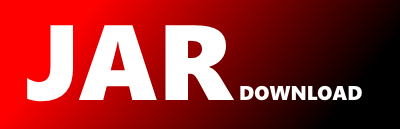
com.pulumi.azurenative.costmanagement.outputs.GetScheduledActionByScopeResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement.outputs;
import com.pulumi.azurenative.costmanagement.outputs.FileDestinationResponse;
import com.pulumi.azurenative.costmanagement.outputs.NotificationPropertiesResponse;
import com.pulumi.azurenative.costmanagement.outputs.SchedulePropertiesResponse;
import com.pulumi.azurenative.costmanagement.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetScheduledActionByScopeResult {
/**
* @return Scheduled action name.
*
*/
private String displayName;
/**
* @return Resource Etag. For update calls, eTag is optional and can be specified to achieve optimistic concurrency. Fetch the resource's eTag by doing a 'GET' call first and then including the latest eTag as part of the request body or 'If-Match' header while performing the update. For create calls, eTag is not required.
*
*/
private String eTag;
/**
* @return Destination format of the view data. This is optional.
*
*/
private @Nullable FileDestinationResponse fileDestination;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Kind of the scheduled action.
*
*/
private @Nullable String kind;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Notification properties based on scheduled action kind.
*
*/
private NotificationPropertiesResponse notification;
/**
* @return Email address of the point of contact that should get the unsubscribe requests and notification emails.
*
*/
private @Nullable String notificationEmail;
/**
* @return Schedule of the scheduled action.
*
*/
private SchedulePropertiesResponse schedule;
/**
* @return For private scheduled action(Create or Update), scope will be empty.<br /> For shared scheduled action(Create or Update By Scope), Cost Management scope can be 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
*
*/
private @Nullable String scope;
/**
* @return Status of the scheduled action.
*
*/
private String status;
/**
* @return Kind of the scheduled action.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Cost analysis viewId used for scheduled action. For example, '/providers/Microsoft.CostManagement/views/swaggerExample'
*
*/
private String viewId;
private GetScheduledActionByScopeResult() {}
/**
* @return Scheduled action name.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Resource Etag. For update calls, eTag is optional and can be specified to achieve optimistic concurrency. Fetch the resource's eTag by doing a 'GET' call first and then including the latest eTag as part of the request body or 'If-Match' header while performing the update. For create calls, eTag is not required.
*
*/
public String eTag() {
return this.eTag;
}
/**
* @return Destination format of the view data. This is optional.
*
*/
public Optional fileDestination() {
return Optional.ofNullable(this.fileDestination);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Kind of the scheduled action.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Notification properties based on scheduled action kind.
*
*/
public NotificationPropertiesResponse notification() {
return this.notification;
}
/**
* @return Email address of the point of contact that should get the unsubscribe requests and notification emails.
*
*/
public Optional notificationEmail() {
return Optional.ofNullable(this.notificationEmail);
}
/**
* @return Schedule of the scheduled action.
*
*/
public SchedulePropertiesResponse schedule() {
return this.schedule;
}
/**
* @return For private scheduled action(Create or Update), scope will be empty.<br /> For shared scheduled action(Create or Update By Scope), Cost Management scope can be 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
*
*/
public Optional scope() {
return Optional.ofNullable(this.scope);
}
/**
* @return Status of the scheduled action.
*
*/
public String status() {
return this.status;
}
/**
* @return Kind of the scheduled action.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Cost analysis viewId used for scheduled action. For example, '/providers/Microsoft.CostManagement/views/swaggerExample'
*
*/
public String viewId() {
return this.viewId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetScheduledActionByScopeResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String displayName;
private String eTag;
private @Nullable FileDestinationResponse fileDestination;
private String id;
private @Nullable String kind;
private String name;
private NotificationPropertiesResponse notification;
private @Nullable String notificationEmail;
private SchedulePropertiesResponse schedule;
private @Nullable String scope;
private String status;
private SystemDataResponse systemData;
private String type;
private String viewId;
public Builder() {}
public Builder(GetScheduledActionByScopeResult defaults) {
Objects.requireNonNull(defaults);
this.displayName = defaults.displayName;
this.eTag = defaults.eTag;
this.fileDestination = defaults.fileDestination;
this.id = defaults.id;
this.kind = defaults.kind;
this.name = defaults.name;
this.notification = defaults.notification;
this.notificationEmail = defaults.notificationEmail;
this.schedule = defaults.schedule;
this.scope = defaults.scope;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.viewId = defaults.viewId;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder eTag(String eTag) {
if (eTag == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "eTag");
}
this.eTag = eTag;
return this;
}
@CustomType.Setter
public Builder fileDestination(@Nullable FileDestinationResponse fileDestination) {
this.fileDestination = fileDestination;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder notification(NotificationPropertiesResponse notification) {
if (notification == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "notification");
}
this.notification = notification;
return this;
}
@CustomType.Setter
public Builder notificationEmail(@Nullable String notificationEmail) {
this.notificationEmail = notificationEmail;
return this;
}
@CustomType.Setter
public Builder schedule(SchedulePropertiesResponse schedule) {
if (schedule == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "schedule");
}
this.schedule = schedule;
return this;
}
@CustomType.Setter
public Builder scope(@Nullable String scope) {
this.scope = scope;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder viewId(String viewId) {
if (viewId == null) {
throw new MissingRequiredPropertyException("GetScheduledActionByScopeResult", "viewId");
}
this.viewId = viewId;
return this;
}
public GetScheduledActionByScopeResult build() {
final var _resultValue = new GetScheduledActionByScopeResult();
_resultValue.displayName = displayName;
_resultValue.eTag = eTag;
_resultValue.fileDestination = fileDestination;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.name = name;
_resultValue.notification = notification;
_resultValue.notificationEmail = notificationEmail;
_resultValue.schedule = schedule;
_resultValue.scope = scope;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.viewId = viewId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy