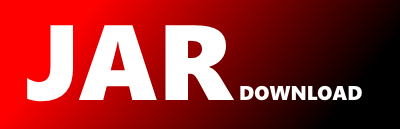
com.pulumi.azurenative.cloudngfw.inputs.SecurityServicesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cloudngfw.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* security services
*
*/
public final class SecurityServicesArgs extends com.pulumi.resources.ResourceArgs {
public static final SecurityServicesArgs Empty = new SecurityServicesArgs();
/**
* Anti spyware Profile data
*
*/
@Import(name="antiSpywareProfile")
private @Nullable Output antiSpywareProfile;
/**
* @return Anti spyware Profile data
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy