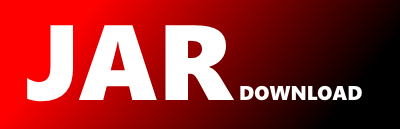
com.pulumi.azurenative.costmanagement.outputs.NotificationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NotificationResponse {
/**
* @return Email addresses to send the notification to when the threshold is breached. Must have at least one contact email or contact group specified at the Subscription or Resource Group scopes. All other scopes must have at least one contact email specified.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
private List contactEmails;
/**
* @return Subscription or Resource Group scopes only. Action groups to send the notification to when the threshold is exceeded. Must be provided as a fully qualified Azure resource id.
*
* Supported for CategoryType(s): Cost.
*
*/
private @Nullable List contactGroups;
/**
* @return Subscription or Resource Group scopes only. Contact roles to send the notification to when the threshold is breached.
*
* Supported for CategoryType(s): Cost.
*
*/
private @Nullable List contactRoles;
/**
* @return The notification is enabled or not.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
private Boolean enabled;
/**
* @return Frequency of a notification. Represents how long the notification will be silent after triggering an alert for a threshold breach. If not specified, the frequency will be set by default based on the timeGrain (Weekly when timeGrain: Last7Days, Monthly when timeGrain: Last30Days).
*
* Supported for CategoryType(s): ReservationUtilization.
*
*/
private @Nullable String frequency;
/**
* @return Language in which the recipient will receive the notification,
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
private @Nullable String locale;
/**
* @return The comparison operator.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* Supported operators for **CategoryType: Cost**
* - GreaterThan
* - GreaterThanOrEqualTo
*
* Supported operators for **CategoryType: ReservationUtilization**
* - LessThan
*
*/
private String operator;
/**
* @return Threshold value associated with a notification. It is always percent with a maximum of 2 decimal places.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* **CategoryType: Cost** - Must be between 0 and 1000. Notification is sent when the cost exceeded the threshold.
*
* **CategoryType: ReservationUtilization** - Must be between 0 and 100. Notification is sent when a reservation has a utilization percentage below the threshold.
*
*/
private Double threshold;
/**
* @return The type of threshold.
*
* Supported for CategoryType(s): Cost.
*
*/
private @Nullable String thresholdType;
private NotificationResponse() {}
/**
* @return Email addresses to send the notification to when the threshold is breached. Must have at least one contact email or contact group specified at the Subscription or Resource Group scopes. All other scopes must have at least one contact email specified.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
public List contactEmails() {
return this.contactEmails;
}
/**
* @return Subscription or Resource Group scopes only. Action groups to send the notification to when the threshold is exceeded. Must be provided as a fully qualified Azure resource id.
*
* Supported for CategoryType(s): Cost.
*
*/
public List contactGroups() {
return this.contactGroups == null ? List.of() : this.contactGroups;
}
/**
* @return Subscription or Resource Group scopes only. Contact roles to send the notification to when the threshold is breached.
*
* Supported for CategoryType(s): Cost.
*
*/
public List contactRoles() {
return this.contactRoles == null ? List.of() : this.contactRoles;
}
/**
* @return The notification is enabled or not.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
public Boolean enabled() {
return this.enabled;
}
/**
* @return Frequency of a notification. Represents how long the notification will be silent after triggering an alert for a threshold breach. If not specified, the frequency will be set by default based on the timeGrain (Weekly when timeGrain: Last7Days, Monthly when timeGrain: Last30Days).
*
* Supported for CategoryType(s): ReservationUtilization.
*
*/
public Optional frequency() {
return Optional.ofNullable(this.frequency);
}
/**
* @return Language in which the recipient will receive the notification,
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
public Optional locale() {
return Optional.ofNullable(this.locale);
}
/**
* @return The comparison operator.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* Supported operators for **CategoryType: Cost**
* - GreaterThan
* - GreaterThanOrEqualTo
*
* Supported operators for **CategoryType: ReservationUtilization**
* - LessThan
*
*/
public String operator() {
return this.operator;
}
/**
* @return Threshold value associated with a notification. It is always percent with a maximum of 2 decimal places.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* **CategoryType: Cost** - Must be between 0 and 1000. Notification is sent when the cost exceeded the threshold.
*
* **CategoryType: ReservationUtilization** - Must be between 0 and 100. Notification is sent when a reservation has a utilization percentage below the threshold.
*
*/
public Double threshold() {
return this.threshold;
}
/**
* @return The type of threshold.
*
* Supported for CategoryType(s): Cost.
*
*/
public Optional thresholdType() {
return Optional.ofNullable(this.thresholdType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NotificationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List contactEmails;
private @Nullable List contactGroups;
private @Nullable List contactRoles;
private Boolean enabled;
private @Nullable String frequency;
private @Nullable String locale;
private String operator;
private Double threshold;
private @Nullable String thresholdType;
public Builder() {}
public Builder(NotificationResponse defaults) {
Objects.requireNonNull(defaults);
this.contactEmails = defaults.contactEmails;
this.contactGroups = defaults.contactGroups;
this.contactRoles = defaults.contactRoles;
this.enabled = defaults.enabled;
this.frequency = defaults.frequency;
this.locale = defaults.locale;
this.operator = defaults.operator;
this.threshold = defaults.threshold;
this.thresholdType = defaults.thresholdType;
}
@CustomType.Setter
public Builder contactEmails(List contactEmails) {
if (contactEmails == null) {
throw new MissingRequiredPropertyException("NotificationResponse", "contactEmails");
}
this.contactEmails = contactEmails;
return this;
}
public Builder contactEmails(String... contactEmails) {
return contactEmails(List.of(contactEmails));
}
@CustomType.Setter
public Builder contactGroups(@Nullable List contactGroups) {
this.contactGroups = contactGroups;
return this;
}
public Builder contactGroups(String... contactGroups) {
return contactGroups(List.of(contactGroups));
}
@CustomType.Setter
public Builder contactRoles(@Nullable List contactRoles) {
this.contactRoles = contactRoles;
return this;
}
public Builder contactRoles(String... contactRoles) {
return contactRoles(List.of(contactRoles));
}
@CustomType.Setter
public Builder enabled(Boolean enabled) {
if (enabled == null) {
throw new MissingRequiredPropertyException("NotificationResponse", "enabled");
}
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder frequency(@Nullable String frequency) {
this.frequency = frequency;
return this;
}
@CustomType.Setter
public Builder locale(@Nullable String locale) {
this.locale = locale;
return this;
}
@CustomType.Setter
public Builder operator(String operator) {
if (operator == null) {
throw new MissingRequiredPropertyException("NotificationResponse", "operator");
}
this.operator = operator;
return this;
}
@CustomType.Setter
public Builder threshold(Double threshold) {
if (threshold == null) {
throw new MissingRequiredPropertyException("NotificationResponse", "threshold");
}
this.threshold = threshold;
return this;
}
@CustomType.Setter
public Builder thresholdType(@Nullable String thresholdType) {
this.thresholdType = thresholdType;
return this;
}
public NotificationResponse build() {
final var _resultValue = new NotificationResponse();
_resultValue.contactEmails = contactEmails;
_resultValue.contactGroups = contactGroups;
_resultValue.contactRoles = contactRoles;
_resultValue.enabled = enabled;
_resultValue.frequency = frequency;
_resultValue.locale = locale;
_resultValue.operator = operator;
_resultValue.threshold = threshold;
_resultValue.thresholdType = thresholdType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy