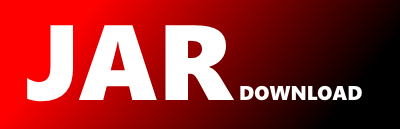
com.pulumi.azurenative.datamigration.outputs.MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse {
/**
* @return Number of applied deletes
*
*/
private Double cdcDeleteCounter;
/**
* @return Number of applied inserts
*
*/
private Double cdcInsertCounter;
/**
* @return Number of applied updates
*
*/
private Double cdcUpdateCounter;
/**
* @return Number of data errors occurred
*
*/
private Double dataErrorsCounter;
/**
* @return Name of the database
*
*/
private String databaseName;
/**
* @return Full load end time
*
*/
private String fullLoadEndedOn;
/**
* @return Estimate to finish full load
*
*/
private String fullLoadEstFinishTime;
/**
* @return Full load start time
*
*/
private String fullLoadStartedOn;
/**
* @return Number of rows applied in full load
*
*/
private Double fullLoadTotalRows;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Last modified time on target
*
*/
private String lastModifiedTime;
/**
* @return Result type
* Expected value is 'TableLevelOutput'.
*
*/
private String resultType;
/**
* @return Current state of the table migration
*
*/
private String state;
/**
* @return Name of the table
*
*/
private String tableName;
/**
* @return Total number of applied changes
*
*/
private Double totalChangesApplied;
private MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse() {}
/**
* @return Number of applied deletes
*
*/
public Double cdcDeleteCounter() {
return this.cdcDeleteCounter;
}
/**
* @return Number of applied inserts
*
*/
public Double cdcInsertCounter() {
return this.cdcInsertCounter;
}
/**
* @return Number of applied updates
*
*/
public Double cdcUpdateCounter() {
return this.cdcUpdateCounter;
}
/**
* @return Number of data errors occurred
*
*/
public Double dataErrorsCounter() {
return this.dataErrorsCounter;
}
/**
* @return Name of the database
*
*/
public String databaseName() {
return this.databaseName;
}
/**
* @return Full load end time
*
*/
public String fullLoadEndedOn() {
return this.fullLoadEndedOn;
}
/**
* @return Estimate to finish full load
*
*/
public String fullLoadEstFinishTime() {
return this.fullLoadEstFinishTime;
}
/**
* @return Full load start time
*
*/
public String fullLoadStartedOn() {
return this.fullLoadStartedOn;
}
/**
* @return Number of rows applied in full load
*
*/
public Double fullLoadTotalRows() {
return this.fullLoadTotalRows;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Last modified time on target
*
*/
public String lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* @return Result type
* Expected value is 'TableLevelOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Current state of the table migration
*
*/
public String state() {
return this.state;
}
/**
* @return Name of the table
*
*/
public String tableName() {
return this.tableName;
}
/**
* @return Total number of applied changes
*
*/
public Double totalChangesApplied() {
return this.totalChangesApplied;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double cdcDeleteCounter;
private Double cdcInsertCounter;
private Double cdcUpdateCounter;
private Double dataErrorsCounter;
private String databaseName;
private String fullLoadEndedOn;
private String fullLoadEstFinishTime;
private String fullLoadStartedOn;
private Double fullLoadTotalRows;
private String id;
private String lastModifiedTime;
private String resultType;
private String state;
private String tableName;
private Double totalChangesApplied;
public Builder() {}
public Builder(MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse defaults) {
Objects.requireNonNull(defaults);
this.cdcDeleteCounter = defaults.cdcDeleteCounter;
this.cdcInsertCounter = defaults.cdcInsertCounter;
this.cdcUpdateCounter = defaults.cdcUpdateCounter;
this.dataErrorsCounter = defaults.dataErrorsCounter;
this.databaseName = defaults.databaseName;
this.fullLoadEndedOn = defaults.fullLoadEndedOn;
this.fullLoadEstFinishTime = defaults.fullLoadEstFinishTime;
this.fullLoadStartedOn = defaults.fullLoadStartedOn;
this.fullLoadTotalRows = defaults.fullLoadTotalRows;
this.id = defaults.id;
this.lastModifiedTime = defaults.lastModifiedTime;
this.resultType = defaults.resultType;
this.state = defaults.state;
this.tableName = defaults.tableName;
this.totalChangesApplied = defaults.totalChangesApplied;
}
@CustomType.Setter
public Builder cdcDeleteCounter(Double cdcDeleteCounter) {
if (cdcDeleteCounter == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "cdcDeleteCounter");
}
this.cdcDeleteCounter = cdcDeleteCounter;
return this;
}
@CustomType.Setter
public Builder cdcInsertCounter(Double cdcInsertCounter) {
if (cdcInsertCounter == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "cdcInsertCounter");
}
this.cdcInsertCounter = cdcInsertCounter;
return this;
}
@CustomType.Setter
public Builder cdcUpdateCounter(Double cdcUpdateCounter) {
if (cdcUpdateCounter == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "cdcUpdateCounter");
}
this.cdcUpdateCounter = cdcUpdateCounter;
return this;
}
@CustomType.Setter
public Builder dataErrorsCounter(Double dataErrorsCounter) {
if (dataErrorsCounter == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "dataErrorsCounter");
}
this.dataErrorsCounter = dataErrorsCounter;
return this;
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder fullLoadEndedOn(String fullLoadEndedOn) {
if (fullLoadEndedOn == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "fullLoadEndedOn");
}
this.fullLoadEndedOn = fullLoadEndedOn;
return this;
}
@CustomType.Setter
public Builder fullLoadEstFinishTime(String fullLoadEstFinishTime) {
if (fullLoadEstFinishTime == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "fullLoadEstFinishTime");
}
this.fullLoadEstFinishTime = fullLoadEstFinishTime;
return this;
}
@CustomType.Setter
public Builder fullLoadStartedOn(String fullLoadStartedOn) {
if (fullLoadStartedOn == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "fullLoadStartedOn");
}
this.fullLoadStartedOn = fullLoadStartedOn;
return this;
}
@CustomType.Setter
public Builder fullLoadTotalRows(Double fullLoadTotalRows) {
if (fullLoadTotalRows == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "fullLoadTotalRows");
}
this.fullLoadTotalRows = fullLoadTotalRows;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastModifiedTime(String lastModifiedTime) {
if (lastModifiedTime == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "lastModifiedTime");
}
this.lastModifiedTime = lastModifiedTime;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder tableName(String tableName) {
if (tableName == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "tableName");
}
this.tableName = tableName;
return this;
}
@CustomType.Setter
public Builder totalChangesApplied(Double totalChangesApplied) {
if (totalChangesApplied == null) {
throw new MissingRequiredPropertyException("MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse", "totalChangesApplied");
}
this.totalChangesApplied = totalChangesApplied;
return this;
}
public MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse build() {
final var _resultValue = new MigratePostgreSqlAzureDbForPostgreSqlSyncTaskOutputTableLevelResponse();
_resultValue.cdcDeleteCounter = cdcDeleteCounter;
_resultValue.cdcInsertCounter = cdcInsertCounter;
_resultValue.cdcUpdateCounter = cdcUpdateCounter;
_resultValue.dataErrorsCounter = dataErrorsCounter;
_resultValue.databaseName = databaseName;
_resultValue.fullLoadEndedOn = fullLoadEndedOn;
_resultValue.fullLoadEstFinishTime = fullLoadEstFinishTime;
_resultValue.fullLoadStartedOn = fullLoadStartedOn;
_resultValue.fullLoadTotalRows = fullLoadTotalRows;
_resultValue.id = id;
_resultValue.lastModifiedTime = lastModifiedTime;
_resultValue.resultType = resultType;
_resultValue.state = state;
_resultValue.tableName = tableName;
_resultValue.totalChangesApplied = totalChangesApplied;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy