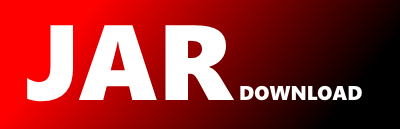
com.pulumi.azurenative.datamigration.inputs.MongoDbMigrationSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.inputs;
import com.pulumi.azurenative.datamigration.enums.MongoDbReplication;
import com.pulumi.azurenative.datamigration.inputs.MongoDbConnectionInfoArgs;
import com.pulumi.azurenative.datamigration.inputs.MongoDbDatabaseSettingsArgs;
import com.pulumi.azurenative.datamigration.inputs.MongoDbThrottlingSettingsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes how a MongoDB data migration should be performed
*
*/
public final class MongoDbMigrationSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final MongoDbMigrationSettingsArgs Empty = new MongoDbMigrationSettingsArgs();
/**
* The RU limit on a CosmosDB target that collections will be temporarily increased to (if lower) during the initial copy of a migration, from 10,000 to 1,000,000, or 0 to use the default boost (which is generally the maximum), or null to not boost the RUs. This setting has no effect on non-CosmosDB targets.
*
*/
@Import(name="boostRUs")
private @Nullable Output boostRUs;
/**
* @return The RU limit on a CosmosDB target that collections will be temporarily increased to (if lower) during the initial copy of a migration, from 10,000 to 1,000,000, or 0 to use the default boost (which is generally the maximum), or null to not boost the RUs. This setting has no effect on non-CosmosDB targets.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy