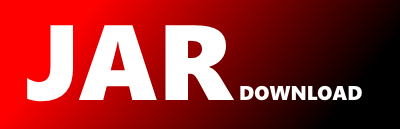
com.pulumi.azurenative.datamigration.outputs.MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.DataIntegrityValidationResultResponse;
import com.pulumi.azurenative.datamigration.outputs.QueryAnalysisValidationResultResponse;
import com.pulumi.azurenative.datamigration.outputs.SchemaComparisonValidationResultResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse {
/**
* @return Provides data integrity validation result between the source and target tables that are migrated.
*
*/
private DataIntegrityValidationResultResponse dataIntegrityValidationResult;
/**
* @return Validation end time
*
*/
private String endedOn;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Migration Identifier
*
*/
private String migrationId;
/**
* @return Results of some of the query execution result between source and target database
*
*/
private QueryAnalysisValidationResultResponse queryAnalysisValidationResult;
/**
* @return Result type
* Expected value is 'MigrationDatabaseLevelValidationOutput'.
*
*/
private String resultType;
/**
* @return Provides schema comparison result between source and target database
*
*/
private SchemaComparisonValidationResultResponse schemaValidationResult;
/**
* @return Name of the source database
*
*/
private String sourceDatabaseName;
/**
* @return Validation start time
*
*/
private String startedOn;
/**
* @return Current status of validation at the database level
*
*/
private String status;
/**
* @return Name of the target database
*
*/
private String targetDatabaseName;
private MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse() {}
/**
* @return Provides data integrity validation result between the source and target tables that are migrated.
*
*/
public DataIntegrityValidationResultResponse dataIntegrityValidationResult() {
return this.dataIntegrityValidationResult;
}
/**
* @return Validation end time
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Migration Identifier
*
*/
public String migrationId() {
return this.migrationId;
}
/**
* @return Results of some of the query execution result between source and target database
*
*/
public QueryAnalysisValidationResultResponse queryAnalysisValidationResult() {
return this.queryAnalysisValidationResult;
}
/**
* @return Result type
* Expected value is 'MigrationDatabaseLevelValidationOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Provides schema comparison result between source and target database
*
*/
public SchemaComparisonValidationResultResponse schemaValidationResult() {
return this.schemaValidationResult;
}
/**
* @return Name of the source database
*
*/
public String sourceDatabaseName() {
return this.sourceDatabaseName;
}
/**
* @return Validation start time
*
*/
public String startedOn() {
return this.startedOn;
}
/**
* @return Current status of validation at the database level
*
*/
public String status() {
return this.status;
}
/**
* @return Name of the target database
*
*/
public String targetDatabaseName() {
return this.targetDatabaseName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private DataIntegrityValidationResultResponse dataIntegrityValidationResult;
private String endedOn;
private String id;
private String migrationId;
private QueryAnalysisValidationResultResponse queryAnalysisValidationResult;
private String resultType;
private SchemaComparisonValidationResultResponse schemaValidationResult;
private String sourceDatabaseName;
private String startedOn;
private String status;
private String targetDatabaseName;
public Builder() {}
public Builder(MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse defaults) {
Objects.requireNonNull(defaults);
this.dataIntegrityValidationResult = defaults.dataIntegrityValidationResult;
this.endedOn = defaults.endedOn;
this.id = defaults.id;
this.migrationId = defaults.migrationId;
this.queryAnalysisValidationResult = defaults.queryAnalysisValidationResult;
this.resultType = defaults.resultType;
this.schemaValidationResult = defaults.schemaValidationResult;
this.sourceDatabaseName = defaults.sourceDatabaseName;
this.startedOn = defaults.startedOn;
this.status = defaults.status;
this.targetDatabaseName = defaults.targetDatabaseName;
}
@CustomType.Setter
public Builder dataIntegrityValidationResult(DataIntegrityValidationResultResponse dataIntegrityValidationResult) {
if (dataIntegrityValidationResult == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "dataIntegrityValidationResult");
}
this.dataIntegrityValidationResult = dataIntegrityValidationResult;
return this;
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder migrationId(String migrationId) {
if (migrationId == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "migrationId");
}
this.migrationId = migrationId;
return this;
}
@CustomType.Setter
public Builder queryAnalysisValidationResult(QueryAnalysisValidationResultResponse queryAnalysisValidationResult) {
if (queryAnalysisValidationResult == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "queryAnalysisValidationResult");
}
this.queryAnalysisValidationResult = queryAnalysisValidationResult;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder schemaValidationResult(SchemaComparisonValidationResultResponse schemaValidationResult) {
if (schemaValidationResult == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "schemaValidationResult");
}
this.schemaValidationResult = schemaValidationResult;
return this;
}
@CustomType.Setter
public Builder sourceDatabaseName(String sourceDatabaseName) {
if (sourceDatabaseName == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "sourceDatabaseName");
}
this.sourceDatabaseName = sourceDatabaseName;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "startedOn");
}
this.startedOn = startedOn;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder targetDatabaseName(String targetDatabaseName) {
if (targetDatabaseName == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse", "targetDatabaseName");
}
this.targetDatabaseName = targetDatabaseName;
return this;
}
public MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse build() {
final var _resultValue = new MigrateSqlServerSqlDbTaskOutputDatabaseLevelValidationResultResponse();
_resultValue.dataIntegrityValidationResult = dataIntegrityValidationResult;
_resultValue.endedOn = endedOn;
_resultValue.id = id;
_resultValue.migrationId = migrationId;
_resultValue.queryAnalysisValidationResult = queryAnalysisValidationResult;
_resultValue.resultType = resultType;
_resultValue.schemaValidationResult = schemaValidationResult;
_resultValue.sourceDatabaseName = sourceDatabaseName;
_resultValue.startedOn = startedOn;
_resultValue.status = status;
_resultValue.targetDatabaseName = targetDatabaseName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy