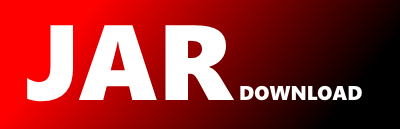
com.pulumi.azurenative.compute.VirtualMachineExtensionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.inputs.KeyVaultSecretReferenceArgs;
import com.pulumi.azurenative.compute.inputs.VirtualMachineExtensionInstanceViewArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualMachineExtensionArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualMachineExtensionArgs Empty = new VirtualMachineExtensionArgs();
/**
* Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*
*/
@Import(name="autoUpgradeMinorVersion")
private @Nullable Output autoUpgradeMinorVersion;
/**
* @return Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy