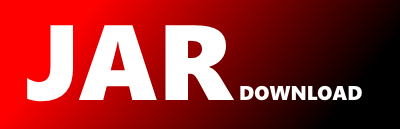
com.pulumi.azurenative.databox.inputs.DataBoxJobDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databox.inputs;
import com.pulumi.azurenative.databox.inputs.ContactDetailsArgs;
import com.pulumi.azurenative.databox.inputs.DataExportDetailsArgs;
import com.pulumi.azurenative.databox.inputs.DataImportDetailsArgs;
import com.pulumi.azurenative.databox.inputs.KeyEncryptionKeyArgs;
import com.pulumi.azurenative.databox.inputs.PreferencesArgs;
import com.pulumi.azurenative.databox.inputs.ReverseShippingDetailsArgs;
import com.pulumi.azurenative.databox.inputs.ShippingAddressArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Databox Job Details
*
*/
public final class DataBoxJobDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final DataBoxJobDetailsArgs Empty = new DataBoxJobDetailsArgs();
/**
* Contact details for notification and shipping.
*
*/
@Import(name="contactDetails", required=true)
private Output contactDetails;
/**
* @return Contact details for notification and shipping.
*
*/
public Output contactDetails() {
return this.contactDetails;
}
/**
* Details of the data to be exported from azure.
*
*/
@Import(name="dataExportDetails")
private @Nullable Output> dataExportDetails;
/**
* @return Details of the data to be exported from azure.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy