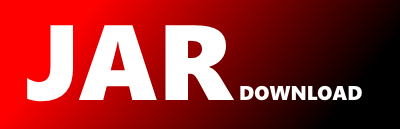
com.pulumi.azurenative.datafactory.outputs.SelfHostedIntegrationRuntimeStatusResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.azurenative.datafactory.outputs.LinkedIntegrationRuntimeResponse;
import com.pulumi.azurenative.datafactory.outputs.SelfHostedIntegrationRuntimeNodeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class SelfHostedIntegrationRuntimeStatusResponse {
/**
* @return Whether Self-hosted integration runtime auto update has been turned on.
*
*/
private String autoUpdate;
/**
* @return The estimated time when the self-hosted integration runtime will be updated.
*
*/
private String autoUpdateETA;
/**
* @return Object with additional information about integration runtime capabilities.
*
*/
private Map capabilities;
/**
* @return The time at which the integration runtime was created, in ISO8601 format.
*
*/
private String createTime;
/**
* @return The data factory name which the integration runtime belong to.
*
*/
private String dataFactoryName;
/**
* @return It is used to set the encryption mode for node-node communication channel (when more than 2 self-hosted integration runtime nodes exist).
*
*/
private String internalChannelEncryption;
/**
* @return The latest version on download center.
*
*/
private String latestVersion;
/**
* @return The list of linked integration runtimes that are created to share with this integration runtime.
*
*/
private @Nullable List links;
/**
* @return The local time zone offset in hours.
*
*/
private String localTimeZoneOffset;
/**
* @return The list of nodes for this integration runtime.
*
*/
private @Nullable List nodes;
/**
* @return The version that the integration runtime is going to update to.
*
*/
private String pushedVersion;
/**
* @return The date at which the integration runtime will be scheduled to update, in ISO8601 format.
*
*/
private String scheduledUpdateDate;
/**
* @return An alternative option to ensure interactive authoring function when your self-hosted integration runtime is unable to establish a connection with Azure Relay.
*
*/
private Boolean selfContainedInteractiveAuthoringEnabled;
/**
* @return The URLs for the services used in integration runtime backend service.
*
*/
private List serviceUrls;
/**
* @return The state of integration runtime.
*
*/
private String state;
/**
* @return The task queue id of the integration runtime.
*
*/
private String taskQueueId;
/**
* @return The type of integration runtime.
* Expected value is 'SelfHosted'.
*
*/
private String type;
/**
* @return The time in the date scheduled by service to update the integration runtime, e.g., PT03H is 3 hours
*
*/
private String updateDelayOffset;
/**
* @return Version of the integration runtime.
*
*/
private String version;
/**
* @return Status of the integration runtime version.
*
*/
private String versionStatus;
private SelfHostedIntegrationRuntimeStatusResponse() {}
/**
* @return Whether Self-hosted integration runtime auto update has been turned on.
*
*/
public String autoUpdate() {
return this.autoUpdate;
}
/**
* @return The estimated time when the self-hosted integration runtime will be updated.
*
*/
public String autoUpdateETA() {
return this.autoUpdateETA;
}
/**
* @return Object with additional information about integration runtime capabilities.
*
*/
public Map capabilities() {
return this.capabilities;
}
/**
* @return The time at which the integration runtime was created, in ISO8601 format.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return The data factory name which the integration runtime belong to.
*
*/
public String dataFactoryName() {
return this.dataFactoryName;
}
/**
* @return It is used to set the encryption mode for node-node communication channel (when more than 2 self-hosted integration runtime nodes exist).
*
*/
public String internalChannelEncryption() {
return this.internalChannelEncryption;
}
/**
* @return The latest version on download center.
*
*/
public String latestVersion() {
return this.latestVersion;
}
/**
* @return The list of linked integration runtimes that are created to share with this integration runtime.
*
*/
public List links() {
return this.links == null ? List.of() : this.links;
}
/**
* @return The local time zone offset in hours.
*
*/
public String localTimeZoneOffset() {
return this.localTimeZoneOffset;
}
/**
* @return The list of nodes for this integration runtime.
*
*/
public List nodes() {
return this.nodes == null ? List.of() : this.nodes;
}
/**
* @return The version that the integration runtime is going to update to.
*
*/
public String pushedVersion() {
return this.pushedVersion;
}
/**
* @return The date at which the integration runtime will be scheduled to update, in ISO8601 format.
*
*/
public String scheduledUpdateDate() {
return this.scheduledUpdateDate;
}
/**
* @return An alternative option to ensure interactive authoring function when your self-hosted integration runtime is unable to establish a connection with Azure Relay.
*
*/
public Boolean selfContainedInteractiveAuthoringEnabled() {
return this.selfContainedInteractiveAuthoringEnabled;
}
/**
* @return The URLs for the services used in integration runtime backend service.
*
*/
public List serviceUrls() {
return this.serviceUrls;
}
/**
* @return The state of integration runtime.
*
*/
public String state() {
return this.state;
}
/**
* @return The task queue id of the integration runtime.
*
*/
public String taskQueueId() {
return this.taskQueueId;
}
/**
* @return The type of integration runtime.
* Expected value is 'SelfHosted'.
*
*/
public String type() {
return this.type;
}
/**
* @return The time in the date scheduled by service to update the integration runtime, e.g., PT03H is 3 hours
*
*/
public String updateDelayOffset() {
return this.updateDelayOffset;
}
/**
* @return Version of the integration runtime.
*
*/
public String version() {
return this.version;
}
/**
* @return Status of the integration runtime version.
*
*/
public String versionStatus() {
return this.versionStatus;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SelfHostedIntegrationRuntimeStatusResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String autoUpdate;
private String autoUpdateETA;
private Map capabilities;
private String createTime;
private String dataFactoryName;
private String internalChannelEncryption;
private String latestVersion;
private @Nullable List links;
private String localTimeZoneOffset;
private @Nullable List nodes;
private String pushedVersion;
private String scheduledUpdateDate;
private Boolean selfContainedInteractiveAuthoringEnabled;
private List serviceUrls;
private String state;
private String taskQueueId;
private String type;
private String updateDelayOffset;
private String version;
private String versionStatus;
public Builder() {}
public Builder(SelfHostedIntegrationRuntimeStatusResponse defaults) {
Objects.requireNonNull(defaults);
this.autoUpdate = defaults.autoUpdate;
this.autoUpdateETA = defaults.autoUpdateETA;
this.capabilities = defaults.capabilities;
this.createTime = defaults.createTime;
this.dataFactoryName = defaults.dataFactoryName;
this.internalChannelEncryption = defaults.internalChannelEncryption;
this.latestVersion = defaults.latestVersion;
this.links = defaults.links;
this.localTimeZoneOffset = defaults.localTimeZoneOffset;
this.nodes = defaults.nodes;
this.pushedVersion = defaults.pushedVersion;
this.scheduledUpdateDate = defaults.scheduledUpdateDate;
this.selfContainedInteractiveAuthoringEnabled = defaults.selfContainedInteractiveAuthoringEnabled;
this.serviceUrls = defaults.serviceUrls;
this.state = defaults.state;
this.taskQueueId = defaults.taskQueueId;
this.type = defaults.type;
this.updateDelayOffset = defaults.updateDelayOffset;
this.version = defaults.version;
this.versionStatus = defaults.versionStatus;
}
@CustomType.Setter
public Builder autoUpdate(String autoUpdate) {
if (autoUpdate == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "autoUpdate");
}
this.autoUpdate = autoUpdate;
return this;
}
@CustomType.Setter
public Builder autoUpdateETA(String autoUpdateETA) {
if (autoUpdateETA == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "autoUpdateETA");
}
this.autoUpdateETA = autoUpdateETA;
return this;
}
@CustomType.Setter
public Builder capabilities(Map capabilities) {
if (capabilities == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "capabilities");
}
this.capabilities = capabilities;
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
if (createTime == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "createTime");
}
this.createTime = createTime;
return this;
}
@CustomType.Setter
public Builder dataFactoryName(String dataFactoryName) {
if (dataFactoryName == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "dataFactoryName");
}
this.dataFactoryName = dataFactoryName;
return this;
}
@CustomType.Setter
public Builder internalChannelEncryption(String internalChannelEncryption) {
if (internalChannelEncryption == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "internalChannelEncryption");
}
this.internalChannelEncryption = internalChannelEncryption;
return this;
}
@CustomType.Setter
public Builder latestVersion(String latestVersion) {
if (latestVersion == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "latestVersion");
}
this.latestVersion = latestVersion;
return this;
}
@CustomType.Setter
public Builder links(@Nullable List links) {
this.links = links;
return this;
}
public Builder links(LinkedIntegrationRuntimeResponse... links) {
return links(List.of(links));
}
@CustomType.Setter
public Builder localTimeZoneOffset(String localTimeZoneOffset) {
if (localTimeZoneOffset == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "localTimeZoneOffset");
}
this.localTimeZoneOffset = localTimeZoneOffset;
return this;
}
@CustomType.Setter
public Builder nodes(@Nullable List nodes) {
this.nodes = nodes;
return this;
}
public Builder nodes(SelfHostedIntegrationRuntimeNodeResponse... nodes) {
return nodes(List.of(nodes));
}
@CustomType.Setter
public Builder pushedVersion(String pushedVersion) {
if (pushedVersion == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "pushedVersion");
}
this.pushedVersion = pushedVersion;
return this;
}
@CustomType.Setter
public Builder scheduledUpdateDate(String scheduledUpdateDate) {
if (scheduledUpdateDate == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "scheduledUpdateDate");
}
this.scheduledUpdateDate = scheduledUpdateDate;
return this;
}
@CustomType.Setter
public Builder selfContainedInteractiveAuthoringEnabled(Boolean selfContainedInteractiveAuthoringEnabled) {
if (selfContainedInteractiveAuthoringEnabled == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "selfContainedInteractiveAuthoringEnabled");
}
this.selfContainedInteractiveAuthoringEnabled = selfContainedInteractiveAuthoringEnabled;
return this;
}
@CustomType.Setter
public Builder serviceUrls(List serviceUrls) {
if (serviceUrls == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "serviceUrls");
}
this.serviceUrls = serviceUrls;
return this;
}
public Builder serviceUrls(String... serviceUrls) {
return serviceUrls(List.of(serviceUrls));
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder taskQueueId(String taskQueueId) {
if (taskQueueId == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "taskQueueId");
}
this.taskQueueId = taskQueueId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder updateDelayOffset(String updateDelayOffset) {
if (updateDelayOffset == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "updateDelayOffset");
}
this.updateDelayOffset = updateDelayOffset;
return this;
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "version");
}
this.version = version;
return this;
}
@CustomType.Setter
public Builder versionStatus(String versionStatus) {
if (versionStatus == null) {
throw new MissingRequiredPropertyException("SelfHostedIntegrationRuntimeStatusResponse", "versionStatus");
}
this.versionStatus = versionStatus;
return this;
}
public SelfHostedIntegrationRuntimeStatusResponse build() {
final var _resultValue = new SelfHostedIntegrationRuntimeStatusResponse();
_resultValue.autoUpdate = autoUpdate;
_resultValue.autoUpdateETA = autoUpdateETA;
_resultValue.capabilities = capabilities;
_resultValue.createTime = createTime;
_resultValue.dataFactoryName = dataFactoryName;
_resultValue.internalChannelEncryption = internalChannelEncryption;
_resultValue.latestVersion = latestVersion;
_resultValue.links = links;
_resultValue.localTimeZoneOffset = localTimeZoneOffset;
_resultValue.nodes = nodes;
_resultValue.pushedVersion = pushedVersion;
_resultValue.scheduledUpdateDate = scheduledUpdateDate;
_resultValue.selfContainedInteractiveAuthoringEnabled = selfContainedInteractiveAuthoringEnabled;
_resultValue.serviceUrls = serviceUrls;
_resultValue.state = state;
_resultValue.taskQueueId = taskQueueId;
_resultValue.type = type;
_resultValue.updateDelayOffset = updateDelayOffset;
_resultValue.version = version;
_resultValue.versionStatus = versionStatus;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy