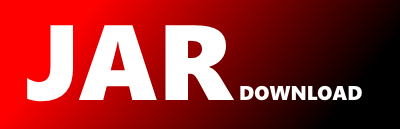
com.pulumi.azurenative.containerregistry.outputs.RunResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry.outputs;
import com.pulumi.azurenative.containerregistry.outputs.AgentPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.ImageDescriptorResponse;
import com.pulumi.azurenative.containerregistry.outputs.ImageUpdateTriggerResponse;
import com.pulumi.azurenative.containerregistry.outputs.PlatformPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.SourceTriggerDescriptorResponse;
import com.pulumi.azurenative.containerregistry.outputs.SystemDataResponse;
import com.pulumi.azurenative.containerregistry.outputs.TimerTriggerDescriptorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class RunResponse {
/**
* @return The machine configuration of the run agent.
*
*/
private @Nullable AgentPropertiesResponse agentConfiguration;
/**
* @return The dedicated agent pool for the run.
*
*/
private @Nullable String agentPoolName;
/**
* @return The time the run was scheduled.
*
*/
private @Nullable String createTime;
/**
* @return The list of custom registries that were logged in during this run.
*
*/
private @Nullable List customRegistries;
/**
* @return The time the run finished.
*
*/
private @Nullable String finishTime;
/**
* @return The resource ID.
*
*/
private String id;
/**
* @return The image update trigger that caused the run. This is applicable if the task has base image trigger configured.
*
*/
private @Nullable ImageUpdateTriggerResponse imageUpdateTrigger;
/**
* @return The value that indicates whether archiving is enabled or not.
*
*/
private @Nullable Boolean isArchiveEnabled;
/**
* @return The last updated time for the run.
*
*/
private @Nullable String lastUpdatedTime;
/**
* @return The image description for the log artifact.
*
*/
private ImageDescriptorResponse logArtifact;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return The list of all images that were generated from the run. This is applicable if the run generates base image dependencies.
*
*/
private @Nullable List outputImages;
/**
* @return The platform properties against which the run will happen.
*
*/
private @Nullable PlatformPropertiesResponse platform;
/**
* @return The provisioning state of a run.
*
*/
private @Nullable String provisioningState;
/**
* @return The error message received from backend systems after the run is scheduled.
*
*/
private String runErrorMessage;
/**
* @return The unique identifier for the run.
*
*/
private @Nullable String runId;
/**
* @return The type of run.
*
*/
private @Nullable String runType;
/**
* @return The scope of the credentials that were used to login to the source registry during this run.
*
*/
private @Nullable String sourceRegistryAuth;
/**
* @return The source trigger that caused the run.
*
*/
private @Nullable SourceTriggerDescriptorResponse sourceTrigger;
/**
* @return The time the run started.
*
*/
private @Nullable String startTime;
/**
* @return The current status of the run.
*
*/
private @Nullable String status;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The task against which run was scheduled.
*
*/
private @Nullable String task;
/**
* @return The timer trigger that caused the run.
*
*/
private @Nullable TimerTriggerDescriptorResponse timerTrigger;
/**
* @return The type of the resource.
*
*/
private String type;
/**
* @return The update trigger token passed for the Run.
*
*/
private @Nullable String updateTriggerToken;
private RunResponse() {}
/**
* @return The machine configuration of the run agent.
*
*/
public Optional agentConfiguration() {
return Optional.ofNullable(this.agentConfiguration);
}
/**
* @return The dedicated agent pool for the run.
*
*/
public Optional agentPoolName() {
return Optional.ofNullable(this.agentPoolName);
}
/**
* @return The time the run was scheduled.
*
*/
public Optional createTime() {
return Optional.ofNullable(this.createTime);
}
/**
* @return The list of custom registries that were logged in during this run.
*
*/
public List customRegistries() {
return this.customRegistries == null ? List.of() : this.customRegistries;
}
/**
* @return The time the run finished.
*
*/
public Optional finishTime() {
return Optional.ofNullable(this.finishTime);
}
/**
* @return The resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The image update trigger that caused the run. This is applicable if the task has base image trigger configured.
*
*/
public Optional imageUpdateTrigger() {
return Optional.ofNullable(this.imageUpdateTrigger);
}
/**
* @return The value that indicates whether archiving is enabled or not.
*
*/
public Optional isArchiveEnabled() {
return Optional.ofNullable(this.isArchiveEnabled);
}
/**
* @return The last updated time for the run.
*
*/
public Optional lastUpdatedTime() {
return Optional.ofNullable(this.lastUpdatedTime);
}
/**
* @return The image description for the log artifact.
*
*/
public ImageDescriptorResponse logArtifact() {
return this.logArtifact;
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The list of all images that were generated from the run. This is applicable if the run generates base image dependencies.
*
*/
public List outputImages() {
return this.outputImages == null ? List.of() : this.outputImages;
}
/**
* @return The platform properties against which the run will happen.
*
*/
public Optional platform() {
return Optional.ofNullable(this.platform);
}
/**
* @return The provisioning state of a run.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return The error message received from backend systems after the run is scheduled.
*
*/
public String runErrorMessage() {
return this.runErrorMessage;
}
/**
* @return The unique identifier for the run.
*
*/
public Optional runId() {
return Optional.ofNullable(this.runId);
}
/**
* @return The type of run.
*
*/
public Optional runType() {
return Optional.ofNullable(this.runType);
}
/**
* @return The scope of the credentials that were used to login to the source registry during this run.
*
*/
public Optional sourceRegistryAuth() {
return Optional.ofNullable(this.sourceRegistryAuth);
}
/**
* @return The source trigger that caused the run.
*
*/
public Optional sourceTrigger() {
return Optional.ofNullable(this.sourceTrigger);
}
/**
* @return The time the run started.
*
*/
public Optional startTime() {
return Optional.ofNullable(this.startTime);
}
/**
* @return The current status of the run.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The task against which run was scheduled.
*
*/
public Optional task() {
return Optional.ofNullable(this.task);
}
/**
* @return The timer trigger that caused the run.
*
*/
public Optional timerTrigger() {
return Optional.ofNullable(this.timerTrigger);
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The update trigger token passed for the Run.
*
*/
public Optional updateTriggerToken() {
return Optional.ofNullable(this.updateTriggerToken);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RunResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AgentPropertiesResponse agentConfiguration;
private @Nullable String agentPoolName;
private @Nullable String createTime;
private @Nullable List customRegistries;
private @Nullable String finishTime;
private String id;
private @Nullable ImageUpdateTriggerResponse imageUpdateTrigger;
private @Nullable Boolean isArchiveEnabled;
private @Nullable String lastUpdatedTime;
private ImageDescriptorResponse logArtifact;
private String name;
private @Nullable List outputImages;
private @Nullable PlatformPropertiesResponse platform;
private @Nullable String provisioningState;
private String runErrorMessage;
private @Nullable String runId;
private @Nullable String runType;
private @Nullable String sourceRegistryAuth;
private @Nullable SourceTriggerDescriptorResponse sourceTrigger;
private @Nullable String startTime;
private @Nullable String status;
private SystemDataResponse systemData;
private @Nullable String task;
private @Nullable TimerTriggerDescriptorResponse timerTrigger;
private String type;
private @Nullable String updateTriggerToken;
public Builder() {}
public Builder(RunResponse defaults) {
Objects.requireNonNull(defaults);
this.agentConfiguration = defaults.agentConfiguration;
this.agentPoolName = defaults.agentPoolName;
this.createTime = defaults.createTime;
this.customRegistries = defaults.customRegistries;
this.finishTime = defaults.finishTime;
this.id = defaults.id;
this.imageUpdateTrigger = defaults.imageUpdateTrigger;
this.isArchiveEnabled = defaults.isArchiveEnabled;
this.lastUpdatedTime = defaults.lastUpdatedTime;
this.logArtifact = defaults.logArtifact;
this.name = defaults.name;
this.outputImages = defaults.outputImages;
this.platform = defaults.platform;
this.provisioningState = defaults.provisioningState;
this.runErrorMessage = defaults.runErrorMessage;
this.runId = defaults.runId;
this.runType = defaults.runType;
this.sourceRegistryAuth = defaults.sourceRegistryAuth;
this.sourceTrigger = defaults.sourceTrigger;
this.startTime = defaults.startTime;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.task = defaults.task;
this.timerTrigger = defaults.timerTrigger;
this.type = defaults.type;
this.updateTriggerToken = defaults.updateTriggerToken;
}
@CustomType.Setter
public Builder agentConfiguration(@Nullable AgentPropertiesResponse agentConfiguration) {
this.agentConfiguration = agentConfiguration;
return this;
}
@CustomType.Setter
public Builder agentPoolName(@Nullable String agentPoolName) {
this.agentPoolName = agentPoolName;
return this;
}
@CustomType.Setter
public Builder createTime(@Nullable String createTime) {
this.createTime = createTime;
return this;
}
@CustomType.Setter
public Builder customRegistries(@Nullable List customRegistries) {
this.customRegistries = customRegistries;
return this;
}
public Builder customRegistries(String... customRegistries) {
return customRegistries(List.of(customRegistries));
}
@CustomType.Setter
public Builder finishTime(@Nullable String finishTime) {
this.finishTime = finishTime;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("RunResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder imageUpdateTrigger(@Nullable ImageUpdateTriggerResponse imageUpdateTrigger) {
this.imageUpdateTrigger = imageUpdateTrigger;
return this;
}
@CustomType.Setter
public Builder isArchiveEnabled(@Nullable Boolean isArchiveEnabled) {
this.isArchiveEnabled = isArchiveEnabled;
return this;
}
@CustomType.Setter
public Builder lastUpdatedTime(@Nullable String lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
return this;
}
@CustomType.Setter
public Builder logArtifact(ImageDescriptorResponse logArtifact) {
if (logArtifact == null) {
throw new MissingRequiredPropertyException("RunResponse", "logArtifact");
}
this.logArtifact = logArtifact;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("RunResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder outputImages(@Nullable List outputImages) {
this.outputImages = outputImages;
return this;
}
public Builder outputImages(ImageDescriptorResponse... outputImages) {
return outputImages(List.of(outputImages));
}
@CustomType.Setter
public Builder platform(@Nullable PlatformPropertiesResponse platform) {
this.platform = platform;
return this;
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder runErrorMessage(String runErrorMessage) {
if (runErrorMessage == null) {
throw new MissingRequiredPropertyException("RunResponse", "runErrorMessage");
}
this.runErrorMessage = runErrorMessage;
return this;
}
@CustomType.Setter
public Builder runId(@Nullable String runId) {
this.runId = runId;
return this;
}
@CustomType.Setter
public Builder runType(@Nullable String runType) {
this.runType = runType;
return this;
}
@CustomType.Setter
public Builder sourceRegistryAuth(@Nullable String sourceRegistryAuth) {
this.sourceRegistryAuth = sourceRegistryAuth;
return this;
}
@CustomType.Setter
public Builder sourceTrigger(@Nullable SourceTriggerDescriptorResponse sourceTrigger) {
this.sourceTrigger = sourceTrigger;
return this;
}
@CustomType.Setter
public Builder startTime(@Nullable String startTime) {
this.startTime = startTime;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("RunResponse", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder task(@Nullable String task) {
this.task = task;
return this;
}
@CustomType.Setter
public Builder timerTrigger(@Nullable TimerTriggerDescriptorResponse timerTrigger) {
this.timerTrigger = timerTrigger;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("RunResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder updateTriggerToken(@Nullable String updateTriggerToken) {
this.updateTriggerToken = updateTriggerToken;
return this;
}
public RunResponse build() {
final var _resultValue = new RunResponse();
_resultValue.agentConfiguration = agentConfiguration;
_resultValue.agentPoolName = agentPoolName;
_resultValue.createTime = createTime;
_resultValue.customRegistries = customRegistries;
_resultValue.finishTime = finishTime;
_resultValue.id = id;
_resultValue.imageUpdateTrigger = imageUpdateTrigger;
_resultValue.isArchiveEnabled = isArchiveEnabled;
_resultValue.lastUpdatedTime = lastUpdatedTime;
_resultValue.logArtifact = logArtifact;
_resultValue.name = name;
_resultValue.outputImages = outputImages;
_resultValue.platform = platform;
_resultValue.provisioningState = provisioningState;
_resultValue.runErrorMessage = runErrorMessage;
_resultValue.runId = runId;
_resultValue.runType = runType;
_resultValue.sourceRegistryAuth = sourceRegistryAuth;
_resultValue.sourceTrigger = sourceTrigger;
_resultValue.startTime = startTime;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.task = task;
_resultValue.timerTrigger = timerTrigger;
_resultValue.type = type;
_resultValue.updateTriggerToken = updateTriggerToken;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy