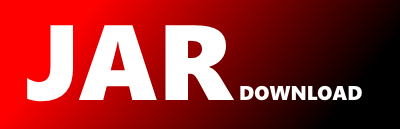
com.pulumi.azurenative.datamigration.outputs.MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse {
/**
* @return Number of applied changes
*
*/
private Double appliedChanges;
/**
* @return Number of cdc deletes
*
*/
private Double cdcDeleteCounter;
/**
* @return Number of cdc inserts
*
*/
private Double cdcInsertCounter;
/**
* @return Number of cdc updates
*
*/
private Double cdcUpdateCounter;
/**
* @return Name of the database
*
*/
private String databaseName;
/**
* @return Migration end time
*
*/
private String endedOn;
/**
* @return Number of tables completed in full load
*
*/
private Double fullLoadCompletedTables;
/**
* @return Number of tables errored in full load
*
*/
private Double fullLoadErroredTables;
/**
* @return Number of tables loading in full load
*
*/
private Double fullLoadLoadingTables;
/**
* @return Number of tables queued in full load
*
*/
private Double fullLoadQueuedTables;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Number of incoming changes
*
*/
private Double incomingChanges;
/**
* @return Indicates if initial load (full load) has been completed
*
*/
private Boolean initializationCompleted;
/**
* @return CDC apply latency
*
*/
private Double latency;
/**
* @return Migration state that this database is in
*
*/
private String migrationState;
/**
* @return Result type
* Expected value is 'DatabaseLevelOutput'.
*
*/
private String resultType;
/**
* @return Migration start time
*
*/
private String startedOn;
private MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse() {}
/**
* @return Number of applied changes
*
*/
public Double appliedChanges() {
return this.appliedChanges;
}
/**
* @return Number of cdc deletes
*
*/
public Double cdcDeleteCounter() {
return this.cdcDeleteCounter;
}
/**
* @return Number of cdc inserts
*
*/
public Double cdcInsertCounter() {
return this.cdcInsertCounter;
}
/**
* @return Number of cdc updates
*
*/
public Double cdcUpdateCounter() {
return this.cdcUpdateCounter;
}
/**
* @return Name of the database
*
*/
public String databaseName() {
return this.databaseName;
}
/**
* @return Migration end time
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return Number of tables completed in full load
*
*/
public Double fullLoadCompletedTables() {
return this.fullLoadCompletedTables;
}
/**
* @return Number of tables errored in full load
*
*/
public Double fullLoadErroredTables() {
return this.fullLoadErroredTables;
}
/**
* @return Number of tables loading in full load
*
*/
public Double fullLoadLoadingTables() {
return this.fullLoadLoadingTables;
}
/**
* @return Number of tables queued in full load
*
*/
public Double fullLoadQueuedTables() {
return this.fullLoadQueuedTables;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Number of incoming changes
*
*/
public Double incomingChanges() {
return this.incomingChanges;
}
/**
* @return Indicates if initial load (full load) has been completed
*
*/
public Boolean initializationCompleted() {
return this.initializationCompleted;
}
/**
* @return CDC apply latency
*
*/
public Double latency() {
return this.latency;
}
/**
* @return Migration state that this database is in
*
*/
public String migrationState() {
return this.migrationState;
}
/**
* @return Result type
* Expected value is 'DatabaseLevelOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Migration start time
*
*/
public String startedOn() {
return this.startedOn;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double appliedChanges;
private Double cdcDeleteCounter;
private Double cdcInsertCounter;
private Double cdcUpdateCounter;
private String databaseName;
private String endedOn;
private Double fullLoadCompletedTables;
private Double fullLoadErroredTables;
private Double fullLoadLoadingTables;
private Double fullLoadQueuedTables;
private String id;
private Double incomingChanges;
private Boolean initializationCompleted;
private Double latency;
private String migrationState;
private String resultType;
private String startedOn;
public Builder() {}
public Builder(MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse defaults) {
Objects.requireNonNull(defaults);
this.appliedChanges = defaults.appliedChanges;
this.cdcDeleteCounter = defaults.cdcDeleteCounter;
this.cdcInsertCounter = defaults.cdcInsertCounter;
this.cdcUpdateCounter = defaults.cdcUpdateCounter;
this.databaseName = defaults.databaseName;
this.endedOn = defaults.endedOn;
this.fullLoadCompletedTables = defaults.fullLoadCompletedTables;
this.fullLoadErroredTables = defaults.fullLoadErroredTables;
this.fullLoadLoadingTables = defaults.fullLoadLoadingTables;
this.fullLoadQueuedTables = defaults.fullLoadQueuedTables;
this.id = defaults.id;
this.incomingChanges = defaults.incomingChanges;
this.initializationCompleted = defaults.initializationCompleted;
this.latency = defaults.latency;
this.migrationState = defaults.migrationState;
this.resultType = defaults.resultType;
this.startedOn = defaults.startedOn;
}
@CustomType.Setter
public Builder appliedChanges(Double appliedChanges) {
if (appliedChanges == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "appliedChanges");
}
this.appliedChanges = appliedChanges;
return this;
}
@CustomType.Setter
public Builder cdcDeleteCounter(Double cdcDeleteCounter) {
if (cdcDeleteCounter == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "cdcDeleteCounter");
}
this.cdcDeleteCounter = cdcDeleteCounter;
return this;
}
@CustomType.Setter
public Builder cdcInsertCounter(Double cdcInsertCounter) {
if (cdcInsertCounter == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "cdcInsertCounter");
}
this.cdcInsertCounter = cdcInsertCounter;
return this;
}
@CustomType.Setter
public Builder cdcUpdateCounter(Double cdcUpdateCounter) {
if (cdcUpdateCounter == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "cdcUpdateCounter");
}
this.cdcUpdateCounter = cdcUpdateCounter;
return this;
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder fullLoadCompletedTables(Double fullLoadCompletedTables) {
if (fullLoadCompletedTables == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "fullLoadCompletedTables");
}
this.fullLoadCompletedTables = fullLoadCompletedTables;
return this;
}
@CustomType.Setter
public Builder fullLoadErroredTables(Double fullLoadErroredTables) {
if (fullLoadErroredTables == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "fullLoadErroredTables");
}
this.fullLoadErroredTables = fullLoadErroredTables;
return this;
}
@CustomType.Setter
public Builder fullLoadLoadingTables(Double fullLoadLoadingTables) {
if (fullLoadLoadingTables == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "fullLoadLoadingTables");
}
this.fullLoadLoadingTables = fullLoadLoadingTables;
return this;
}
@CustomType.Setter
public Builder fullLoadQueuedTables(Double fullLoadQueuedTables) {
if (fullLoadQueuedTables == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "fullLoadQueuedTables");
}
this.fullLoadQueuedTables = fullLoadQueuedTables;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder incomingChanges(Double incomingChanges) {
if (incomingChanges == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "incomingChanges");
}
this.incomingChanges = incomingChanges;
return this;
}
@CustomType.Setter
public Builder initializationCompleted(Boolean initializationCompleted) {
if (initializationCompleted == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "initializationCompleted");
}
this.initializationCompleted = initializationCompleted;
return this;
}
@CustomType.Setter
public Builder latency(Double latency) {
if (latency == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "latency");
}
this.latency = latency;
return this;
}
@CustomType.Setter
public Builder migrationState(String migrationState) {
if (migrationState == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "migrationState");
}
this.migrationState = migrationState;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse", "startedOn");
}
this.startedOn = startedOn;
return this;
}
public MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse build() {
final var _resultValue = new MigrateSqlServerSqlDbSyncTaskOutputDatabaseLevelResponse();
_resultValue.appliedChanges = appliedChanges;
_resultValue.cdcDeleteCounter = cdcDeleteCounter;
_resultValue.cdcInsertCounter = cdcInsertCounter;
_resultValue.cdcUpdateCounter = cdcUpdateCounter;
_resultValue.databaseName = databaseName;
_resultValue.endedOn = endedOn;
_resultValue.fullLoadCompletedTables = fullLoadCompletedTables;
_resultValue.fullLoadErroredTables = fullLoadErroredTables;
_resultValue.fullLoadLoadingTables = fullLoadLoadingTables;
_resultValue.fullLoadQueuedTables = fullLoadQueuedTables;
_resultValue.id = id;
_resultValue.incomingChanges = incomingChanges;
_resultValue.initializationCompleted = initializationCompleted;
_resultValue.latency = latency;
_resultValue.migrationState = migrationState;
_resultValue.resultType = resultType;
_resultValue.startedOn = startedOn;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy