.httpsRedirect = httpsRedirect;
_resultValue.id = id;
_resultValue.linkToDefaultDomain = linkToDefaultDomain;
_resultValue.name = name;
_resultValue.originGroup = originGroup;
_resultValue.originPath = originPath;
_resultValue.patternsToMatch = patternsToMatch;
_resultValue.provisioningState = provisioningState;
_resultValue.ruleSets = ruleSets;
_resultValue.supportedProtocols = supportedProtocols;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy