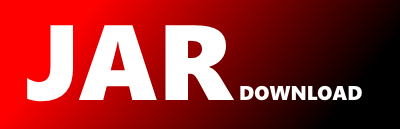
com.pulumi.azurenative.compute.outputs.GetDedicatedHostResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.DedicatedHostInstanceViewResponse;
import com.pulumi.azurenative.compute.outputs.SkuResponse;
import com.pulumi.azurenative.compute.outputs.SubResourceReadOnlyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDedicatedHostResult {
/**
* @return Specifies whether the dedicated host should be replaced automatically in case of a failure. The value is defaulted to 'true' when not provided.
*
*/
private @Nullable Boolean autoReplaceOnFailure;
/**
* @return A unique id generated and assigned to the dedicated host by the platform. Does not change throughout the lifetime of the host.
*
*/
private String hostId;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return The dedicated host instance view.
*
*/
private DedicatedHostInstanceViewResponse instanceView;
/**
* @return Specifies the software license type that will be applied to the VMs deployed on the dedicated host. Possible values are: **None,** **Windows_Server_Hybrid,** **Windows_Server_Perpetual.** The default value is: **None.**
*
*/
private @Nullable String licenseType;
/**
* @return Resource location
*
*/
private String location;
/**
* @return Resource name
*
*/
private String name;
/**
* @return Fault domain of the dedicated host within a dedicated host group.
*
*/
private @Nullable Integer platformFaultDomain;
/**
* @return The provisioning state, which only appears in the response.
*
*/
private String provisioningState;
/**
* @return The date when the host was first provisioned.
*
*/
private String provisioningTime;
/**
* @return SKU of the dedicated host for Hardware Generation and VM family. Only name is required to be set. List Microsoft.Compute SKUs for a list of possible values.
*
*/
private SkuResponse sku;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Specifies the time at which the Dedicated Host resource was created. Minimum api-version: 2021-11-01.
*
*/
private String timeCreated;
/**
* @return Resource type
*
*/
private String type;
/**
* @return A list of references to all virtual machines in the Dedicated Host.
*
*/
private List virtualMachines;
private GetDedicatedHostResult() {}
/**
* @return Specifies whether the dedicated host should be replaced automatically in case of a failure. The value is defaulted to 'true' when not provided.
*
*/
public Optional autoReplaceOnFailure() {
return Optional.ofNullable(this.autoReplaceOnFailure);
}
/**
* @return A unique id generated and assigned to the dedicated host by the platform. Does not change throughout the lifetime of the host.
*
*/
public String hostId() {
return this.hostId;
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return The dedicated host instance view.
*
*/
public DedicatedHostInstanceViewResponse instanceView() {
return this.instanceView;
}
/**
* @return Specifies the software license type that will be applied to the VMs deployed on the dedicated host. Possible values are: **None,** **Windows_Server_Hybrid,** **Windows_Server_Perpetual.** The default value is: **None.**
*
*/
public Optional licenseType() {
return Optional.ofNullable(this.licenseType);
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return Fault domain of the dedicated host within a dedicated host group.
*
*/
public Optional platformFaultDomain() {
return Optional.ofNullable(this.platformFaultDomain);
}
/**
* @return The provisioning state, which only appears in the response.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The date when the host was first provisioned.
*
*/
public String provisioningTime() {
return this.provisioningTime;
}
/**
* @return SKU of the dedicated host for Hardware Generation and VM family. Only name is required to be set. List Microsoft.Compute SKUs for a list of possible values.
*
*/
public SkuResponse sku() {
return this.sku;
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Specifies the time at which the Dedicated Host resource was created. Minimum api-version: 2021-11-01.
*
*/
public String timeCreated() {
return this.timeCreated;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
/**
* @return A list of references to all virtual machines in the Dedicated Host.
*
*/
public List virtualMachines() {
return this.virtualMachines;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDedicatedHostResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean autoReplaceOnFailure;
private String hostId;
private String id;
private DedicatedHostInstanceViewResponse instanceView;
private @Nullable String licenseType;
private String location;
private String name;
private @Nullable Integer platformFaultDomain;
private String provisioningState;
private String provisioningTime;
private SkuResponse sku;
private @Nullable Map tags;
private String timeCreated;
private String type;
private List virtualMachines;
public Builder() {}
public Builder(GetDedicatedHostResult defaults) {
Objects.requireNonNull(defaults);
this.autoReplaceOnFailure = defaults.autoReplaceOnFailure;
this.hostId = defaults.hostId;
this.id = defaults.id;
this.instanceView = defaults.instanceView;
this.licenseType = defaults.licenseType;
this.location = defaults.location;
this.name = defaults.name;
this.platformFaultDomain = defaults.platformFaultDomain;
this.provisioningState = defaults.provisioningState;
this.provisioningTime = defaults.provisioningTime;
this.sku = defaults.sku;
this.tags = defaults.tags;
this.timeCreated = defaults.timeCreated;
this.type = defaults.type;
this.virtualMachines = defaults.virtualMachines;
}
@CustomType.Setter
public Builder autoReplaceOnFailure(@Nullable Boolean autoReplaceOnFailure) {
this.autoReplaceOnFailure = autoReplaceOnFailure;
return this;
}
@CustomType.Setter
public Builder hostId(String hostId) {
if (hostId == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "hostId");
}
this.hostId = hostId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder instanceView(DedicatedHostInstanceViewResponse instanceView) {
if (instanceView == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "instanceView");
}
this.instanceView = instanceView;
return this;
}
@CustomType.Setter
public Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder platformFaultDomain(@Nullable Integer platformFaultDomain) {
this.platformFaultDomain = platformFaultDomain;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder provisioningTime(String provisioningTime) {
if (provisioningTime == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "provisioningTime");
}
this.provisioningTime = provisioningTime;
return this;
}
@CustomType.Setter
public Builder sku(SkuResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder timeCreated(String timeCreated) {
if (timeCreated == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "timeCreated");
}
this.timeCreated = timeCreated;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualMachines(List virtualMachines) {
if (virtualMachines == null) {
throw new MissingRequiredPropertyException("GetDedicatedHostResult", "virtualMachines");
}
this.virtualMachines = virtualMachines;
return this;
}
public Builder virtualMachines(SubResourceReadOnlyResponse... virtualMachines) {
return virtualMachines(List.of(virtualMachines));
}
public GetDedicatedHostResult build() {
final var _resultValue = new GetDedicatedHostResult();
_resultValue.autoReplaceOnFailure = autoReplaceOnFailure;
_resultValue.hostId = hostId;
_resultValue.id = id;
_resultValue.instanceView = instanceView;
_resultValue.licenseType = licenseType;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.platformFaultDomain = platformFaultDomain;
_resultValue.provisioningState = provisioningState;
_resultValue.provisioningTime = provisioningTime;
_resultValue.sku = sku;
_resultValue.tags = tags;
_resultValue.timeCreated = timeCreated;
_resultValue.type = type;
_resultValue.virtualMachines = virtualMachines;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy