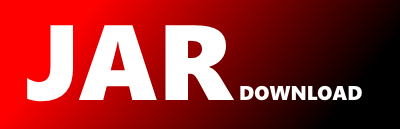
com.pulumi.azurenative.compute.outputs.GetDiskResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.CreationDataResponse;
import com.pulumi.azurenative.compute.outputs.DiskSecurityProfileResponse;
import com.pulumi.azurenative.compute.outputs.DiskSkuResponse;
import com.pulumi.azurenative.compute.outputs.EncryptionResponse;
import com.pulumi.azurenative.compute.outputs.EncryptionSettingsCollectionResponse;
import com.pulumi.azurenative.compute.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.compute.outputs.PropertyUpdatesInProgressResponse;
import com.pulumi.azurenative.compute.outputs.PurchasePlanResponse;
import com.pulumi.azurenative.compute.outputs.ShareInfoElementResponse;
import com.pulumi.azurenative.compute.outputs.SupportedCapabilitiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDiskResult {
/**
* @return Set to true to enable bursting beyond the provisioned performance target of the disk. Bursting is disabled by default. Does not apply to Ultra disks.
*
*/
private @Nullable Boolean burstingEnabled;
/**
* @return Latest time when bursting was last enabled on a disk.
*
*/
private String burstingEnabledTime;
/**
* @return Percentage complete for the background copy when a resource is created via the CopyStart operation.
*
*/
private @Nullable Double completionPercent;
/**
* @return Disk source information. CreationData information cannot be changed after the disk has been created.
*
*/
private CreationDataResponse creationData;
/**
* @return Additional authentication requirements when exporting or uploading to a disk or snapshot.
*
*/
private @Nullable String dataAccessAuthMode;
/**
* @return ARM id of the DiskAccess resource for using private endpoints on disks.
*
*/
private @Nullable String diskAccessId;
/**
* @return The total number of IOPS that will be allowed across all VMs mounting the shared disk as ReadOnly. One operation can transfer between 4k and 256k bytes.
*
*/
private @Nullable Double diskIOPSReadOnly;
/**
* @return The number of IOPS allowed for this disk; only settable for UltraSSD disks. One operation can transfer between 4k and 256k bytes.
*
*/
private @Nullable Double diskIOPSReadWrite;
/**
* @return The total throughput (MBps) that will be allowed across all VMs mounting the shared disk as ReadOnly. MBps means millions of bytes per second - MB here uses the ISO notation, of powers of 10.
*
*/
private @Nullable Double diskMBpsReadOnly;
/**
* @return The bandwidth allowed for this disk; only settable for UltraSSD disks. MBps means millions of bytes per second - MB here uses the ISO notation, of powers of 10.
*
*/
private @Nullable Double diskMBpsReadWrite;
/**
* @return The size of the disk in bytes. This field is read only.
*
*/
private Double diskSizeBytes;
/**
* @return If creationData.createOption is Empty, this field is mandatory and it indicates the size of the disk to create. If this field is present for updates or creation with other options, it indicates a resize. Resizes are only allowed if the disk is not attached to a running VM, and can only increase the disk's size.
*
*/
private @Nullable Integer diskSizeGB;
/**
* @return The state of the disk.
*
*/
private String diskState;
/**
* @return Encryption property can be used to encrypt data at rest with customer managed keys or platform managed keys.
*
*/
private @Nullable EncryptionResponse encryption;
/**
* @return Encryption settings collection used for Azure Disk Encryption, can contain multiple encryption settings per disk or snapshot.
*
*/
private @Nullable EncryptionSettingsCollectionResponse encryptionSettingsCollection;
/**
* @return The extended location where the disk will be created. Extended location cannot be changed.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return The hypervisor generation of the Virtual Machine. Applicable to OS disks only.
*
*/
private @Nullable String hyperVGeneration;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return Resource location
*
*/
private String location;
/**
* @return A relative URI containing the ID of the VM that has the disk attached.
*
*/
private String managedBy;
/**
* @return List of relative URIs containing the IDs of the VMs that have the disk attached. maxShares should be set to a value greater than one for disks to allow attaching them to multiple VMs.
*
*/
private List managedByExtended;
/**
* @return The maximum number of VMs that can attach to the disk at the same time. Value greater than one indicates a disk that can be mounted on multiple VMs at the same time.
*
*/
private @Nullable Integer maxShares;
/**
* @return Resource name
*
*/
private String name;
/**
* @return Policy for accessing the disk via network.
*
*/
private @Nullable String networkAccessPolicy;
/**
* @return Setting this property to true improves reliability and performance of data disks that are frequently (more than 5 times a day) by detached from one virtual machine and attached to another. This property should not be set for disks that are not detached and attached frequently as it causes the disks to not align with the fault domain of the virtual machine.
*
*/
private @Nullable Boolean optimizedForFrequentAttach;
/**
* @return The Operating System type.
*
*/
private @Nullable String osType;
/**
* @return Properties of the disk for which update is pending.
*
*/
private PropertyUpdatesInProgressResponse propertyUpdatesInProgress;
/**
* @return The disk provisioning state.
*
*/
private String provisioningState;
/**
* @return Policy for controlling export on the disk.
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Purchase plan information for the the image from which the OS disk was created. E.g. - {name: 2019-Datacenter, publisher: MicrosoftWindowsServer, product: WindowsServer}
*
*/
private @Nullable PurchasePlanResponse purchasePlan;
/**
* @return Contains the security related information for the resource.
*
*/
private @Nullable DiskSecurityProfileResponse securityProfile;
/**
* @return Details of the list of all VMs that have the disk attached. maxShares should be set to a value greater than one for disks to allow attaching them to multiple VMs.
*
*/
private List shareInfo;
/**
* @return The disks sku name. Can be Standard_LRS, Premium_LRS, StandardSSD_LRS, UltraSSD_LRS, Premium_ZRS, StandardSSD_ZRS, or PremiumV2_LRS.
*
*/
private @Nullable DiskSkuResponse sku;
/**
* @return List of supported capabilities for the image from which the OS disk was created.
*
*/
private @Nullable SupportedCapabilitiesResponse supportedCapabilities;
/**
* @return Indicates the OS on a disk supports hibernation.
*
*/
private @Nullable Boolean supportsHibernation;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Performance tier of the disk (e.g, P4, S10) as described here: https://azure.microsoft.com/en-us/pricing/details/managed-disks/. Does not apply to Ultra disks.
*
*/
private @Nullable String tier;
/**
* @return The time when the disk was created.
*
*/
private String timeCreated;
/**
* @return Resource type
*
*/
private String type;
/**
* @return Unique Guid identifying the resource.
*
*/
private String uniqueId;
/**
* @return The Logical zone list for Disk.
*
*/
private @Nullable List zones;
private GetDiskResult() {}
/**
* @return Set to true to enable bursting beyond the provisioned performance target of the disk. Bursting is disabled by default. Does not apply to Ultra disks.
*
*/
public Optional burstingEnabled() {
return Optional.ofNullable(this.burstingEnabled);
}
/**
* @return Latest time when bursting was last enabled on a disk.
*
*/
public String burstingEnabledTime() {
return this.burstingEnabledTime;
}
/**
* @return Percentage complete for the background copy when a resource is created via the CopyStart operation.
*
*/
public Optional completionPercent() {
return Optional.ofNullable(this.completionPercent);
}
/**
* @return Disk source information. CreationData information cannot be changed after the disk has been created.
*
*/
public CreationDataResponse creationData() {
return this.creationData;
}
/**
* @return Additional authentication requirements when exporting or uploading to a disk or snapshot.
*
*/
public Optional dataAccessAuthMode() {
return Optional.ofNullable(this.dataAccessAuthMode);
}
/**
* @return ARM id of the DiskAccess resource for using private endpoints on disks.
*
*/
public Optional diskAccessId() {
return Optional.ofNullable(this.diskAccessId);
}
/**
* @return The total number of IOPS that will be allowed across all VMs mounting the shared disk as ReadOnly. One operation can transfer between 4k and 256k bytes.
*
*/
public Optional diskIOPSReadOnly() {
return Optional.ofNullable(this.diskIOPSReadOnly);
}
/**
* @return The number of IOPS allowed for this disk; only settable for UltraSSD disks. One operation can transfer between 4k and 256k bytes.
*
*/
public Optional diskIOPSReadWrite() {
return Optional.ofNullable(this.diskIOPSReadWrite);
}
/**
* @return The total throughput (MBps) that will be allowed across all VMs mounting the shared disk as ReadOnly. MBps means millions of bytes per second - MB here uses the ISO notation, of powers of 10.
*
*/
public Optional diskMBpsReadOnly() {
return Optional.ofNullable(this.diskMBpsReadOnly);
}
/**
* @return The bandwidth allowed for this disk; only settable for UltraSSD disks. MBps means millions of bytes per second - MB here uses the ISO notation, of powers of 10.
*
*/
public Optional diskMBpsReadWrite() {
return Optional.ofNullable(this.diskMBpsReadWrite);
}
/**
* @return The size of the disk in bytes. This field is read only.
*
*/
public Double diskSizeBytes() {
return this.diskSizeBytes;
}
/**
* @return If creationData.createOption is Empty, this field is mandatory and it indicates the size of the disk to create. If this field is present for updates or creation with other options, it indicates a resize. Resizes are only allowed if the disk is not attached to a running VM, and can only increase the disk's size.
*
*/
public Optional diskSizeGB() {
return Optional.ofNullable(this.diskSizeGB);
}
/**
* @return The state of the disk.
*
*/
public String diskState() {
return this.diskState;
}
/**
* @return Encryption property can be used to encrypt data at rest with customer managed keys or platform managed keys.
*
*/
public Optional encryption() {
return Optional.ofNullable(this.encryption);
}
/**
* @return Encryption settings collection used for Azure Disk Encryption, can contain multiple encryption settings per disk or snapshot.
*
*/
public Optional encryptionSettingsCollection() {
return Optional.ofNullable(this.encryptionSettingsCollection);
}
/**
* @return The extended location where the disk will be created. Extended location cannot be changed.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return The hypervisor generation of the Virtual Machine. Applicable to OS disks only.
*
*/
public Optional hyperVGeneration() {
return Optional.ofNullable(this.hyperVGeneration);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return A relative URI containing the ID of the VM that has the disk attached.
*
*/
public String managedBy() {
return this.managedBy;
}
/**
* @return List of relative URIs containing the IDs of the VMs that have the disk attached. maxShares should be set to a value greater than one for disks to allow attaching them to multiple VMs.
*
*/
public List managedByExtended() {
return this.managedByExtended;
}
/**
* @return The maximum number of VMs that can attach to the disk at the same time. Value greater than one indicates a disk that can be mounted on multiple VMs at the same time.
*
*/
public Optional maxShares() {
return Optional.ofNullable(this.maxShares);
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return Policy for accessing the disk via network.
*
*/
public Optional networkAccessPolicy() {
return Optional.ofNullable(this.networkAccessPolicy);
}
/**
* @return Setting this property to true improves reliability and performance of data disks that are frequently (more than 5 times a day) by detached from one virtual machine and attached to another. This property should not be set for disks that are not detached and attached frequently as it causes the disks to not align with the fault domain of the virtual machine.
*
*/
public Optional optimizedForFrequentAttach() {
return Optional.ofNullable(this.optimizedForFrequentAttach);
}
/**
* @return The Operating System type.
*
*/
public Optional osType() {
return Optional.ofNullable(this.osType);
}
/**
* @return Properties of the disk for which update is pending.
*
*/
public PropertyUpdatesInProgressResponse propertyUpdatesInProgress() {
return this.propertyUpdatesInProgress;
}
/**
* @return The disk provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Policy for controlling export on the disk.
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Purchase plan information for the the image from which the OS disk was created. E.g. - {name: 2019-Datacenter, publisher: MicrosoftWindowsServer, product: WindowsServer}
*
*/
public Optional purchasePlan() {
return Optional.ofNullable(this.purchasePlan);
}
/**
* @return Contains the security related information for the resource.
*
*/
public Optional securityProfile() {
return Optional.ofNullable(this.securityProfile);
}
/**
* @return Details of the list of all VMs that have the disk attached. maxShares should be set to a value greater than one for disks to allow attaching them to multiple VMs.
*
*/
public List shareInfo() {
return this.shareInfo;
}
/**
* @return The disks sku name. Can be Standard_LRS, Premium_LRS, StandardSSD_LRS, UltraSSD_LRS, Premium_ZRS, StandardSSD_ZRS, or PremiumV2_LRS.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return List of supported capabilities for the image from which the OS disk was created.
*
*/
public Optional supportedCapabilities() {
return Optional.ofNullable(this.supportedCapabilities);
}
/**
* @return Indicates the OS on a disk supports hibernation.
*
*/
public Optional supportsHibernation() {
return Optional.ofNullable(this.supportsHibernation);
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Performance tier of the disk (e.g, P4, S10) as described here: https://azure.microsoft.com/en-us/pricing/details/managed-disks/. Does not apply to Ultra disks.
*
*/
public Optional tier() {
return Optional.ofNullable(this.tier);
}
/**
* @return The time when the disk was created.
*
*/
public String timeCreated() {
return this.timeCreated;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
/**
* @return Unique Guid identifying the resource.
*
*/
public String uniqueId() {
return this.uniqueId;
}
/**
* @return The Logical zone list for Disk.
*
*/
public List zones() {
return this.zones == null ? List.of() : this.zones;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDiskResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean burstingEnabled;
private String burstingEnabledTime;
private @Nullable Double completionPercent;
private CreationDataResponse creationData;
private @Nullable String dataAccessAuthMode;
private @Nullable String diskAccessId;
private @Nullable Double diskIOPSReadOnly;
private @Nullable Double diskIOPSReadWrite;
private @Nullable Double diskMBpsReadOnly;
private @Nullable Double diskMBpsReadWrite;
private Double diskSizeBytes;
private @Nullable Integer diskSizeGB;
private String diskState;
private @Nullable EncryptionResponse encryption;
private @Nullable EncryptionSettingsCollectionResponse encryptionSettingsCollection;
private @Nullable ExtendedLocationResponse extendedLocation;
private @Nullable String hyperVGeneration;
private String id;
private String location;
private String managedBy;
private List managedByExtended;
private @Nullable Integer maxShares;
private String name;
private @Nullable String networkAccessPolicy;
private @Nullable Boolean optimizedForFrequentAttach;
private @Nullable String osType;
private PropertyUpdatesInProgressResponse propertyUpdatesInProgress;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private @Nullable PurchasePlanResponse purchasePlan;
private @Nullable DiskSecurityProfileResponse securityProfile;
private List shareInfo;
private @Nullable DiskSkuResponse sku;
private @Nullable SupportedCapabilitiesResponse supportedCapabilities;
private @Nullable Boolean supportsHibernation;
private @Nullable Map tags;
private @Nullable String tier;
private String timeCreated;
private String type;
private String uniqueId;
private @Nullable List zones;
public Builder() {}
public Builder(GetDiskResult defaults) {
Objects.requireNonNull(defaults);
this.burstingEnabled = defaults.burstingEnabled;
this.burstingEnabledTime = defaults.burstingEnabledTime;
this.completionPercent = defaults.completionPercent;
this.creationData = defaults.creationData;
this.dataAccessAuthMode = defaults.dataAccessAuthMode;
this.diskAccessId = defaults.diskAccessId;
this.diskIOPSReadOnly = defaults.diskIOPSReadOnly;
this.diskIOPSReadWrite = defaults.diskIOPSReadWrite;
this.diskMBpsReadOnly = defaults.diskMBpsReadOnly;
this.diskMBpsReadWrite = defaults.diskMBpsReadWrite;
this.diskSizeBytes = defaults.diskSizeBytes;
this.diskSizeGB = defaults.diskSizeGB;
this.diskState = defaults.diskState;
this.encryption = defaults.encryption;
this.encryptionSettingsCollection = defaults.encryptionSettingsCollection;
this.extendedLocation = defaults.extendedLocation;
this.hyperVGeneration = defaults.hyperVGeneration;
this.id = defaults.id;
this.location = defaults.location;
this.managedBy = defaults.managedBy;
this.managedByExtended = defaults.managedByExtended;
this.maxShares = defaults.maxShares;
this.name = defaults.name;
this.networkAccessPolicy = defaults.networkAccessPolicy;
this.optimizedForFrequentAttach = defaults.optimizedForFrequentAttach;
this.osType = defaults.osType;
this.propertyUpdatesInProgress = defaults.propertyUpdatesInProgress;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.purchasePlan = defaults.purchasePlan;
this.securityProfile = defaults.securityProfile;
this.shareInfo = defaults.shareInfo;
this.sku = defaults.sku;
this.supportedCapabilities = defaults.supportedCapabilities;
this.supportsHibernation = defaults.supportsHibernation;
this.tags = defaults.tags;
this.tier = defaults.tier;
this.timeCreated = defaults.timeCreated;
this.type = defaults.type;
this.uniqueId = defaults.uniqueId;
this.zones = defaults.zones;
}
@CustomType.Setter
public Builder burstingEnabled(@Nullable Boolean burstingEnabled) {
this.burstingEnabled = burstingEnabled;
return this;
}
@CustomType.Setter
public Builder burstingEnabledTime(String burstingEnabledTime) {
if (burstingEnabledTime == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "burstingEnabledTime");
}
this.burstingEnabledTime = burstingEnabledTime;
return this;
}
@CustomType.Setter
public Builder completionPercent(@Nullable Double completionPercent) {
this.completionPercent = completionPercent;
return this;
}
@CustomType.Setter
public Builder creationData(CreationDataResponse creationData) {
if (creationData == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "creationData");
}
this.creationData = creationData;
return this;
}
@CustomType.Setter
public Builder dataAccessAuthMode(@Nullable String dataAccessAuthMode) {
this.dataAccessAuthMode = dataAccessAuthMode;
return this;
}
@CustomType.Setter
public Builder diskAccessId(@Nullable String diskAccessId) {
this.diskAccessId = diskAccessId;
return this;
}
@CustomType.Setter
public Builder diskIOPSReadOnly(@Nullable Double diskIOPSReadOnly) {
this.diskIOPSReadOnly = diskIOPSReadOnly;
return this;
}
@CustomType.Setter
public Builder diskIOPSReadWrite(@Nullable Double diskIOPSReadWrite) {
this.diskIOPSReadWrite = diskIOPSReadWrite;
return this;
}
@CustomType.Setter
public Builder diskMBpsReadOnly(@Nullable Double diskMBpsReadOnly) {
this.diskMBpsReadOnly = diskMBpsReadOnly;
return this;
}
@CustomType.Setter
public Builder diskMBpsReadWrite(@Nullable Double diskMBpsReadWrite) {
this.diskMBpsReadWrite = diskMBpsReadWrite;
return this;
}
@CustomType.Setter
public Builder diskSizeBytes(Double diskSizeBytes) {
if (diskSizeBytes == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "diskSizeBytes");
}
this.diskSizeBytes = diskSizeBytes;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(@Nullable Integer diskSizeGB) {
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder diskState(String diskState) {
if (diskState == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "diskState");
}
this.diskState = diskState;
return this;
}
@CustomType.Setter
public Builder encryption(@Nullable EncryptionResponse encryption) {
this.encryption = encryption;
return this;
}
@CustomType.Setter
public Builder encryptionSettingsCollection(@Nullable EncryptionSettingsCollectionResponse encryptionSettingsCollection) {
this.encryptionSettingsCollection = encryptionSettingsCollection;
return this;
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder hyperVGeneration(@Nullable String hyperVGeneration) {
this.hyperVGeneration = hyperVGeneration;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedBy(String managedBy) {
if (managedBy == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "managedBy");
}
this.managedBy = managedBy;
return this;
}
@CustomType.Setter
public Builder managedByExtended(List managedByExtended) {
if (managedByExtended == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "managedByExtended");
}
this.managedByExtended = managedByExtended;
return this;
}
public Builder managedByExtended(String... managedByExtended) {
return managedByExtended(List.of(managedByExtended));
}
@CustomType.Setter
public Builder maxShares(@Nullable Integer maxShares) {
this.maxShares = maxShares;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkAccessPolicy(@Nullable String networkAccessPolicy) {
this.networkAccessPolicy = networkAccessPolicy;
return this;
}
@CustomType.Setter
public Builder optimizedForFrequentAttach(@Nullable Boolean optimizedForFrequentAttach) {
this.optimizedForFrequentAttach = optimizedForFrequentAttach;
return this;
}
@CustomType.Setter
public Builder osType(@Nullable String osType) {
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder propertyUpdatesInProgress(PropertyUpdatesInProgressResponse propertyUpdatesInProgress) {
if (propertyUpdatesInProgress == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "propertyUpdatesInProgress");
}
this.propertyUpdatesInProgress = propertyUpdatesInProgress;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder purchasePlan(@Nullable PurchasePlanResponse purchasePlan) {
this.purchasePlan = purchasePlan;
return this;
}
@CustomType.Setter
public Builder securityProfile(@Nullable DiskSecurityProfileResponse securityProfile) {
this.securityProfile = securityProfile;
return this;
}
@CustomType.Setter
public Builder shareInfo(List shareInfo) {
if (shareInfo == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "shareInfo");
}
this.shareInfo = shareInfo;
return this;
}
public Builder shareInfo(ShareInfoElementResponse... shareInfo) {
return shareInfo(List.of(shareInfo));
}
@CustomType.Setter
public Builder sku(@Nullable DiskSkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder supportedCapabilities(@Nullable SupportedCapabilitiesResponse supportedCapabilities) {
this.supportedCapabilities = supportedCapabilities;
return this;
}
@CustomType.Setter
public Builder supportsHibernation(@Nullable Boolean supportsHibernation) {
this.supportsHibernation = supportsHibernation;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tier(@Nullable String tier) {
this.tier = tier;
return this;
}
@CustomType.Setter
public Builder timeCreated(String timeCreated) {
if (timeCreated == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "timeCreated");
}
this.timeCreated = timeCreated;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder uniqueId(String uniqueId) {
if (uniqueId == null) {
throw new MissingRequiredPropertyException("GetDiskResult", "uniqueId");
}
this.uniqueId = uniqueId;
return this;
}
@CustomType.Setter
public Builder zones(@Nullable List zones) {
this.zones = zones;
return this;
}
public Builder zones(String... zones) {
return zones(List.of(zones));
}
public GetDiskResult build() {
final var _resultValue = new GetDiskResult();
_resultValue.burstingEnabled = burstingEnabled;
_resultValue.burstingEnabledTime = burstingEnabledTime;
_resultValue.completionPercent = completionPercent;
_resultValue.creationData = creationData;
_resultValue.dataAccessAuthMode = dataAccessAuthMode;
_resultValue.diskAccessId = diskAccessId;
_resultValue.diskIOPSReadOnly = diskIOPSReadOnly;
_resultValue.diskIOPSReadWrite = diskIOPSReadWrite;
_resultValue.diskMBpsReadOnly = diskMBpsReadOnly;
_resultValue.diskMBpsReadWrite = diskMBpsReadWrite;
_resultValue.diskSizeBytes = diskSizeBytes;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.diskState = diskState;
_resultValue.encryption = encryption;
_resultValue.encryptionSettingsCollection = encryptionSettingsCollection;
_resultValue.extendedLocation = extendedLocation;
_resultValue.hyperVGeneration = hyperVGeneration;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.managedBy = managedBy;
_resultValue.managedByExtended = managedByExtended;
_resultValue.maxShares = maxShares;
_resultValue.name = name;
_resultValue.networkAccessPolicy = networkAccessPolicy;
_resultValue.optimizedForFrequentAttach = optimizedForFrequentAttach;
_resultValue.osType = osType;
_resultValue.propertyUpdatesInProgress = propertyUpdatesInProgress;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.purchasePlan = purchasePlan;
_resultValue.securityProfile = securityProfile;
_resultValue.shareInfo = shareInfo;
_resultValue.sku = sku;
_resultValue.supportedCapabilities = supportedCapabilities;
_resultValue.supportsHibernation = supportsHibernation;
_resultValue.tags = tags;
_resultValue.tier = tier;
_resultValue.timeCreated = timeCreated;
_resultValue.type = type;
_resultValue.uniqueId = uniqueId;
_resultValue.zones = zones;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy