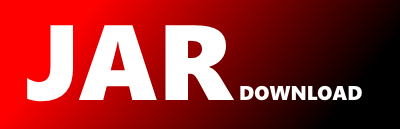
com.pulumi.azurenative.databox.inputs.ContactDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databox.inputs;
import com.pulumi.azurenative.databox.inputs.NotificationPreferenceArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Contact Details.
*
*/
public final class ContactDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final ContactDetailsArgs Empty = new ContactDetailsArgs();
/**
* Contact name of the person.
*
*/
@Import(name="contactName", required=true)
private Output contactName;
/**
* @return Contact name of the person.
*
*/
public Output contactName() {
return this.contactName;
}
/**
* List of Email-ids to be notified about job progress.
*
*/
@Import(name="emailList", required=true)
private Output> emailList;
/**
* @return List of Email-ids to be notified about job progress.
*
*/
public Output> emailList() {
return this.emailList;
}
/**
* Mobile number of the contact person.
*
*/
@Import(name="mobile")
private @Nullable Output mobile;
/**
* @return Mobile number of the contact person.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy