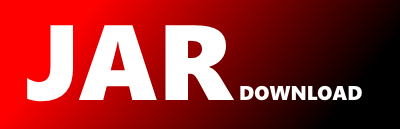
com.pulumi.azurenative.community.CommunityTrainingArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.community;
import com.pulumi.azurenative.community.inputs.IdentityConfigurationPropertiesArgs;
import com.pulumi.azurenative.community.inputs.SkuArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CommunityTrainingArgs extends com.pulumi.resources.ResourceArgs {
public static final CommunityTrainingArgs Empty = new CommunityTrainingArgs();
/**
* The name of the Community Training Resource
*
*/
@Import(name="communityTrainingName")
private @Nullable Output communityTrainingName;
/**
* @return The name of the Community Training Resource
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy