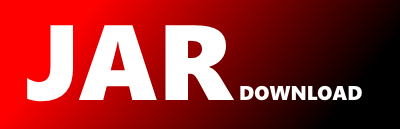
com.pulumi.azurenative.connectedvmwarevsphere.outputs.InfrastructureProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.connectedvmwarevsphere.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InfrastructureProfileResponse {
/**
* @return Gets the name of the corresponding resource in Kubernetes.
*
*/
private String customResourceName;
/**
* @return Firmware type
*
*/
private @Nullable String firmwareType;
/**
* @return Gets or sets the folder path of the vm.
*
*/
private String folderPath;
/**
* @return Gets or sets the instance uuid of the vm.
*
*/
private String instanceUuid;
/**
* @return Gets or sets the inventory Item ID for the virtual machine.
*
*/
private @Nullable String inventoryItemId;
/**
* @return Gets or sets the vCenter Managed Object name for the virtual machine.
*
*/
private String moName;
/**
* @return Gets or sets the vCenter MoRef (Managed Object Reference) ID for the virtual machine.
*
*/
private String moRefId;
/**
* @return Gets or sets the SMBIOS UUID of the vm.
*
*/
private @Nullable String smbiosUuid;
/**
* @return Gets or sets the ARM Id of the template resource to deploy the virtual machine.
*
*/
private @Nullable String templateId;
/**
* @return Gets or sets the ARM Id of the vCenter resource in which this resource pool resides.
*
*/
private @Nullable String vCenterId;
private InfrastructureProfileResponse() {}
/**
* @return Gets the name of the corresponding resource in Kubernetes.
*
*/
public String customResourceName() {
return this.customResourceName;
}
/**
* @return Firmware type
*
*/
public Optional firmwareType() {
return Optional.ofNullable(this.firmwareType);
}
/**
* @return Gets or sets the folder path of the vm.
*
*/
public String folderPath() {
return this.folderPath;
}
/**
* @return Gets or sets the instance uuid of the vm.
*
*/
public String instanceUuid() {
return this.instanceUuid;
}
/**
* @return Gets or sets the inventory Item ID for the virtual machine.
*
*/
public Optional inventoryItemId() {
return Optional.ofNullable(this.inventoryItemId);
}
/**
* @return Gets or sets the vCenter Managed Object name for the virtual machine.
*
*/
public String moName() {
return this.moName;
}
/**
* @return Gets or sets the vCenter MoRef (Managed Object Reference) ID for the virtual machine.
*
*/
public String moRefId() {
return this.moRefId;
}
/**
* @return Gets or sets the SMBIOS UUID of the vm.
*
*/
public Optional smbiosUuid() {
return Optional.ofNullable(this.smbiosUuid);
}
/**
* @return Gets or sets the ARM Id of the template resource to deploy the virtual machine.
*
*/
public Optional templateId() {
return Optional.ofNullable(this.templateId);
}
/**
* @return Gets or sets the ARM Id of the vCenter resource in which this resource pool resides.
*
*/
public Optional vCenterId() {
return Optional.ofNullable(this.vCenterId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InfrastructureProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String customResourceName;
private @Nullable String firmwareType;
private String folderPath;
private String instanceUuid;
private @Nullable String inventoryItemId;
private String moName;
private String moRefId;
private @Nullable String smbiosUuid;
private @Nullable String templateId;
private @Nullable String vCenterId;
public Builder() {}
public Builder(InfrastructureProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.customResourceName = defaults.customResourceName;
this.firmwareType = defaults.firmwareType;
this.folderPath = defaults.folderPath;
this.instanceUuid = defaults.instanceUuid;
this.inventoryItemId = defaults.inventoryItemId;
this.moName = defaults.moName;
this.moRefId = defaults.moRefId;
this.smbiosUuid = defaults.smbiosUuid;
this.templateId = defaults.templateId;
this.vCenterId = defaults.vCenterId;
}
@CustomType.Setter
public Builder customResourceName(String customResourceName) {
if (customResourceName == null) {
throw new MissingRequiredPropertyException("InfrastructureProfileResponse", "customResourceName");
}
this.customResourceName = customResourceName;
return this;
}
@CustomType.Setter
public Builder firmwareType(@Nullable String firmwareType) {
this.firmwareType = firmwareType;
return this;
}
@CustomType.Setter
public Builder folderPath(String folderPath) {
if (folderPath == null) {
throw new MissingRequiredPropertyException("InfrastructureProfileResponse", "folderPath");
}
this.folderPath = folderPath;
return this;
}
@CustomType.Setter
public Builder instanceUuid(String instanceUuid) {
if (instanceUuid == null) {
throw new MissingRequiredPropertyException("InfrastructureProfileResponse", "instanceUuid");
}
this.instanceUuid = instanceUuid;
return this;
}
@CustomType.Setter
public Builder inventoryItemId(@Nullable String inventoryItemId) {
this.inventoryItemId = inventoryItemId;
return this;
}
@CustomType.Setter
public Builder moName(String moName) {
if (moName == null) {
throw new MissingRequiredPropertyException("InfrastructureProfileResponse", "moName");
}
this.moName = moName;
return this;
}
@CustomType.Setter
public Builder moRefId(String moRefId) {
if (moRefId == null) {
throw new MissingRequiredPropertyException("InfrastructureProfileResponse", "moRefId");
}
this.moRefId = moRefId;
return this;
}
@CustomType.Setter
public Builder smbiosUuid(@Nullable String smbiosUuid) {
this.smbiosUuid = smbiosUuid;
return this;
}
@CustomType.Setter
public Builder templateId(@Nullable String templateId) {
this.templateId = templateId;
return this;
}
@CustomType.Setter
public Builder vCenterId(@Nullable String vCenterId) {
this.vCenterId = vCenterId;
return this;
}
public InfrastructureProfileResponse build() {
final var _resultValue = new InfrastructureProfileResponse();
_resultValue.customResourceName = customResourceName;
_resultValue.firmwareType = firmwareType;
_resultValue.folderPath = folderPath;
_resultValue.instanceUuid = instanceUuid;
_resultValue.inventoryItemId = inventoryItemId;
_resultValue.moName = moName;
_resultValue.moRefId = moRefId;
_resultValue.smbiosUuid = smbiosUuid;
_resultValue.templateId = templateId;
_resultValue.vCenterId = vCenterId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy