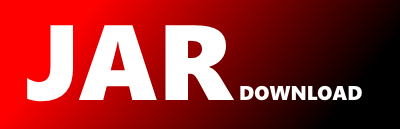
com.pulumi.azurenative.datafactory.outputs.GetChangeDataCaptureResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.azurenative.datafactory.outputs.ChangeDataCaptureResponseFolder;
import com.pulumi.azurenative.datafactory.outputs.MapperPolicyResponse;
import com.pulumi.azurenative.datafactory.outputs.MapperSourceConnectionsInfoResponse;
import com.pulumi.azurenative.datafactory.outputs.MapperTargetConnectionsInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetChangeDataCaptureResult {
/**
* @return A boolean to determine if the vnet configuration needs to be overwritten.
*
*/
private @Nullable Boolean allowVNetOverride;
/**
* @return The description of the change data capture.
*
*/
private @Nullable String description;
/**
* @return Etag identifies change in the resource.
*
*/
private String etag;
/**
* @return The folder that this CDC is in. If not specified, CDC will appear at the root level.
*
*/
private @Nullable ChangeDataCaptureResponseFolder folder;
/**
* @return The resource identifier.
*
*/
private String id;
/**
* @return The resource name.
*
*/
private String name;
/**
* @return CDC policy
*
*/
private MapperPolicyResponse policy;
/**
* @return List of sources connections that can be used as sources in the CDC.
*
*/
private List sourceConnectionsInfo;
/**
* @return Status of the CDC as to if it is running or stopped.
*
*/
private @Nullable String status;
/**
* @return List of target connections that can be used as sources in the CDC.
*
*/
private List targetConnectionsInfo;
/**
* @return The resource type.
*
*/
private String type;
private GetChangeDataCaptureResult() {}
/**
* @return A boolean to determine if the vnet configuration needs to be overwritten.
*
*/
public Optional allowVNetOverride() {
return Optional.ofNullable(this.allowVNetOverride);
}
/**
* @return The description of the change data capture.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Etag identifies change in the resource.
*
*/
public String etag() {
return this.etag;
}
/**
* @return The folder that this CDC is in. If not specified, CDC will appear at the root level.
*
*/
public Optional folder() {
return Optional.ofNullable(this.folder);
}
/**
* @return The resource identifier.
*
*/
public String id() {
return this.id;
}
/**
* @return The resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return CDC policy
*
*/
public MapperPolicyResponse policy() {
return this.policy;
}
/**
* @return List of sources connections that can be used as sources in the CDC.
*
*/
public List sourceConnectionsInfo() {
return this.sourceConnectionsInfo;
}
/**
* @return Status of the CDC as to if it is running or stopped.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return List of target connections that can be used as sources in the CDC.
*
*/
public List targetConnectionsInfo() {
return this.targetConnectionsInfo;
}
/**
* @return The resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetChangeDataCaptureResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowVNetOverride;
private @Nullable String description;
private String etag;
private @Nullable ChangeDataCaptureResponseFolder folder;
private String id;
private String name;
private MapperPolicyResponse policy;
private List sourceConnectionsInfo;
private @Nullable String status;
private List targetConnectionsInfo;
private String type;
public Builder() {}
public Builder(GetChangeDataCaptureResult defaults) {
Objects.requireNonNull(defaults);
this.allowVNetOverride = defaults.allowVNetOverride;
this.description = defaults.description;
this.etag = defaults.etag;
this.folder = defaults.folder;
this.id = defaults.id;
this.name = defaults.name;
this.policy = defaults.policy;
this.sourceConnectionsInfo = defaults.sourceConnectionsInfo;
this.status = defaults.status;
this.targetConnectionsInfo = defaults.targetConnectionsInfo;
this.type = defaults.type;
}
@CustomType.Setter
public Builder allowVNetOverride(@Nullable Boolean allowVNetOverride) {
this.allowVNetOverride = allowVNetOverride;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder folder(@Nullable ChangeDataCaptureResponseFolder folder) {
this.folder = folder;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder policy(MapperPolicyResponse policy) {
if (policy == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "policy");
}
this.policy = policy;
return this;
}
@CustomType.Setter
public Builder sourceConnectionsInfo(List sourceConnectionsInfo) {
if (sourceConnectionsInfo == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "sourceConnectionsInfo");
}
this.sourceConnectionsInfo = sourceConnectionsInfo;
return this;
}
public Builder sourceConnectionsInfo(MapperSourceConnectionsInfoResponse... sourceConnectionsInfo) {
return sourceConnectionsInfo(List.of(sourceConnectionsInfo));
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder targetConnectionsInfo(List targetConnectionsInfo) {
if (targetConnectionsInfo == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "targetConnectionsInfo");
}
this.targetConnectionsInfo = targetConnectionsInfo;
return this;
}
public Builder targetConnectionsInfo(MapperTargetConnectionsInfoResponse... targetConnectionsInfo) {
return targetConnectionsInfo(List.of(targetConnectionsInfo));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetChangeDataCaptureResult", "type");
}
this.type = type;
return this;
}
public GetChangeDataCaptureResult build() {
final var _resultValue = new GetChangeDataCaptureResult();
_resultValue.allowVNetOverride = allowVNetOverride;
_resultValue.description = description;
_resultValue.etag = etag;
_resultValue.folder = folder;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.policy = policy;
_resultValue.sourceConnectionsInfo = sourceConnectionsInfo;
_resultValue.status = status;
_resultValue.targetConnectionsInfo = targetConnectionsInfo;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy