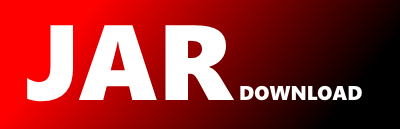
com.pulumi.azurenative.datalakestore.outputs.GetAccountResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datalakestore.outputs;
import com.pulumi.azurenative.datalakestore.outputs.EncryptionConfigResponse;
import com.pulumi.azurenative.datalakestore.outputs.EncryptionIdentityResponse;
import com.pulumi.azurenative.datalakestore.outputs.FirewallRuleResponse;
import com.pulumi.azurenative.datalakestore.outputs.TrustedIdProviderResponse;
import com.pulumi.azurenative.datalakestore.outputs.VirtualNetworkRuleResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetAccountResult {
/**
* @return The unique identifier associated with this Data Lake Store account.
*
*/
private String accountId;
/**
* @return The account creation time.
*
*/
private String creationTime;
/**
* @return The commitment tier in use for the current month.
*
*/
private String currentTier;
/**
* @return The default owner group for all new folders and files created in the Data Lake Store account.
*
*/
private String defaultGroup;
/**
* @return The Key Vault encryption configuration.
*
*/
private EncryptionConfigResponse encryptionConfig;
/**
* @return The current state of encryption provisioning for this Data Lake Store account.
*
*/
private String encryptionProvisioningState;
/**
* @return The current state of encryption for this Data Lake Store account.
*
*/
private String encryptionState;
/**
* @return The full CName endpoint for this account.
*
*/
private String endpoint;
/**
* @return The current state of allowing or disallowing IPs originating within Azure through the firewall. If the firewall is disabled, this is not enforced.
*
*/
private String firewallAllowAzureIps;
/**
* @return The list of firewall rules associated with this Data Lake Store account.
*
*/
private List firewallRules;
/**
* @return The current state of the IP address firewall for this Data Lake Store account.
*
*/
private String firewallState;
/**
* @return The resource identifier.
*
*/
private String id;
/**
* @return The Key Vault encryption identity, if any.
*
*/
private EncryptionIdentityResponse identity;
/**
* @return The account last modified time.
*
*/
private String lastModifiedTime;
/**
* @return The resource location.
*
*/
private String location;
/**
* @return The resource name.
*
*/
private String name;
/**
* @return The commitment tier to use for next month.
*
*/
private String newTier;
/**
* @return The provisioning status of the Data Lake Store account.
*
*/
private String provisioningState;
/**
* @return The state of the Data Lake Store account.
*
*/
private String state;
/**
* @return The resource tags.
*
*/
private Map tags;
/**
* @return The current state of the trusted identity provider feature for this Data Lake Store account.
*
*/
private String trustedIdProviderState;
/**
* @return The list of trusted identity providers associated with this Data Lake Store account.
*
*/
private List trustedIdProviders;
/**
* @return The resource type.
*
*/
private String type;
/**
* @return The list of virtual network rules associated with this Data Lake Store account.
*
*/
private List virtualNetworkRules;
private GetAccountResult() {}
/**
* @return The unique identifier associated with this Data Lake Store account.
*
*/
public String accountId() {
return this.accountId;
}
/**
* @return The account creation time.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return The commitment tier in use for the current month.
*
*/
public String currentTier() {
return this.currentTier;
}
/**
* @return The default owner group for all new folders and files created in the Data Lake Store account.
*
*/
public String defaultGroup() {
return this.defaultGroup;
}
/**
* @return The Key Vault encryption configuration.
*
*/
public EncryptionConfigResponse encryptionConfig() {
return this.encryptionConfig;
}
/**
* @return The current state of encryption provisioning for this Data Lake Store account.
*
*/
public String encryptionProvisioningState() {
return this.encryptionProvisioningState;
}
/**
* @return The current state of encryption for this Data Lake Store account.
*
*/
public String encryptionState() {
return this.encryptionState;
}
/**
* @return The full CName endpoint for this account.
*
*/
public String endpoint() {
return this.endpoint;
}
/**
* @return The current state of allowing or disallowing IPs originating within Azure through the firewall. If the firewall is disabled, this is not enforced.
*
*/
public String firewallAllowAzureIps() {
return this.firewallAllowAzureIps;
}
/**
* @return The list of firewall rules associated with this Data Lake Store account.
*
*/
public List firewallRules() {
return this.firewallRules;
}
/**
* @return The current state of the IP address firewall for this Data Lake Store account.
*
*/
public String firewallState() {
return this.firewallState;
}
/**
* @return The resource identifier.
*
*/
public String id() {
return this.id;
}
/**
* @return The Key Vault encryption identity, if any.
*
*/
public EncryptionIdentityResponse identity() {
return this.identity;
}
/**
* @return The account last modified time.
*
*/
public String lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* @return The resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return The resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The commitment tier to use for next month.
*
*/
public String newTier() {
return this.newTier;
}
/**
* @return The provisioning status of the Data Lake Store account.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The state of the Data Lake Store account.
*
*/
public String state() {
return this.state;
}
/**
* @return The resource tags.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return The current state of the trusted identity provider feature for this Data Lake Store account.
*
*/
public String trustedIdProviderState() {
return this.trustedIdProviderState;
}
/**
* @return The list of trusted identity providers associated with this Data Lake Store account.
*
*/
public List trustedIdProviders() {
return this.trustedIdProviders;
}
/**
* @return The resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The list of virtual network rules associated with this Data Lake Store account.
*
*/
public List virtualNetworkRules() {
return this.virtualNetworkRules;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAccountResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accountId;
private String creationTime;
private String currentTier;
private String defaultGroup;
private EncryptionConfigResponse encryptionConfig;
private String encryptionProvisioningState;
private String encryptionState;
private String endpoint;
private String firewallAllowAzureIps;
private List firewallRules;
private String firewallState;
private String id;
private EncryptionIdentityResponse identity;
private String lastModifiedTime;
private String location;
private String name;
private String newTier;
private String provisioningState;
private String state;
private Map tags;
private String trustedIdProviderState;
private List trustedIdProviders;
private String type;
private List virtualNetworkRules;
public Builder() {}
public Builder(GetAccountResult defaults) {
Objects.requireNonNull(defaults);
this.accountId = defaults.accountId;
this.creationTime = defaults.creationTime;
this.currentTier = defaults.currentTier;
this.defaultGroup = defaults.defaultGroup;
this.encryptionConfig = defaults.encryptionConfig;
this.encryptionProvisioningState = defaults.encryptionProvisioningState;
this.encryptionState = defaults.encryptionState;
this.endpoint = defaults.endpoint;
this.firewallAllowAzureIps = defaults.firewallAllowAzureIps;
this.firewallRules = defaults.firewallRules;
this.firewallState = defaults.firewallState;
this.id = defaults.id;
this.identity = defaults.identity;
this.lastModifiedTime = defaults.lastModifiedTime;
this.location = defaults.location;
this.name = defaults.name;
this.newTier = defaults.newTier;
this.provisioningState = defaults.provisioningState;
this.state = defaults.state;
this.tags = defaults.tags;
this.trustedIdProviderState = defaults.trustedIdProviderState;
this.trustedIdProviders = defaults.trustedIdProviders;
this.type = defaults.type;
this.virtualNetworkRules = defaults.virtualNetworkRules;
}
@CustomType.Setter
public Builder accountId(String accountId) {
if (accountId == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "accountId");
}
this.accountId = accountId;
return this;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder currentTier(String currentTier) {
if (currentTier == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "currentTier");
}
this.currentTier = currentTier;
return this;
}
@CustomType.Setter
public Builder defaultGroup(String defaultGroup) {
if (defaultGroup == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "defaultGroup");
}
this.defaultGroup = defaultGroup;
return this;
}
@CustomType.Setter
public Builder encryptionConfig(EncryptionConfigResponse encryptionConfig) {
if (encryptionConfig == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "encryptionConfig");
}
this.encryptionConfig = encryptionConfig;
return this;
}
@CustomType.Setter
public Builder encryptionProvisioningState(String encryptionProvisioningState) {
if (encryptionProvisioningState == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "encryptionProvisioningState");
}
this.encryptionProvisioningState = encryptionProvisioningState;
return this;
}
@CustomType.Setter
public Builder encryptionState(String encryptionState) {
if (encryptionState == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "encryptionState");
}
this.encryptionState = encryptionState;
return this;
}
@CustomType.Setter
public Builder endpoint(String endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder firewallAllowAzureIps(String firewallAllowAzureIps) {
if (firewallAllowAzureIps == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "firewallAllowAzureIps");
}
this.firewallAllowAzureIps = firewallAllowAzureIps;
return this;
}
@CustomType.Setter
public Builder firewallRules(List firewallRules) {
if (firewallRules == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "firewallRules");
}
this.firewallRules = firewallRules;
return this;
}
public Builder firewallRules(FirewallRuleResponse... firewallRules) {
return firewallRules(List.of(firewallRules));
}
@CustomType.Setter
public Builder firewallState(String firewallState) {
if (firewallState == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "firewallState");
}
this.firewallState = firewallState;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(EncryptionIdentityResponse identity) {
if (identity == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "identity");
}
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder lastModifiedTime(String lastModifiedTime) {
if (lastModifiedTime == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "lastModifiedTime");
}
this.lastModifiedTime = lastModifiedTime;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder newTier(String newTier) {
if (newTier == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "newTier");
}
this.newTier = newTier;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder trustedIdProviderState(String trustedIdProviderState) {
if (trustedIdProviderState == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "trustedIdProviderState");
}
this.trustedIdProviderState = trustedIdProviderState;
return this;
}
@CustomType.Setter
public Builder trustedIdProviders(List trustedIdProviders) {
if (trustedIdProviders == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "trustedIdProviders");
}
this.trustedIdProviders = trustedIdProviders;
return this;
}
public Builder trustedIdProviders(TrustedIdProviderResponse... trustedIdProviders) {
return trustedIdProviders(List.of(trustedIdProviders));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualNetworkRules(List virtualNetworkRules) {
if (virtualNetworkRules == null) {
throw new MissingRequiredPropertyException("GetAccountResult", "virtualNetworkRules");
}
this.virtualNetworkRules = virtualNetworkRules;
return this;
}
public Builder virtualNetworkRules(VirtualNetworkRuleResponse... virtualNetworkRules) {
return virtualNetworkRules(List.of(virtualNetworkRules));
}
public GetAccountResult build() {
final var _resultValue = new GetAccountResult();
_resultValue.accountId = accountId;
_resultValue.creationTime = creationTime;
_resultValue.currentTier = currentTier;
_resultValue.defaultGroup = defaultGroup;
_resultValue.encryptionConfig = encryptionConfig;
_resultValue.encryptionProvisioningState = encryptionProvisioningState;
_resultValue.encryptionState = encryptionState;
_resultValue.endpoint = endpoint;
_resultValue.firewallAllowAzureIps = firewallAllowAzureIps;
_resultValue.firewallRules = firewallRules;
_resultValue.firewallState = firewallState;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.lastModifiedTime = lastModifiedTime;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.newTier = newTier;
_resultValue.provisioningState = provisioningState;
_resultValue.state = state;
_resultValue.tags = tags;
_resultValue.trustedIdProviderState = trustedIdProviderState;
_resultValue.trustedIdProviders = trustedIdProviders;
_resultValue.type = type;
_resultValue.virtualNetworkRules = virtualNetworkRules;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy