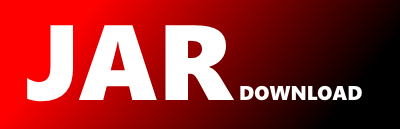
com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement.inputs;
import com.pulumi.azurenative.costmanagement.enums.ExportType;
import com.pulumi.azurenative.costmanagement.enums.TimeframeType;
import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
import com.pulumi.azurenative.costmanagement.inputs.ExportTimePeriodArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The definition of an export.
*
*/
public final class ExportDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final ExportDefinitionArgs Empty = new ExportDefinitionArgs();
/**
* The definition for data in the export.
*
*/
@Import(name="dataSet")
private @Nullable Output dataSet;
/**
* @return The definition for data in the export.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy