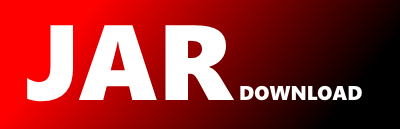
com.pulumi.azurenative.cloudngfw.PostRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cloudngfw;
import com.pulumi.azurenative.cloudngfw.enums.ActionEnum;
import com.pulumi.azurenative.cloudngfw.enums.BooleanEnum;
import com.pulumi.azurenative.cloudngfw.enums.DecryptionRuleTypeEnum;
import com.pulumi.azurenative.cloudngfw.enums.StateEnum;
import com.pulumi.azurenative.cloudngfw.inputs.CategoryArgs;
import com.pulumi.azurenative.cloudngfw.inputs.DestinationAddrArgs;
import com.pulumi.azurenative.cloudngfw.inputs.SourceAddrArgs;
import com.pulumi.azurenative.cloudngfw.inputs.TagInfoArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PostRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final PostRuleArgs Empty = new PostRuleArgs();
/**
* rule action
*
*/
@Import(name="actionType")
private @Nullable Output> actionType;
/**
* @return rule action
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy