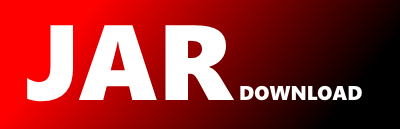
com.pulumi.azurenative.datamigration.outputs.MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.DataItemMigrationSummaryResultResponse;
import com.pulumi.azurenative.datamigration.outputs.ReportableExceptionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse {
/**
* @return Name of the database
*
*/
private String databaseName;
/**
* @return Migration end time
*
*/
private String endedOn;
/**
* @return Number of database/object errors.
*
*/
private Double errorCount;
/**
* @return Wildcard string prefix to use for querying all errors of the item
*
*/
private String errorPrefix;
/**
* @return Migration exceptions and warnings.
*
*/
private List exceptionsAndWarnings;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Last time the storage was updated
*
*/
private String lastStorageUpdate;
/**
* @return Migration progress message
*
*/
private String message;
/**
* @return Number of objects
*
*/
private Double numberOfObjects;
/**
* @return Number of successfully completed objects
*
*/
private Double numberOfObjectsCompleted;
/**
* @return Summary of object results in the migration
*
*/
private Map objectSummary;
/**
* @return Wildcard string prefix to use for querying all sub-tem results of the item
*
*/
private String resultPrefix;
/**
* @return Result type
* Expected value is 'DatabaseLevelOutput'.
*
*/
private String resultType;
/**
* @return Migration stage that this database is in
*
*/
private String stage;
/**
* @return Migration start time
*
*/
private String startedOn;
/**
* @return Current state of migration
*
*/
private String state;
/**
* @return Status message
*
*/
private String statusMessage;
private MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse() {}
/**
* @return Name of the database
*
*/
public String databaseName() {
return this.databaseName;
}
/**
* @return Migration end time
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return Number of database/object errors.
*
*/
public Double errorCount() {
return this.errorCount;
}
/**
* @return Wildcard string prefix to use for querying all errors of the item
*
*/
public String errorPrefix() {
return this.errorPrefix;
}
/**
* @return Migration exceptions and warnings.
*
*/
public List exceptionsAndWarnings() {
return this.exceptionsAndWarnings;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Last time the storage was updated
*
*/
public String lastStorageUpdate() {
return this.lastStorageUpdate;
}
/**
* @return Migration progress message
*
*/
public String message() {
return this.message;
}
/**
* @return Number of objects
*
*/
public Double numberOfObjects() {
return this.numberOfObjects;
}
/**
* @return Number of successfully completed objects
*
*/
public Double numberOfObjectsCompleted() {
return this.numberOfObjectsCompleted;
}
/**
* @return Summary of object results in the migration
*
*/
public Map objectSummary() {
return this.objectSummary;
}
/**
* @return Wildcard string prefix to use for querying all sub-tem results of the item
*
*/
public String resultPrefix() {
return this.resultPrefix;
}
/**
* @return Result type
* Expected value is 'DatabaseLevelOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Migration stage that this database is in
*
*/
public String stage() {
return this.stage;
}
/**
* @return Migration start time
*
*/
public String startedOn() {
return this.startedOn;
}
/**
* @return Current state of migration
*
*/
public String state() {
return this.state;
}
/**
* @return Status message
*
*/
public String statusMessage() {
return this.statusMessage;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String databaseName;
private String endedOn;
private Double errorCount;
private String errorPrefix;
private List exceptionsAndWarnings;
private String id;
private String lastStorageUpdate;
private String message;
private Double numberOfObjects;
private Double numberOfObjectsCompleted;
private Map objectSummary;
private String resultPrefix;
private String resultType;
private String stage;
private String startedOn;
private String state;
private String statusMessage;
public Builder() {}
public Builder(MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse defaults) {
Objects.requireNonNull(defaults);
this.databaseName = defaults.databaseName;
this.endedOn = defaults.endedOn;
this.errorCount = defaults.errorCount;
this.errorPrefix = defaults.errorPrefix;
this.exceptionsAndWarnings = defaults.exceptionsAndWarnings;
this.id = defaults.id;
this.lastStorageUpdate = defaults.lastStorageUpdate;
this.message = defaults.message;
this.numberOfObjects = defaults.numberOfObjects;
this.numberOfObjectsCompleted = defaults.numberOfObjectsCompleted;
this.objectSummary = defaults.objectSummary;
this.resultPrefix = defaults.resultPrefix;
this.resultType = defaults.resultType;
this.stage = defaults.stage;
this.startedOn = defaults.startedOn;
this.state = defaults.state;
this.statusMessage = defaults.statusMessage;
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder errorCount(Double errorCount) {
if (errorCount == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "errorCount");
}
this.errorCount = errorCount;
return this;
}
@CustomType.Setter
public Builder errorPrefix(String errorPrefix) {
if (errorPrefix == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "errorPrefix");
}
this.errorPrefix = errorPrefix;
return this;
}
@CustomType.Setter
public Builder exceptionsAndWarnings(List exceptionsAndWarnings) {
if (exceptionsAndWarnings == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "exceptionsAndWarnings");
}
this.exceptionsAndWarnings = exceptionsAndWarnings;
return this;
}
public Builder exceptionsAndWarnings(ReportableExceptionResponse... exceptionsAndWarnings) {
return exceptionsAndWarnings(List.of(exceptionsAndWarnings));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastStorageUpdate(String lastStorageUpdate) {
if (lastStorageUpdate == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "lastStorageUpdate");
}
this.lastStorageUpdate = lastStorageUpdate;
return this;
}
@CustomType.Setter
public Builder message(String message) {
if (message == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "message");
}
this.message = message;
return this;
}
@CustomType.Setter
public Builder numberOfObjects(Double numberOfObjects) {
if (numberOfObjects == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "numberOfObjects");
}
this.numberOfObjects = numberOfObjects;
return this;
}
@CustomType.Setter
public Builder numberOfObjectsCompleted(Double numberOfObjectsCompleted) {
if (numberOfObjectsCompleted == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "numberOfObjectsCompleted");
}
this.numberOfObjectsCompleted = numberOfObjectsCompleted;
return this;
}
@CustomType.Setter
public Builder objectSummary(Map objectSummary) {
if (objectSummary == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "objectSummary");
}
this.objectSummary = objectSummary;
return this;
}
@CustomType.Setter
public Builder resultPrefix(String resultPrefix) {
if (resultPrefix == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "resultPrefix");
}
this.resultPrefix = resultPrefix;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder stage(String stage) {
if (stage == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "stage");
}
this.stage = stage;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "startedOn");
}
this.startedOn = startedOn;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder statusMessage(String statusMessage) {
if (statusMessage == null) {
throw new MissingRequiredPropertyException("MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse", "statusMessage");
}
this.statusMessage = statusMessage;
return this;
}
public MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse build() {
final var _resultValue = new MigrateMySqlAzureDbForMySqlOfflineTaskOutputDatabaseLevelResponse();
_resultValue.databaseName = databaseName;
_resultValue.endedOn = endedOn;
_resultValue.errorCount = errorCount;
_resultValue.errorPrefix = errorPrefix;
_resultValue.exceptionsAndWarnings = exceptionsAndWarnings;
_resultValue.id = id;
_resultValue.lastStorageUpdate = lastStorageUpdate;
_resultValue.message = message;
_resultValue.numberOfObjects = numberOfObjects;
_resultValue.numberOfObjectsCompleted = numberOfObjectsCompleted;
_resultValue.objectSummary = objectSummary;
_resultValue.resultPrefix = resultPrefix;
_resultValue.resultType = resultType;
_resultValue.stage = stage;
_resultValue.startedOn = startedOn;
_resultValue.state = state;
_resultValue.statusMessage = statusMessage;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy