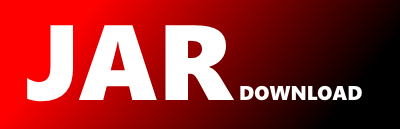
com.pulumi.azurenative.databoxedge.outputs.GetShareResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databoxedge.outputs;
import com.pulumi.azurenative.databoxedge.outputs.AzureContainerInfoResponse;
import com.pulumi.azurenative.databoxedge.outputs.ClientAccessRightResponse;
import com.pulumi.azurenative.databoxedge.outputs.MountPointMapResponse;
import com.pulumi.azurenative.databoxedge.outputs.RefreshDetailsResponse;
import com.pulumi.azurenative.databoxedge.outputs.SystemDataResponse;
import com.pulumi.azurenative.databoxedge.outputs.UserAccessRightResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetShareResult {
/**
* @return Access protocol to be used by the share.
*
*/
private String accessProtocol;
/**
* @return Azure container mapping for the share.
*
*/
private @Nullable AzureContainerInfoResponse azureContainerInfo;
/**
* @return List of IP addresses and corresponding access rights on the share(required for NFS protocol).
*
*/
private @Nullable List clientAccessRights;
/**
* @return Data policy of the share.
*
*/
private @Nullable String dataPolicy;
/**
* @return Description for the share.
*
*/
private @Nullable String description;
/**
* @return The path ID that uniquely identifies the object.
*
*/
private String id;
/**
* @return Current monitoring status of the share.
*
*/
private String monitoringStatus;
/**
* @return The object name.
*
*/
private String name;
/**
* @return Details of the refresh job on this share.
*
*/
private @Nullable RefreshDetailsResponse refreshDetails;
/**
* @return Share mount point to the role.
*
*/
private List shareMappings;
/**
* @return Current status of the share.
*
*/
private String shareStatus;
/**
* @return Metadata pertaining to creation and last modification of Share
*
*/
private SystemDataResponse systemData;
/**
* @return The hierarchical type of the object.
*
*/
private String type;
/**
* @return Mapping of users and corresponding access rights on the share (required for SMB protocol).
*
*/
private @Nullable List userAccessRights;
private GetShareResult() {}
/**
* @return Access protocol to be used by the share.
*
*/
public String accessProtocol() {
return this.accessProtocol;
}
/**
* @return Azure container mapping for the share.
*
*/
public Optional azureContainerInfo() {
return Optional.ofNullable(this.azureContainerInfo);
}
/**
* @return List of IP addresses and corresponding access rights on the share(required for NFS protocol).
*
*/
public List clientAccessRights() {
return this.clientAccessRights == null ? List.of() : this.clientAccessRights;
}
/**
* @return Data policy of the share.
*
*/
public Optional dataPolicy() {
return Optional.ofNullable(this.dataPolicy);
}
/**
* @return Description for the share.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The path ID that uniquely identifies the object.
*
*/
public String id() {
return this.id;
}
/**
* @return Current monitoring status of the share.
*
*/
public String monitoringStatus() {
return this.monitoringStatus;
}
/**
* @return The object name.
*
*/
public String name() {
return this.name;
}
/**
* @return Details of the refresh job on this share.
*
*/
public Optional refreshDetails() {
return Optional.ofNullable(this.refreshDetails);
}
/**
* @return Share mount point to the role.
*
*/
public List shareMappings() {
return this.shareMappings;
}
/**
* @return Current status of the share.
*
*/
public String shareStatus() {
return this.shareStatus;
}
/**
* @return Metadata pertaining to creation and last modification of Share
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The hierarchical type of the object.
*
*/
public String type() {
return this.type;
}
/**
* @return Mapping of users and corresponding access rights on the share (required for SMB protocol).
*
*/
public List userAccessRights() {
return this.userAccessRights == null ? List.of() : this.userAccessRights;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetShareResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessProtocol;
private @Nullable AzureContainerInfoResponse azureContainerInfo;
private @Nullable List clientAccessRights;
private @Nullable String dataPolicy;
private @Nullable String description;
private String id;
private String monitoringStatus;
private String name;
private @Nullable RefreshDetailsResponse refreshDetails;
private List shareMappings;
private String shareStatus;
private SystemDataResponse systemData;
private String type;
private @Nullable List userAccessRights;
public Builder() {}
public Builder(GetShareResult defaults) {
Objects.requireNonNull(defaults);
this.accessProtocol = defaults.accessProtocol;
this.azureContainerInfo = defaults.azureContainerInfo;
this.clientAccessRights = defaults.clientAccessRights;
this.dataPolicy = defaults.dataPolicy;
this.description = defaults.description;
this.id = defaults.id;
this.monitoringStatus = defaults.monitoringStatus;
this.name = defaults.name;
this.refreshDetails = defaults.refreshDetails;
this.shareMappings = defaults.shareMappings;
this.shareStatus = defaults.shareStatus;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.userAccessRights = defaults.userAccessRights;
}
@CustomType.Setter
public Builder accessProtocol(String accessProtocol) {
if (accessProtocol == null) {
throw new MissingRequiredPropertyException("GetShareResult", "accessProtocol");
}
this.accessProtocol = accessProtocol;
return this;
}
@CustomType.Setter
public Builder azureContainerInfo(@Nullable AzureContainerInfoResponse azureContainerInfo) {
this.azureContainerInfo = azureContainerInfo;
return this;
}
@CustomType.Setter
public Builder clientAccessRights(@Nullable List clientAccessRights) {
this.clientAccessRights = clientAccessRights;
return this;
}
public Builder clientAccessRights(ClientAccessRightResponse... clientAccessRights) {
return clientAccessRights(List.of(clientAccessRights));
}
@CustomType.Setter
public Builder dataPolicy(@Nullable String dataPolicy) {
this.dataPolicy = dataPolicy;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetShareResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder monitoringStatus(String monitoringStatus) {
if (monitoringStatus == null) {
throw new MissingRequiredPropertyException("GetShareResult", "monitoringStatus");
}
this.monitoringStatus = monitoringStatus;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetShareResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder refreshDetails(@Nullable RefreshDetailsResponse refreshDetails) {
this.refreshDetails = refreshDetails;
return this;
}
@CustomType.Setter
public Builder shareMappings(List shareMappings) {
if (shareMappings == null) {
throw new MissingRequiredPropertyException("GetShareResult", "shareMappings");
}
this.shareMappings = shareMappings;
return this;
}
public Builder shareMappings(MountPointMapResponse... shareMappings) {
return shareMappings(List.of(shareMappings));
}
@CustomType.Setter
public Builder shareStatus(String shareStatus) {
if (shareStatus == null) {
throw new MissingRequiredPropertyException("GetShareResult", "shareStatus");
}
this.shareStatus = shareStatus;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetShareResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetShareResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder userAccessRights(@Nullable List userAccessRights) {
this.userAccessRights = userAccessRights;
return this;
}
public Builder userAccessRights(UserAccessRightResponse... userAccessRights) {
return userAccessRights(List.of(userAccessRights));
}
public GetShareResult build() {
final var _resultValue = new GetShareResult();
_resultValue.accessProtocol = accessProtocol;
_resultValue.azureContainerInfo = azureContainerInfo;
_resultValue.clientAccessRights = clientAccessRights;
_resultValue.dataPolicy = dataPolicy;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.monitoringStatus = monitoringStatus;
_resultValue.name = name;
_resultValue.refreshDetails = refreshDetails;
_resultValue.shareMappings = shareMappings;
_resultValue.shareStatus = shareStatus;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.userAccessRights = userAccessRights;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy