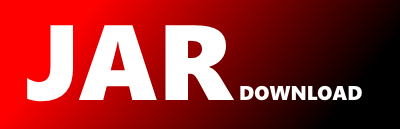
com.pulumi.azurenative.datamigration.outputs.DatabaseFileInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DatabaseFileInfoResponse {
/**
* @return Name of the database
*
*/
private @Nullable String databaseName;
/**
* @return Database file type
*
*/
private @Nullable String fileType;
/**
* @return Unique identifier for database file
*
*/
private @Nullable String id;
/**
* @return Logical name of the file
*
*/
private @Nullable String logicalName;
/**
* @return Operating-system full path of the file
*
*/
private @Nullable String physicalFullName;
/**
* @return Suggested full path of the file for restoring
*
*/
private @Nullable String restoreFullName;
/**
* @return Size of the file in megabytes
*
*/
private @Nullable Double sizeMB;
private DatabaseFileInfoResponse() {}
/**
* @return Name of the database
*
*/
public Optional databaseName() {
return Optional.ofNullable(this.databaseName);
}
/**
* @return Database file type
*
*/
public Optional fileType() {
return Optional.ofNullable(this.fileType);
}
/**
* @return Unique identifier for database file
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Logical name of the file
*
*/
public Optional logicalName() {
return Optional.ofNullable(this.logicalName);
}
/**
* @return Operating-system full path of the file
*
*/
public Optional physicalFullName() {
return Optional.ofNullable(this.physicalFullName);
}
/**
* @return Suggested full path of the file for restoring
*
*/
public Optional restoreFullName() {
return Optional.ofNullable(this.restoreFullName);
}
/**
* @return Size of the file in megabytes
*
*/
public Optional sizeMB() {
return Optional.ofNullable(this.sizeMB);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DatabaseFileInfoResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String databaseName;
private @Nullable String fileType;
private @Nullable String id;
private @Nullable String logicalName;
private @Nullable String physicalFullName;
private @Nullable String restoreFullName;
private @Nullable Double sizeMB;
public Builder() {}
public Builder(DatabaseFileInfoResponse defaults) {
Objects.requireNonNull(defaults);
this.databaseName = defaults.databaseName;
this.fileType = defaults.fileType;
this.id = defaults.id;
this.logicalName = defaults.logicalName;
this.physicalFullName = defaults.physicalFullName;
this.restoreFullName = defaults.restoreFullName;
this.sizeMB = defaults.sizeMB;
}
@CustomType.Setter
public Builder databaseName(@Nullable String databaseName) {
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder fileType(@Nullable String fileType) {
this.fileType = fileType;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder logicalName(@Nullable String logicalName) {
this.logicalName = logicalName;
return this;
}
@CustomType.Setter
public Builder physicalFullName(@Nullable String physicalFullName) {
this.physicalFullName = physicalFullName;
return this;
}
@CustomType.Setter
public Builder restoreFullName(@Nullable String restoreFullName) {
this.restoreFullName = restoreFullName;
return this;
}
@CustomType.Setter
public Builder sizeMB(@Nullable Double sizeMB) {
this.sizeMB = sizeMB;
return this;
}
public DatabaseFileInfoResponse build() {
final var _resultValue = new DatabaseFileInfoResponse();
_resultValue.databaseName = databaseName;
_resultValue.fileType = fileType;
_resultValue.id = id;
_resultValue.logicalName = logicalName;
_resultValue.physicalFullName = physicalFullName;
_resultValue.restoreFullName = restoreFullName;
_resultValue.sizeMB = sizeMB;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy