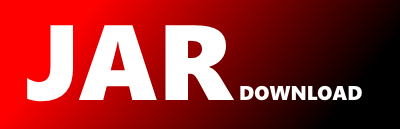
com.pulumi.azurenative.containerregistry.outputs.DockerBuildStepResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry.outputs;
import com.pulumi.azurenative.containerregistry.outputs.ArgumentResponse;
import com.pulumi.azurenative.containerregistry.outputs.BaseImageDependencyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DockerBuildStepResponse {
/**
* @return The collection of override arguments to be used when executing this build step.
*
*/
private @Nullable List arguments;
/**
* @return List of base image dependencies for a step.
*
*/
private List baseImageDependencies;
/**
* @return The token (git PAT or SAS token of storage account blob) associated with the context for a step.
*
*/
private @Nullable String contextAccessToken;
/**
* @return The URL(absolute or relative) of the source context for the task step.
*
*/
private @Nullable String contextPath;
/**
* @return The Docker file path relative to the source context.
*
*/
private String dockerFilePath;
/**
* @return The fully qualified image names including the repository and tag.
*
*/
private @Nullable List imageNames;
/**
* @return The value of this property indicates whether the image built should be pushed to the registry or not.
*
*/
private @Nullable Boolean isPushEnabled;
/**
* @return The value of this property indicates whether the image cache is enabled or not.
*
*/
private @Nullable Boolean noCache;
/**
* @return The name of the target build stage for the docker build.
*
*/
private @Nullable String target;
/**
* @return The type of the step.
* Expected value is 'Docker'.
*
*/
private String type;
private DockerBuildStepResponse() {}
/**
* @return The collection of override arguments to be used when executing this build step.
*
*/
public List arguments() {
return this.arguments == null ? List.of() : this.arguments;
}
/**
* @return List of base image dependencies for a step.
*
*/
public List baseImageDependencies() {
return this.baseImageDependencies;
}
/**
* @return The token (git PAT or SAS token of storage account blob) associated with the context for a step.
*
*/
public Optional contextAccessToken() {
return Optional.ofNullable(this.contextAccessToken);
}
/**
* @return The URL(absolute or relative) of the source context for the task step.
*
*/
public Optional contextPath() {
return Optional.ofNullable(this.contextPath);
}
/**
* @return The Docker file path relative to the source context.
*
*/
public String dockerFilePath() {
return this.dockerFilePath;
}
/**
* @return The fully qualified image names including the repository and tag.
*
*/
public List imageNames() {
return this.imageNames == null ? List.of() : this.imageNames;
}
/**
* @return The value of this property indicates whether the image built should be pushed to the registry or not.
*
*/
public Optional isPushEnabled() {
return Optional.ofNullable(this.isPushEnabled);
}
/**
* @return The value of this property indicates whether the image cache is enabled or not.
*
*/
public Optional noCache() {
return Optional.ofNullable(this.noCache);
}
/**
* @return The name of the target build stage for the docker build.
*
*/
public Optional target() {
return Optional.ofNullable(this.target);
}
/**
* @return The type of the step.
* Expected value is 'Docker'.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DockerBuildStepResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List arguments;
private List baseImageDependencies;
private @Nullable String contextAccessToken;
private @Nullable String contextPath;
private String dockerFilePath;
private @Nullable List imageNames;
private @Nullable Boolean isPushEnabled;
private @Nullable Boolean noCache;
private @Nullable String target;
private String type;
public Builder() {}
public Builder(DockerBuildStepResponse defaults) {
Objects.requireNonNull(defaults);
this.arguments = defaults.arguments;
this.baseImageDependencies = defaults.baseImageDependencies;
this.contextAccessToken = defaults.contextAccessToken;
this.contextPath = defaults.contextPath;
this.dockerFilePath = defaults.dockerFilePath;
this.imageNames = defaults.imageNames;
this.isPushEnabled = defaults.isPushEnabled;
this.noCache = defaults.noCache;
this.target = defaults.target;
this.type = defaults.type;
}
@CustomType.Setter
public Builder arguments(@Nullable List arguments) {
this.arguments = arguments;
return this;
}
public Builder arguments(ArgumentResponse... arguments) {
return arguments(List.of(arguments));
}
@CustomType.Setter
public Builder baseImageDependencies(List baseImageDependencies) {
if (baseImageDependencies == null) {
throw new MissingRequiredPropertyException("DockerBuildStepResponse", "baseImageDependencies");
}
this.baseImageDependencies = baseImageDependencies;
return this;
}
public Builder baseImageDependencies(BaseImageDependencyResponse... baseImageDependencies) {
return baseImageDependencies(List.of(baseImageDependencies));
}
@CustomType.Setter
public Builder contextAccessToken(@Nullable String contextAccessToken) {
this.contextAccessToken = contextAccessToken;
return this;
}
@CustomType.Setter
public Builder contextPath(@Nullable String contextPath) {
this.contextPath = contextPath;
return this;
}
@CustomType.Setter
public Builder dockerFilePath(String dockerFilePath) {
if (dockerFilePath == null) {
throw new MissingRequiredPropertyException("DockerBuildStepResponse", "dockerFilePath");
}
this.dockerFilePath = dockerFilePath;
return this;
}
@CustomType.Setter
public Builder imageNames(@Nullable List imageNames) {
this.imageNames = imageNames;
return this;
}
public Builder imageNames(String... imageNames) {
return imageNames(List.of(imageNames));
}
@CustomType.Setter
public Builder isPushEnabled(@Nullable Boolean isPushEnabled) {
this.isPushEnabled = isPushEnabled;
return this;
}
@CustomType.Setter
public Builder noCache(@Nullable Boolean noCache) {
this.noCache = noCache;
return this;
}
@CustomType.Setter
public Builder target(@Nullable String target) {
this.target = target;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("DockerBuildStepResponse", "type");
}
this.type = type;
return this;
}
public DockerBuildStepResponse build() {
final var _resultValue = new DockerBuildStepResponse();
_resultValue.arguments = arguments;
_resultValue.baseImageDependencies = baseImageDependencies;
_resultValue.contextAccessToken = contextAccessToken;
_resultValue.contextPath = contextPath;
_resultValue.dockerFilePath = dockerFilePath;
_resultValue.imageNames = imageNames;
_resultValue.isPushEnabled = isPushEnabled;
_resultValue.noCache = noCache;
_resultValue.target = target;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy