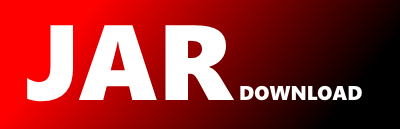
com.pulumi.azurenative.datamigration.outputs.MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.BackupSetInfoResponse;
import com.pulumi.azurenative.datamigration.outputs.ReportableExceptionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse {
/**
* @return Backup sets that are currently active (Either being uploaded or getting restored)
*
*/
private List activeBackupSets;
/**
* @return Name of container created in the Azure Storage account where backups are copied to
*
*/
private String containerName;
/**
* @return Database migration end time
*
*/
private String endedOn;
/**
* @return prefix string to use for querying errors for this database
*
*/
private String errorPrefix;
/**
* @return Migration exceptions and warnings
*
*/
private List exceptionsAndWarnings;
/**
* @return Details of full backup set
*
*/
private BackupSetInfoResponse fullBackupSetInfo;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Whether full backup has been applied to the target database or not
*
*/
private Boolean isFullBackupRestored;
/**
* @return Last applied backup set information
*
*/
private BackupSetInfoResponse lastRestoredBackupSetInfo;
/**
* @return Current state of database
*
*/
private String migrationState;
/**
* @return Result type
* Expected value is 'DatabaseLevelOutput'.
*
*/
private String resultType;
/**
* @return Name of the database
*
*/
private String sourceDatabaseName;
/**
* @return Database migration start time
*
*/
private String startedOn;
private MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse() {}
/**
* @return Backup sets that are currently active (Either being uploaded or getting restored)
*
*/
public List activeBackupSets() {
return this.activeBackupSets;
}
/**
* @return Name of container created in the Azure Storage account where backups are copied to
*
*/
public String containerName() {
return this.containerName;
}
/**
* @return Database migration end time
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return prefix string to use for querying errors for this database
*
*/
public String errorPrefix() {
return this.errorPrefix;
}
/**
* @return Migration exceptions and warnings
*
*/
public List exceptionsAndWarnings() {
return this.exceptionsAndWarnings;
}
/**
* @return Details of full backup set
*
*/
public BackupSetInfoResponse fullBackupSetInfo() {
return this.fullBackupSetInfo;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Whether full backup has been applied to the target database or not
*
*/
public Boolean isFullBackupRestored() {
return this.isFullBackupRestored;
}
/**
* @return Last applied backup set information
*
*/
public BackupSetInfoResponse lastRestoredBackupSetInfo() {
return this.lastRestoredBackupSetInfo;
}
/**
* @return Current state of database
*
*/
public String migrationState() {
return this.migrationState;
}
/**
* @return Result type
* Expected value is 'DatabaseLevelOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Name of the database
*
*/
public String sourceDatabaseName() {
return this.sourceDatabaseName;
}
/**
* @return Database migration start time
*
*/
public String startedOn() {
return this.startedOn;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List activeBackupSets;
private String containerName;
private String endedOn;
private String errorPrefix;
private List exceptionsAndWarnings;
private BackupSetInfoResponse fullBackupSetInfo;
private String id;
private Boolean isFullBackupRestored;
private BackupSetInfoResponse lastRestoredBackupSetInfo;
private String migrationState;
private String resultType;
private String sourceDatabaseName;
private String startedOn;
public Builder() {}
public Builder(MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse defaults) {
Objects.requireNonNull(defaults);
this.activeBackupSets = defaults.activeBackupSets;
this.containerName = defaults.containerName;
this.endedOn = defaults.endedOn;
this.errorPrefix = defaults.errorPrefix;
this.exceptionsAndWarnings = defaults.exceptionsAndWarnings;
this.fullBackupSetInfo = defaults.fullBackupSetInfo;
this.id = defaults.id;
this.isFullBackupRestored = defaults.isFullBackupRestored;
this.lastRestoredBackupSetInfo = defaults.lastRestoredBackupSetInfo;
this.migrationState = defaults.migrationState;
this.resultType = defaults.resultType;
this.sourceDatabaseName = defaults.sourceDatabaseName;
this.startedOn = defaults.startedOn;
}
@CustomType.Setter
public Builder activeBackupSets(List activeBackupSets) {
if (activeBackupSets == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "activeBackupSets");
}
this.activeBackupSets = activeBackupSets;
return this;
}
public Builder activeBackupSets(BackupSetInfoResponse... activeBackupSets) {
return activeBackupSets(List.of(activeBackupSets));
}
@CustomType.Setter
public Builder containerName(String containerName) {
if (containerName == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "containerName");
}
this.containerName = containerName;
return this;
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder errorPrefix(String errorPrefix) {
if (errorPrefix == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "errorPrefix");
}
this.errorPrefix = errorPrefix;
return this;
}
@CustomType.Setter
public Builder exceptionsAndWarnings(List exceptionsAndWarnings) {
if (exceptionsAndWarnings == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "exceptionsAndWarnings");
}
this.exceptionsAndWarnings = exceptionsAndWarnings;
return this;
}
public Builder exceptionsAndWarnings(ReportableExceptionResponse... exceptionsAndWarnings) {
return exceptionsAndWarnings(List.of(exceptionsAndWarnings));
}
@CustomType.Setter
public Builder fullBackupSetInfo(BackupSetInfoResponse fullBackupSetInfo) {
if (fullBackupSetInfo == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "fullBackupSetInfo");
}
this.fullBackupSetInfo = fullBackupSetInfo;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isFullBackupRestored(Boolean isFullBackupRestored) {
if (isFullBackupRestored == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "isFullBackupRestored");
}
this.isFullBackupRestored = isFullBackupRestored;
return this;
}
@CustomType.Setter
public Builder lastRestoredBackupSetInfo(BackupSetInfoResponse lastRestoredBackupSetInfo) {
if (lastRestoredBackupSetInfo == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "lastRestoredBackupSetInfo");
}
this.lastRestoredBackupSetInfo = lastRestoredBackupSetInfo;
return this;
}
@CustomType.Setter
public Builder migrationState(String migrationState) {
if (migrationState == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "migrationState");
}
this.migrationState = migrationState;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder sourceDatabaseName(String sourceDatabaseName) {
if (sourceDatabaseName == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "sourceDatabaseName");
}
this.sourceDatabaseName = sourceDatabaseName;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse", "startedOn");
}
this.startedOn = startedOn;
return this;
}
public MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse build() {
final var _resultValue = new MigrateSqlServerSqlMISyncTaskOutputDatabaseLevelResponse();
_resultValue.activeBackupSets = activeBackupSets;
_resultValue.containerName = containerName;
_resultValue.endedOn = endedOn;
_resultValue.errorPrefix = errorPrefix;
_resultValue.exceptionsAndWarnings = exceptionsAndWarnings;
_resultValue.fullBackupSetInfo = fullBackupSetInfo;
_resultValue.id = id;
_resultValue.isFullBackupRestored = isFullBackupRestored;
_resultValue.lastRestoredBackupSetInfo = lastRestoredBackupSetInfo;
_resultValue.migrationState = migrationState;
_resultValue.resultType = resultType;
_resultValue.sourceDatabaseName = sourceDatabaseName;
_resultValue.startedOn = startedOn;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy