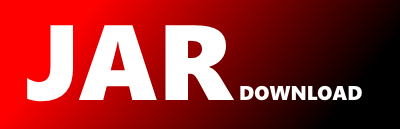
com.pulumi.azurenative.customerinsights.outputs.GetConnectorMappingResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights.outputs;
import com.pulumi.azurenative.customerinsights.outputs.ConnectorMappingPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetConnectorMappingResult {
/**
* @return The connector mapping name
*
*/
private String connectorMappingName;
/**
* @return The connector name.
*
*/
private String connectorName;
/**
* @return Type of connector.
*
*/
private @Nullable String connectorType;
/**
* @return The created time.
*
*/
private String created;
/**
* @return The DataFormat ID.
*
*/
private String dataFormatId;
/**
* @return The description of the connector mapping.
*
*/
private @Nullable String description;
/**
* @return Display name for the connector mapping.
*
*/
private @Nullable String displayName;
/**
* @return Defines which entity type the file should map to.
*
*/
private String entityType;
/**
* @return The mapping entity name.
*
*/
private String entityTypeName;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return The last modified time.
*
*/
private String lastModified;
/**
* @return The properties of the mapping.
*
*/
private ConnectorMappingPropertiesResponse mappingProperties;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The next run time based on customer's settings.
*
*/
private String nextRunTime;
/**
* @return The RunId.
*
*/
private String runId;
/**
* @return State of connector mapping.
*
*/
private String state;
/**
* @return The hub name.
*
*/
private String tenantId;
/**
* @return Resource type.
*
*/
private String type;
private GetConnectorMappingResult() {}
/**
* @return The connector mapping name
*
*/
public String connectorMappingName() {
return this.connectorMappingName;
}
/**
* @return The connector name.
*
*/
public String connectorName() {
return this.connectorName;
}
/**
* @return Type of connector.
*
*/
public Optional connectorType() {
return Optional.ofNullable(this.connectorType);
}
/**
* @return The created time.
*
*/
public String created() {
return this.created;
}
/**
* @return The DataFormat ID.
*
*/
public String dataFormatId() {
return this.dataFormatId;
}
/**
* @return The description of the connector mapping.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Display name for the connector mapping.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return Defines which entity type the file should map to.
*
*/
public String entityType() {
return this.entityType;
}
/**
* @return The mapping entity name.
*
*/
public String entityTypeName() {
return this.entityTypeName;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The last modified time.
*
*/
public String lastModified() {
return this.lastModified;
}
/**
* @return The properties of the mapping.
*
*/
public ConnectorMappingPropertiesResponse mappingProperties() {
return this.mappingProperties;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The next run time based on customer's settings.
*
*/
public String nextRunTime() {
return this.nextRunTime;
}
/**
* @return The RunId.
*
*/
public String runId() {
return this.runId;
}
/**
* @return State of connector mapping.
*
*/
public String state() {
return this.state;
}
/**
* @return The hub name.
*
*/
public String tenantId() {
return this.tenantId;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetConnectorMappingResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String connectorMappingName;
private String connectorName;
private @Nullable String connectorType;
private String created;
private String dataFormatId;
private @Nullable String description;
private @Nullable String displayName;
private String entityType;
private String entityTypeName;
private String id;
private String lastModified;
private ConnectorMappingPropertiesResponse mappingProperties;
private String name;
private String nextRunTime;
private String runId;
private String state;
private String tenantId;
private String type;
public Builder() {}
public Builder(GetConnectorMappingResult defaults) {
Objects.requireNonNull(defaults);
this.connectorMappingName = defaults.connectorMappingName;
this.connectorName = defaults.connectorName;
this.connectorType = defaults.connectorType;
this.created = defaults.created;
this.dataFormatId = defaults.dataFormatId;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.entityType = defaults.entityType;
this.entityTypeName = defaults.entityTypeName;
this.id = defaults.id;
this.lastModified = defaults.lastModified;
this.mappingProperties = defaults.mappingProperties;
this.name = defaults.name;
this.nextRunTime = defaults.nextRunTime;
this.runId = defaults.runId;
this.state = defaults.state;
this.tenantId = defaults.tenantId;
this.type = defaults.type;
}
@CustomType.Setter
public Builder connectorMappingName(String connectorMappingName) {
if (connectorMappingName == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "connectorMappingName");
}
this.connectorMappingName = connectorMappingName;
return this;
}
@CustomType.Setter
public Builder connectorName(String connectorName) {
if (connectorName == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "connectorName");
}
this.connectorName = connectorName;
return this;
}
@CustomType.Setter
public Builder connectorType(@Nullable String connectorType) {
this.connectorType = connectorType;
return this;
}
@CustomType.Setter
public Builder created(String created) {
if (created == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "created");
}
this.created = created;
return this;
}
@CustomType.Setter
public Builder dataFormatId(String dataFormatId) {
if (dataFormatId == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "dataFormatId");
}
this.dataFormatId = dataFormatId;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder entityType(String entityType) {
if (entityType == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "entityType");
}
this.entityType = entityType;
return this;
}
@CustomType.Setter
public Builder entityTypeName(String entityTypeName) {
if (entityTypeName == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "entityTypeName");
}
this.entityTypeName = entityTypeName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastModified(String lastModified) {
if (lastModified == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "lastModified");
}
this.lastModified = lastModified;
return this;
}
@CustomType.Setter
public Builder mappingProperties(ConnectorMappingPropertiesResponse mappingProperties) {
if (mappingProperties == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "mappingProperties");
}
this.mappingProperties = mappingProperties;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nextRunTime(String nextRunTime) {
if (nextRunTime == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "nextRunTime");
}
this.nextRunTime = nextRunTime;
return this;
}
@CustomType.Setter
public Builder runId(String runId) {
if (runId == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "runId");
}
this.runId = runId;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "tenantId");
}
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetConnectorMappingResult", "type");
}
this.type = type;
return this;
}
public GetConnectorMappingResult build() {
final var _resultValue = new GetConnectorMappingResult();
_resultValue.connectorMappingName = connectorMappingName;
_resultValue.connectorName = connectorName;
_resultValue.connectorType = connectorType;
_resultValue.created = created;
_resultValue.dataFormatId = dataFormatId;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.entityType = entityType;
_resultValue.entityTypeName = entityTypeName;
_resultValue.id = id;
_resultValue.lastModified = lastModified;
_resultValue.mappingProperties = mappingProperties;
_resultValue.name = name;
_resultValue.nextRunTime = nextRunTime;
_resultValue.runId = runId;
_resultValue.state = state;
_resultValue.tenantId = tenantId;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy