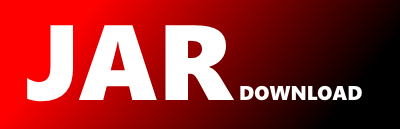
com.pulumi.azurenative.datafactory.outputs.DelimitedTextDatasetResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.azurenative.datafactory.outputs.AmazonS3CompatibleLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.AmazonS3LocationResponse;
import com.pulumi.azurenative.datafactory.outputs.AzureBlobFSLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.AzureBlobStorageLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.AzureDataLakeStoreLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.AzureFileStorageLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.DatasetResponseFolder;
import com.pulumi.azurenative.datafactory.outputs.FileServerLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.FtpServerLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.GoogleCloudStorageLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.HdfsLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.HttpServerLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.LakeHouseLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.LinkedServiceReferenceResponse;
import com.pulumi.azurenative.datafactory.outputs.OracleCloudStorageLocationResponse;
import com.pulumi.azurenative.datafactory.outputs.ParameterSpecificationResponse;
import com.pulumi.azurenative.datafactory.outputs.SftpLocationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DelimitedTextDatasetResponse {
/**
* @return List of tags that can be used for describing the Dataset.
*
*/
private @Nullable List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy