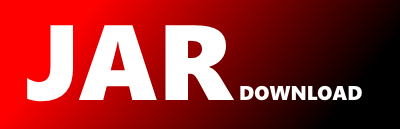
com.pulumi.azurenative.costmanagement.outputs.ExportRunResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement.outputs;
import com.pulumi.azurenative.costmanagement.outputs.CommonExportPropertiesResponse;
import com.pulumi.azurenative.costmanagement.outputs.ErrorDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ExportRunResponse {
/**
* @return eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
private @Nullable String eTag;
/**
* @return The details of any error.
*
*/
private @Nullable ErrorDetailsResponse error;
/**
* @return The type of the export run.
*
*/
private @Nullable String executionType;
/**
* @return The name of the exported file.
*
*/
private @Nullable String fileName;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The time when the export run finished.
*
*/
private @Nullable String processingEndTime;
/**
* @return The time when export was picked up to be run.
*
*/
private @Nullable String processingStartTime;
/**
* @return The export settings that were in effect for this run.
*
*/
private @Nullable CommonExportPropertiesResponse runSettings;
/**
* @return The last known status of the export run.
*
*/
private @Nullable String status;
/**
* @return The identifier for the entity that triggered the export. For on-demand runs it is the user email. For scheduled runs it is 'System'.
*
*/
private @Nullable String submittedBy;
/**
* @return The time when export was queued to be run.
*
*/
private @Nullable String submittedTime;
/**
* @return Resource type.
*
*/
private String type;
private ExportRunResponse() {}
/**
* @return eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
public Optional eTag() {
return Optional.ofNullable(this.eTag);
}
/**
* @return The details of any error.
*
*/
public Optional error() {
return Optional.ofNullable(this.error);
}
/**
* @return The type of the export run.
*
*/
public Optional executionType() {
return Optional.ofNullable(this.executionType);
}
/**
* @return The name of the exported file.
*
*/
public Optional fileName() {
return Optional.ofNullable(this.fileName);
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The time when the export run finished.
*
*/
public Optional processingEndTime() {
return Optional.ofNullable(this.processingEndTime);
}
/**
* @return The time when export was picked up to be run.
*
*/
public Optional processingStartTime() {
return Optional.ofNullable(this.processingStartTime);
}
/**
* @return The export settings that were in effect for this run.
*
*/
public Optional runSettings() {
return Optional.ofNullable(this.runSettings);
}
/**
* @return The last known status of the export run.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return The identifier for the entity that triggered the export. For on-demand runs it is the user email. For scheduled runs it is 'System'.
*
*/
public Optional submittedBy() {
return Optional.ofNullable(this.submittedBy);
}
/**
* @return The time when export was queued to be run.
*
*/
public Optional submittedTime() {
return Optional.ofNullable(this.submittedTime);
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ExportRunResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String eTag;
private @Nullable ErrorDetailsResponse error;
private @Nullable String executionType;
private @Nullable String fileName;
private String id;
private String name;
private @Nullable String processingEndTime;
private @Nullable String processingStartTime;
private @Nullable CommonExportPropertiesResponse runSettings;
private @Nullable String status;
private @Nullable String submittedBy;
private @Nullable String submittedTime;
private String type;
public Builder() {}
public Builder(ExportRunResponse defaults) {
Objects.requireNonNull(defaults);
this.eTag = defaults.eTag;
this.error = defaults.error;
this.executionType = defaults.executionType;
this.fileName = defaults.fileName;
this.id = defaults.id;
this.name = defaults.name;
this.processingEndTime = defaults.processingEndTime;
this.processingStartTime = defaults.processingStartTime;
this.runSettings = defaults.runSettings;
this.status = defaults.status;
this.submittedBy = defaults.submittedBy;
this.submittedTime = defaults.submittedTime;
this.type = defaults.type;
}
@CustomType.Setter
public Builder eTag(@Nullable String eTag) {
this.eTag = eTag;
return this;
}
@CustomType.Setter
public Builder error(@Nullable ErrorDetailsResponse error) {
this.error = error;
return this;
}
@CustomType.Setter
public Builder executionType(@Nullable String executionType) {
this.executionType = executionType;
return this;
}
@CustomType.Setter
public Builder fileName(@Nullable String fileName) {
this.fileName = fileName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("ExportRunResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("ExportRunResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder processingEndTime(@Nullable String processingEndTime) {
this.processingEndTime = processingEndTime;
return this;
}
@CustomType.Setter
public Builder processingStartTime(@Nullable String processingStartTime) {
this.processingStartTime = processingStartTime;
return this;
}
@CustomType.Setter
public Builder runSettings(@Nullable CommonExportPropertiesResponse runSettings) {
this.runSettings = runSettings;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder submittedBy(@Nullable String submittedBy) {
this.submittedBy = submittedBy;
return this;
}
@CustomType.Setter
public Builder submittedTime(@Nullable String submittedTime) {
this.submittedTime = submittedTime;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ExportRunResponse", "type");
}
this.type = type;
return this;
}
public ExportRunResponse build() {
final var _resultValue = new ExportRunResponse();
_resultValue.eTag = eTag;
_resultValue.error = error;
_resultValue.executionType = executionType;
_resultValue.fileName = fileName;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.processingEndTime = processingEndTime;
_resultValue.processingStartTime = processingStartTime;
_resultValue.runSettings = runSettings;
_resultValue.status = status;
_resultValue.submittedBy = submittedBy;
_resultValue.submittedTime = submittedTime;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy