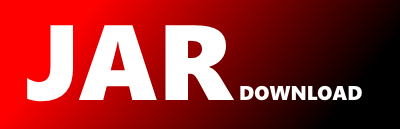
com.pulumi.azurenative.customerinsights.outputs.GetLinkResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights.outputs;
import com.pulumi.azurenative.customerinsights.outputs.ParticipantPropertyReferenceResponse;
import com.pulumi.azurenative.customerinsights.outputs.TypePropertiesMappingResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLinkResult {
/**
* @return Localized descriptions for the Link.
*
*/
private @Nullable Map description;
/**
* @return Localized display name for the Link.
*
*/
private @Nullable Map displayName;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return The link name.
*
*/
private String linkName;
/**
* @return The set of properties mappings between the source and target Types.
*
*/
private @Nullable List mappings;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Determines whether this link is supposed to create or delete instances if Link is NOT Reference Only.
*
*/
private @Nullable String operationType;
/**
* @return The properties that represent the participating profile.
*
*/
private List participantPropertyReferences;
/**
* @return Provisioning state.
*
*/
private String provisioningState;
/**
* @return Indicating whether the link is reference only link. This flag is ignored if the Mappings are defined. If the mappings are not defined and it is set to true, links processing will not create or update profiles.
*
*/
private @Nullable Boolean referenceOnly;
/**
* @return Type of source entity.
*
*/
private String sourceEntityType;
/**
* @return Name of the source Entity Type.
*
*/
private String sourceEntityTypeName;
/**
* @return Type of target entity.
*
*/
private String targetEntityType;
/**
* @return Name of the target Entity Type.
*
*/
private String targetEntityTypeName;
/**
* @return The hub name.
*
*/
private String tenantId;
/**
* @return Resource type.
*
*/
private String type;
private GetLinkResult() {}
/**
* @return Localized descriptions for the Link.
*
*/
public Map description() {
return this.description == null ? Map.of() : this.description;
}
/**
* @return Localized display name for the Link.
*
*/
public Map displayName() {
return this.displayName == null ? Map.of() : this.displayName;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The link name.
*
*/
public String linkName() {
return this.linkName;
}
/**
* @return The set of properties mappings between the source and target Types.
*
*/
public List mappings() {
return this.mappings == null ? List.of() : this.mappings;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Determines whether this link is supposed to create or delete instances if Link is NOT Reference Only.
*
*/
public Optional operationType() {
return Optional.ofNullable(this.operationType);
}
/**
* @return The properties that represent the participating profile.
*
*/
public List participantPropertyReferences() {
return this.participantPropertyReferences;
}
/**
* @return Provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Indicating whether the link is reference only link. This flag is ignored if the Mappings are defined. If the mappings are not defined and it is set to true, links processing will not create or update profiles.
*
*/
public Optional referenceOnly() {
return Optional.ofNullable(this.referenceOnly);
}
/**
* @return Type of source entity.
*
*/
public String sourceEntityType() {
return this.sourceEntityType;
}
/**
* @return Name of the source Entity Type.
*
*/
public String sourceEntityTypeName() {
return this.sourceEntityTypeName;
}
/**
* @return Type of target entity.
*
*/
public String targetEntityType() {
return this.targetEntityType;
}
/**
* @return Name of the target Entity Type.
*
*/
public String targetEntityTypeName() {
return this.targetEntityTypeName;
}
/**
* @return The hub name.
*
*/
public String tenantId() {
return this.tenantId;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLinkResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Map description;
private @Nullable Map displayName;
private String id;
private String linkName;
private @Nullable List mappings;
private String name;
private @Nullable String operationType;
private List participantPropertyReferences;
private String provisioningState;
private @Nullable Boolean referenceOnly;
private String sourceEntityType;
private String sourceEntityTypeName;
private String targetEntityType;
private String targetEntityTypeName;
private String tenantId;
private String type;
public Builder() {}
public Builder(GetLinkResult defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.displayName = defaults.displayName;
this.id = defaults.id;
this.linkName = defaults.linkName;
this.mappings = defaults.mappings;
this.name = defaults.name;
this.operationType = defaults.operationType;
this.participantPropertyReferences = defaults.participantPropertyReferences;
this.provisioningState = defaults.provisioningState;
this.referenceOnly = defaults.referenceOnly;
this.sourceEntityType = defaults.sourceEntityType;
this.sourceEntityTypeName = defaults.sourceEntityTypeName;
this.targetEntityType = defaults.targetEntityType;
this.targetEntityTypeName = defaults.targetEntityTypeName;
this.tenantId = defaults.tenantId;
this.type = defaults.type;
}
@CustomType.Setter
public Builder description(@Nullable Map description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable Map displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder linkName(String linkName) {
if (linkName == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "linkName");
}
this.linkName = linkName;
return this;
}
@CustomType.Setter
public Builder mappings(@Nullable List mappings) {
this.mappings = mappings;
return this;
}
public Builder mappings(TypePropertiesMappingResponse... mappings) {
return mappings(List.of(mappings));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder operationType(@Nullable String operationType) {
this.operationType = operationType;
return this;
}
@CustomType.Setter
public Builder participantPropertyReferences(List participantPropertyReferences) {
if (participantPropertyReferences == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "participantPropertyReferences");
}
this.participantPropertyReferences = participantPropertyReferences;
return this;
}
public Builder participantPropertyReferences(ParticipantPropertyReferenceResponse... participantPropertyReferences) {
return participantPropertyReferences(List.of(participantPropertyReferences));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder referenceOnly(@Nullable Boolean referenceOnly) {
this.referenceOnly = referenceOnly;
return this;
}
@CustomType.Setter
public Builder sourceEntityType(String sourceEntityType) {
if (sourceEntityType == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "sourceEntityType");
}
this.sourceEntityType = sourceEntityType;
return this;
}
@CustomType.Setter
public Builder sourceEntityTypeName(String sourceEntityTypeName) {
if (sourceEntityTypeName == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "sourceEntityTypeName");
}
this.sourceEntityTypeName = sourceEntityTypeName;
return this;
}
@CustomType.Setter
public Builder targetEntityType(String targetEntityType) {
if (targetEntityType == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "targetEntityType");
}
this.targetEntityType = targetEntityType;
return this;
}
@CustomType.Setter
public Builder targetEntityTypeName(String targetEntityTypeName) {
if (targetEntityTypeName == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "targetEntityTypeName");
}
this.targetEntityTypeName = targetEntityTypeName;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "tenantId");
}
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLinkResult", "type");
}
this.type = type;
return this;
}
public GetLinkResult build() {
final var _resultValue = new GetLinkResult();
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.id = id;
_resultValue.linkName = linkName;
_resultValue.mappings = mappings;
_resultValue.name = name;
_resultValue.operationType = operationType;
_resultValue.participantPropertyReferences = participantPropertyReferences;
_resultValue.provisioningState = provisioningState;
_resultValue.referenceOnly = referenceOnly;
_resultValue.sourceEntityType = sourceEntityType;
_resultValue.sourceEntityTypeName = sourceEntityTypeName;
_resultValue.targetEntityType = targetEntityType;
_resultValue.targetEntityTypeName = targetEntityTypeName;
_resultValue.tenantId = tenantId;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy