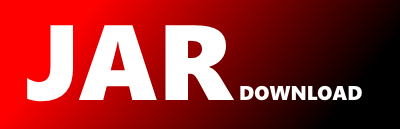
com.pulumi.azurenative.customerinsights.CustomerinsightsFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.customerinsights.inputs.GetConnectorArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetConnectorMappingArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetConnectorMappingPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetConnectorPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetHubArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetHubPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetImageUploadUrlForDataArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetImageUploadUrlForDataPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetImageUploadUrlForEntityTypeArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetImageUploadUrlForEntityTypePlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetKpiArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetKpiPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetLinkArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetLinkPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetPredictionArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetPredictionModelStatusArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetPredictionModelStatusPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetPredictionPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetPredictionTrainingResultsArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetPredictionTrainingResultsPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetProfileArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetProfilePlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetRelationshipArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetRelationshipLinkArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetRelationshipLinkPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetRelationshipPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetRoleAssignmentArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetRoleAssignmentPlainArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetViewArgs;
import com.pulumi.azurenative.customerinsights.inputs.GetViewPlainArgs;
import com.pulumi.azurenative.customerinsights.outputs.GetConnectorMappingResult;
import com.pulumi.azurenative.customerinsights.outputs.GetConnectorResult;
import com.pulumi.azurenative.customerinsights.outputs.GetHubResult;
import com.pulumi.azurenative.customerinsights.outputs.GetImageUploadUrlForDataResult;
import com.pulumi.azurenative.customerinsights.outputs.GetImageUploadUrlForEntityTypeResult;
import com.pulumi.azurenative.customerinsights.outputs.GetKpiResult;
import com.pulumi.azurenative.customerinsights.outputs.GetLinkResult;
import com.pulumi.azurenative.customerinsights.outputs.GetPredictionModelStatusResult;
import com.pulumi.azurenative.customerinsights.outputs.GetPredictionResult;
import com.pulumi.azurenative.customerinsights.outputs.GetPredictionTrainingResultsResult;
import com.pulumi.azurenative.customerinsights.outputs.GetProfileResult;
import com.pulumi.azurenative.customerinsights.outputs.GetRelationshipLinkResult;
import com.pulumi.azurenative.customerinsights.outputs.GetRelationshipResult;
import com.pulumi.azurenative.customerinsights.outputs.GetRoleAssignmentResult;
import com.pulumi.azurenative.customerinsights.outputs.GetViewResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class CustomerinsightsFunctions {
/**
* Gets a connector in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getConnector(GetConnectorArgs args) {
return getConnector(args, InvokeOptions.Empty);
}
/**
* Gets a connector in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getConnectorPlain(GetConnectorPlainArgs args) {
return getConnectorPlain(args, InvokeOptions.Empty);
}
/**
* Gets a connector in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getConnector(GetConnectorArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getConnector", TypeShape.of(GetConnectorResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a connector in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getConnectorPlain(GetConnectorPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getConnector", TypeShape.of(GetConnectorResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a connector mapping in the connector.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getConnectorMapping(GetConnectorMappingArgs args) {
return getConnectorMapping(args, InvokeOptions.Empty);
}
/**
* Gets a connector mapping in the connector.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getConnectorMappingPlain(GetConnectorMappingPlainArgs args) {
return getConnectorMappingPlain(args, InvokeOptions.Empty);
}
/**
* Gets a connector mapping in the connector.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getConnectorMapping(GetConnectorMappingArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getConnectorMapping", TypeShape.of(GetConnectorMappingResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a connector mapping in the connector.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getConnectorMappingPlain(GetConnectorMappingPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getConnectorMapping", TypeShape.of(GetConnectorMappingResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getHub(GetHubArgs args) {
return getHub(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getHubPlain(GetHubPlainArgs args) {
return getHubPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getHub(GetHubArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getHub", TypeShape.of(GetHubResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getHubPlain(GetHubPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getHub", TypeShape.of(GetHubResult.class), args, Utilities.withVersion(options));
}
/**
* Gets data image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getImageUploadUrlForData(GetImageUploadUrlForDataArgs args) {
return getImageUploadUrlForData(args, InvokeOptions.Empty);
}
/**
* Gets data image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getImageUploadUrlForDataPlain(GetImageUploadUrlForDataPlainArgs args) {
return getImageUploadUrlForDataPlain(args, InvokeOptions.Empty);
}
/**
* Gets data image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getImageUploadUrlForData(GetImageUploadUrlForDataArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getImageUploadUrlForData", TypeShape.of(GetImageUploadUrlForDataResult.class), args, Utilities.withVersion(options));
}
/**
* Gets data image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getImageUploadUrlForDataPlain(GetImageUploadUrlForDataPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getImageUploadUrlForData", TypeShape.of(GetImageUploadUrlForDataResult.class), args, Utilities.withVersion(options));
}
/**
* Gets entity type (profile or interaction) image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getImageUploadUrlForEntityType(GetImageUploadUrlForEntityTypeArgs args) {
return getImageUploadUrlForEntityType(args, InvokeOptions.Empty);
}
/**
* Gets entity type (profile or interaction) image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getImageUploadUrlForEntityTypePlain(GetImageUploadUrlForEntityTypePlainArgs args) {
return getImageUploadUrlForEntityTypePlain(args, InvokeOptions.Empty);
}
/**
* Gets entity type (profile or interaction) image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getImageUploadUrlForEntityType(GetImageUploadUrlForEntityTypeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getImageUploadUrlForEntityType", TypeShape.of(GetImageUploadUrlForEntityTypeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets entity type (profile or interaction) image upload URL.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getImageUploadUrlForEntityTypePlain(GetImageUploadUrlForEntityTypePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getImageUploadUrlForEntityType", TypeShape.of(GetImageUploadUrlForEntityTypeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a KPI in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getKpi(GetKpiArgs args) {
return getKpi(args, InvokeOptions.Empty);
}
/**
* Gets a KPI in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getKpiPlain(GetKpiPlainArgs args) {
return getKpiPlain(args, InvokeOptions.Empty);
}
/**
* Gets a KPI in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getKpi(GetKpiArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getKpi", TypeShape.of(GetKpiResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a KPI in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getKpiPlain(GetKpiPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getKpi", TypeShape.of(GetKpiResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a link in the hub.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static Output getLink(GetLinkArgs args) {
return getLink(args, InvokeOptions.Empty);
}
/**
* Gets a link in the hub.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static CompletableFuture getLinkPlain(GetLinkPlainArgs args) {
return getLinkPlain(args, InvokeOptions.Empty);
}
/**
* Gets a link in the hub.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static Output getLink(GetLinkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getLink", TypeShape.of(GetLinkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a link in the hub.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static CompletableFuture getLinkPlain(GetLinkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getLink", TypeShape.of(GetLinkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Prediction in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getPrediction(GetPredictionArgs args) {
return getPrediction(args, InvokeOptions.Empty);
}
/**
* Gets a Prediction in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getPredictionPlain(GetPredictionPlainArgs args) {
return getPredictionPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Prediction in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getPrediction(GetPredictionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getPrediction", TypeShape.of(GetPredictionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Prediction in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getPredictionPlain(GetPredictionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getPrediction", TypeShape.of(GetPredictionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets model status of the prediction.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getPredictionModelStatus(GetPredictionModelStatusArgs args) {
return getPredictionModelStatus(args, InvokeOptions.Empty);
}
/**
* Gets model status of the prediction.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getPredictionModelStatusPlain(GetPredictionModelStatusPlainArgs args) {
return getPredictionModelStatusPlain(args, InvokeOptions.Empty);
}
/**
* Gets model status of the prediction.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getPredictionModelStatus(GetPredictionModelStatusArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getPredictionModelStatus", TypeShape.of(GetPredictionModelStatusResult.class), args, Utilities.withVersion(options));
}
/**
* Gets model status of the prediction.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getPredictionModelStatusPlain(GetPredictionModelStatusPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getPredictionModelStatus", TypeShape.of(GetPredictionModelStatusResult.class), args, Utilities.withVersion(options));
}
/**
* Gets training results.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getPredictionTrainingResults(GetPredictionTrainingResultsArgs args) {
return getPredictionTrainingResults(args, InvokeOptions.Empty);
}
/**
* Gets training results.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getPredictionTrainingResultsPlain(GetPredictionTrainingResultsPlainArgs args) {
return getPredictionTrainingResultsPlain(args, InvokeOptions.Empty);
}
/**
* Gets training results.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getPredictionTrainingResults(GetPredictionTrainingResultsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getPredictionTrainingResults", TypeShape.of(GetPredictionTrainingResultsResult.class), args, Utilities.withVersion(options));
}
/**
* Gets training results.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getPredictionTrainingResultsPlain(GetPredictionTrainingResultsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getPredictionTrainingResults", TypeShape.of(GetPredictionTrainingResultsResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified profile.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getProfile(GetProfileArgs args) {
return getProfile(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified profile.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getProfilePlain(GetProfilePlainArgs args) {
return getProfilePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified profile.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getProfile(GetProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getProfile", TypeShape.of(GetProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified profile.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getProfilePlain(GetProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getProfile", TypeShape.of(GetProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified relationship.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getRelationship(GetRelationshipArgs args) {
return getRelationship(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified relationship.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getRelationshipPlain(GetRelationshipPlainArgs args) {
return getRelationshipPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified relationship.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getRelationship(GetRelationshipArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getRelationship", TypeShape.of(GetRelationshipResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified relationship.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getRelationshipPlain(GetRelationshipPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getRelationship", TypeShape.of(GetRelationshipResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified relationship Link.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static Output getRelationshipLink(GetRelationshipLinkArgs args) {
return getRelationshipLink(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified relationship Link.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static CompletableFuture getRelationshipLinkPlain(GetRelationshipLinkPlainArgs args) {
return getRelationshipLinkPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified relationship Link.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static Output getRelationshipLink(GetRelationshipLinkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getRelationshipLink", TypeShape.of(GetRelationshipLinkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified relationship Link.
* Azure REST API version: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
*/
public static CompletableFuture getRelationshipLinkPlain(GetRelationshipLinkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getRelationshipLink", TypeShape.of(GetRelationshipLinkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the role assignment in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getRoleAssignment(GetRoleAssignmentArgs args) {
return getRoleAssignment(args, InvokeOptions.Empty);
}
/**
* Gets the role assignment in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getRoleAssignmentPlain(GetRoleAssignmentPlainArgs args) {
return getRoleAssignmentPlain(args, InvokeOptions.Empty);
}
/**
* Gets the role assignment in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getRoleAssignment(GetRoleAssignmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getRoleAssignment", TypeShape.of(GetRoleAssignmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the role assignment in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getRoleAssignmentPlain(GetRoleAssignmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getRoleAssignment", TypeShape.of(GetRoleAssignmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a view in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getView(GetViewArgs args) {
return getView(args, InvokeOptions.Empty);
}
/**
* Gets a view in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getViewPlain(GetViewPlainArgs args) {
return getViewPlain(args, InvokeOptions.Empty);
}
/**
* Gets a view in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static Output getView(GetViewArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:customerinsights:getView", TypeShape.of(GetViewResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a view in the hub.
* Azure REST API version: 2017-04-26.
*
*/
public static CompletableFuture getViewPlain(GetViewPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:customerinsights:getView", TypeShape.of(GetViewResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy