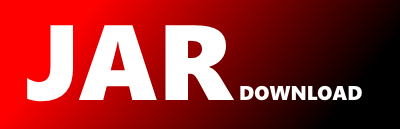
com.pulumi.azurenative.datafactory.outputs.TextFormatResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class TextFormatResponse {
/**
* @return The column delimiter. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object columnDelimiter;
/**
* @return Deserializer. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object deserializer;
/**
* @return The code page name of the preferred encoding. If miss, the default value is ΓÇ£utf-8ΓÇ¥, unless BOM denotes another Unicode encoding. Refer to the ΓÇ£NameΓÇ¥ column of the table in the following link to set supported values: https://msdn.microsoft.com/library/system.text.encoding.aspx. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object encodingName;
/**
* @return The escape character. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object escapeChar;
/**
* @return When used as input, treat the first row of data as headers. When used as output,write the headers into the output as the first row of data. The default value is false. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object firstRowAsHeader;
/**
* @return The null value string. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object nullValue;
/**
* @return The quote character. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object quoteChar;
/**
* @return The row delimiter. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object rowDelimiter;
/**
* @return Serializer. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object serializer;
/**
* @return The number of lines/rows to be skipped when parsing text files. The default value is 0. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object skipLineCount;
/**
* @return Treat empty column values in the text file as null. The default value is true. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object treatEmptyAsNull;
/**
* @return Type of dataset storage format.
* Expected value is 'TextFormat'.
*
*/
private String type;
private TextFormatResponse() {}
/**
* @return The column delimiter. Type: string (or Expression with resultType string).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy