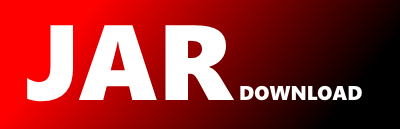
com.pulumi.azurenative.databox.outputs.DataBoxJobDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databox.outputs;
import com.pulumi.azurenative.databox.outputs.ContactDetailsResponse;
import com.pulumi.azurenative.databox.outputs.CopyProgressResponse;
import com.pulumi.azurenative.databox.outputs.DataBoxAccountCopyLogDetailsResponse;
import com.pulumi.azurenative.databox.outputs.DataBoxCustomerDiskCopyLogDetailsResponse;
import com.pulumi.azurenative.databox.outputs.DataBoxDiskCopyLogDetailsResponse;
import com.pulumi.azurenative.databox.outputs.DataBoxHeavyAccountCopyLogDetailsResponse;
import com.pulumi.azurenative.databox.outputs.DataExportDetailsResponse;
import com.pulumi.azurenative.databox.outputs.DataImportDetailsResponse;
import com.pulumi.azurenative.databox.outputs.DatacenterAddressInstructionResponseResponse;
import com.pulumi.azurenative.databox.outputs.DatacenterAddressLocationResponseResponse;
import com.pulumi.azurenative.databox.outputs.DeviceErasureDetailsResponse;
import com.pulumi.azurenative.databox.outputs.JobStagesResponse;
import com.pulumi.azurenative.databox.outputs.KeyEncryptionKeyResponse;
import com.pulumi.azurenative.databox.outputs.LastMitigationActionOnJobResponse;
import com.pulumi.azurenative.databox.outputs.PackageShippingDetailsResponse;
import com.pulumi.azurenative.databox.outputs.PreferencesResponse;
import com.pulumi.azurenative.databox.outputs.ReverseShippingDetailsResponse;
import com.pulumi.azurenative.databox.outputs.ShippingAddressResponse;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DataBoxJobDetailsResponse {
/**
* @return Available actions on the job.
*
*/
private List actions;
/**
* @return Shared access key to download the chain of custody logs
*
*/
private String chainOfCustodySasKey;
/**
* @return Contact details for notification and shipping.
*
*/
private ContactDetailsResponse contactDetails;
/**
* @return List of copy log details.
*
*/
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy