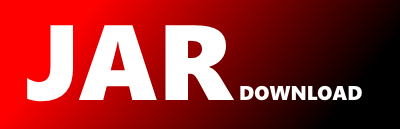
com.pulumi.azurenative.compute.CapacityReservationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.inputs.SkuArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CapacityReservationArgs extends com.pulumi.resources.ResourceArgs {
public static final CapacityReservationArgs Empty = new CapacityReservationArgs();
/**
* The name of the capacity reservation group.
*
*/
@Import(name="capacityReservationGroupName", required=true)
private Output capacityReservationGroupName;
/**
* @return The name of the capacity reservation group.
*
*/
public Output capacityReservationGroupName() {
return this.capacityReservationGroupName;
}
/**
* The name of the capacity reservation.
*
*/
@Import(name="capacityReservationName")
private @Nullable Output capacityReservationName;
/**
* @return The name of the capacity reservation.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy