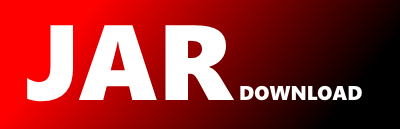
com.pulumi.azurenative.connectedvmwarevsphere.outputs.VirtualDiskResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.connectedvmwarevsphere.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualDiskResponse {
/**
* @return Gets or sets the controller id.
*
*/
private @Nullable Integer controllerKey;
/**
* @return Gets or sets the device key value.
*
*/
private @Nullable Integer deviceKey;
/**
* @return Gets or sets the device name.
*
*/
private @Nullable String deviceName;
/**
* @return Gets or sets the disk mode.
*
*/
private @Nullable String diskMode;
/**
* @return Gets or sets the disk object id.
*
*/
private String diskObjectId;
/**
* @return Gets or sets the disk total size.
*
*/
private @Nullable Integer diskSizeGB;
/**
* @return Gets or sets the disk backing type.
*
*/
private @Nullable String diskType;
/**
* @return Gets or sets the label of the virtual disk in vCenter.
*
*/
private String label;
/**
* @return Gets or sets the name of the virtual disk.
*
*/
private @Nullable String name;
/**
* @return Gets or sets the unit number of the disk on the controller.
*
*/
private @Nullable Integer unitNumber;
private VirtualDiskResponse() {}
/**
* @return Gets or sets the controller id.
*
*/
public Optional controllerKey() {
return Optional.ofNullable(this.controllerKey);
}
/**
* @return Gets or sets the device key value.
*
*/
public Optional deviceKey() {
return Optional.ofNullable(this.deviceKey);
}
/**
* @return Gets or sets the device name.
*
*/
public Optional deviceName() {
return Optional.ofNullable(this.deviceName);
}
/**
* @return Gets or sets the disk mode.
*
*/
public Optional diskMode() {
return Optional.ofNullable(this.diskMode);
}
/**
* @return Gets or sets the disk object id.
*
*/
public String diskObjectId() {
return this.diskObjectId;
}
/**
* @return Gets or sets the disk total size.
*
*/
public Optional diskSizeGB() {
return Optional.ofNullable(this.diskSizeGB);
}
/**
* @return Gets or sets the disk backing type.
*
*/
public Optional diskType() {
return Optional.ofNullable(this.diskType);
}
/**
* @return Gets or sets the label of the virtual disk in vCenter.
*
*/
public String label() {
return this.label;
}
/**
* @return Gets or sets the name of the virtual disk.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Gets or sets the unit number of the disk on the controller.
*
*/
public Optional unitNumber() {
return Optional.ofNullable(this.unitNumber);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualDiskResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer controllerKey;
private @Nullable Integer deviceKey;
private @Nullable String deviceName;
private @Nullable String diskMode;
private String diskObjectId;
private @Nullable Integer diskSizeGB;
private @Nullable String diskType;
private String label;
private @Nullable String name;
private @Nullable Integer unitNumber;
public Builder() {}
public Builder(VirtualDiskResponse defaults) {
Objects.requireNonNull(defaults);
this.controllerKey = defaults.controllerKey;
this.deviceKey = defaults.deviceKey;
this.deviceName = defaults.deviceName;
this.diskMode = defaults.diskMode;
this.diskObjectId = defaults.diskObjectId;
this.diskSizeGB = defaults.diskSizeGB;
this.diskType = defaults.diskType;
this.label = defaults.label;
this.name = defaults.name;
this.unitNumber = defaults.unitNumber;
}
@CustomType.Setter
public Builder controllerKey(@Nullable Integer controllerKey) {
this.controllerKey = controllerKey;
return this;
}
@CustomType.Setter
public Builder deviceKey(@Nullable Integer deviceKey) {
this.deviceKey = deviceKey;
return this;
}
@CustomType.Setter
public Builder deviceName(@Nullable String deviceName) {
this.deviceName = deviceName;
return this;
}
@CustomType.Setter
public Builder diskMode(@Nullable String diskMode) {
this.diskMode = diskMode;
return this;
}
@CustomType.Setter
public Builder diskObjectId(String diskObjectId) {
if (diskObjectId == null) {
throw new MissingRequiredPropertyException("VirtualDiskResponse", "diskObjectId");
}
this.diskObjectId = diskObjectId;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(@Nullable Integer diskSizeGB) {
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder diskType(@Nullable String diskType) {
this.diskType = diskType;
return this;
}
@CustomType.Setter
public Builder label(String label) {
if (label == null) {
throw new MissingRequiredPropertyException("VirtualDiskResponse", "label");
}
this.label = label;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder unitNumber(@Nullable Integer unitNumber) {
this.unitNumber = unitNumber;
return this;
}
public VirtualDiskResponse build() {
final var _resultValue = new VirtualDiskResponse();
_resultValue.controllerKey = controllerKey;
_resultValue.deviceKey = deviceKey;
_resultValue.deviceName = deviceName;
_resultValue.diskMode = diskMode;
_resultValue.diskObjectId = diskObjectId;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.diskType = diskType;
_resultValue.label = label;
_resultValue.name = name;
_resultValue.unitNumber = unitNumber;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy