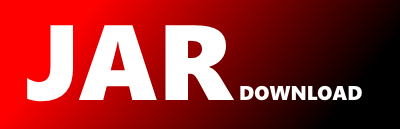
com.pulumi.azurenative.containerregistry.ConnectedRegistryArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry;
import com.pulumi.azurenative.containerregistry.enums.ConnectedRegistryMode;
import com.pulumi.azurenative.containerregistry.inputs.LoggingPropertiesArgs;
import com.pulumi.azurenative.containerregistry.inputs.ParentPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ConnectedRegistryArgs extends com.pulumi.resources.ResourceArgs {
public static final ConnectedRegistryArgs Empty = new ConnectedRegistryArgs();
/**
* The list of the ACR token resource IDs used to authenticate clients to the connected registry.
*
*/
@Import(name="clientTokenIds")
private @Nullable Output> clientTokenIds;
/**
* @return The list of the ACR token resource IDs used to authenticate clients to the connected registry.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy