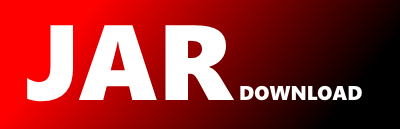
com.pulumi.azurenative.datamigration.outputs.MongoDbCollectionInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.MongoDbShardKeyInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MongoDbCollectionInfoResponse {
/**
* @return The average document size, or -1 if the average size is unknown
*
*/
private Double averageDocumentSize;
/**
* @return The estimated total data size, in bytes, or -1 if the size is unknown.
*
*/
private Double dataSize;
/**
* @return The name of the database containing the collection
*
*/
private String databaseName;
/**
* @return The estimated total number of documents, or -1 if the document count is unknown
*
*/
private Double documentCount;
/**
* @return Whether the collection is a capped collection (i.e. whether it has a fixed size and acts like a circular buffer)
*
*/
private Boolean isCapped;
/**
* @return Whether the collection is system collection
*
*/
private Boolean isSystemCollection;
/**
* @return Whether the collection is a view of another collection
*
*/
private Boolean isView;
/**
* @return The unqualified name of the database or collection
*
*/
private String name;
/**
* @return The qualified name of the database or collection. For a collection, this is the database-qualified name.
*
*/
private String qualifiedName;
/**
* @return The shard key on the collection, or null if the collection is not sharded
*
*/
private @Nullable MongoDbShardKeyInfoResponse shardKey;
/**
* @return Whether the database has sharding enabled. Note that the migration task will enable sharding on the target if necessary.
*
*/
private Boolean supportsSharding;
/**
* @return The name of the collection that this is a view of, if IsView is true
*
*/
private @Nullable String viewOf;
private MongoDbCollectionInfoResponse() {}
/**
* @return The average document size, or -1 if the average size is unknown
*
*/
public Double averageDocumentSize() {
return this.averageDocumentSize;
}
/**
* @return The estimated total data size, in bytes, or -1 if the size is unknown.
*
*/
public Double dataSize() {
return this.dataSize;
}
/**
* @return The name of the database containing the collection
*
*/
public String databaseName() {
return this.databaseName;
}
/**
* @return The estimated total number of documents, or -1 if the document count is unknown
*
*/
public Double documentCount() {
return this.documentCount;
}
/**
* @return Whether the collection is a capped collection (i.e. whether it has a fixed size and acts like a circular buffer)
*
*/
public Boolean isCapped() {
return this.isCapped;
}
/**
* @return Whether the collection is system collection
*
*/
public Boolean isSystemCollection() {
return this.isSystemCollection;
}
/**
* @return Whether the collection is a view of another collection
*
*/
public Boolean isView() {
return this.isView;
}
/**
* @return The unqualified name of the database or collection
*
*/
public String name() {
return this.name;
}
/**
* @return The qualified name of the database or collection. For a collection, this is the database-qualified name.
*
*/
public String qualifiedName() {
return this.qualifiedName;
}
/**
* @return The shard key on the collection, or null if the collection is not sharded
*
*/
public Optional shardKey() {
return Optional.ofNullable(this.shardKey);
}
/**
* @return Whether the database has sharding enabled. Note that the migration task will enable sharding on the target if necessary.
*
*/
public Boolean supportsSharding() {
return this.supportsSharding;
}
/**
* @return The name of the collection that this is a view of, if IsView is true
*
*/
public Optional viewOf() {
return Optional.ofNullable(this.viewOf);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MongoDbCollectionInfoResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double averageDocumentSize;
private Double dataSize;
private String databaseName;
private Double documentCount;
private Boolean isCapped;
private Boolean isSystemCollection;
private Boolean isView;
private String name;
private String qualifiedName;
private @Nullable MongoDbShardKeyInfoResponse shardKey;
private Boolean supportsSharding;
private @Nullable String viewOf;
public Builder() {}
public Builder(MongoDbCollectionInfoResponse defaults) {
Objects.requireNonNull(defaults);
this.averageDocumentSize = defaults.averageDocumentSize;
this.dataSize = defaults.dataSize;
this.databaseName = defaults.databaseName;
this.documentCount = defaults.documentCount;
this.isCapped = defaults.isCapped;
this.isSystemCollection = defaults.isSystemCollection;
this.isView = defaults.isView;
this.name = defaults.name;
this.qualifiedName = defaults.qualifiedName;
this.shardKey = defaults.shardKey;
this.supportsSharding = defaults.supportsSharding;
this.viewOf = defaults.viewOf;
}
@CustomType.Setter
public Builder averageDocumentSize(Double averageDocumentSize) {
if (averageDocumentSize == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "averageDocumentSize");
}
this.averageDocumentSize = averageDocumentSize;
return this;
}
@CustomType.Setter
public Builder dataSize(Double dataSize) {
if (dataSize == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "dataSize");
}
this.dataSize = dataSize;
return this;
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder documentCount(Double documentCount) {
if (documentCount == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "documentCount");
}
this.documentCount = documentCount;
return this;
}
@CustomType.Setter
public Builder isCapped(Boolean isCapped) {
if (isCapped == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "isCapped");
}
this.isCapped = isCapped;
return this;
}
@CustomType.Setter
public Builder isSystemCollection(Boolean isSystemCollection) {
if (isSystemCollection == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "isSystemCollection");
}
this.isSystemCollection = isSystemCollection;
return this;
}
@CustomType.Setter
public Builder isView(Boolean isView) {
if (isView == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "isView");
}
this.isView = isView;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder qualifiedName(String qualifiedName) {
if (qualifiedName == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "qualifiedName");
}
this.qualifiedName = qualifiedName;
return this;
}
@CustomType.Setter
public Builder shardKey(@Nullable MongoDbShardKeyInfoResponse shardKey) {
this.shardKey = shardKey;
return this;
}
@CustomType.Setter
public Builder supportsSharding(Boolean supportsSharding) {
if (supportsSharding == null) {
throw new MissingRequiredPropertyException("MongoDbCollectionInfoResponse", "supportsSharding");
}
this.supportsSharding = supportsSharding;
return this;
}
@CustomType.Setter
public Builder viewOf(@Nullable String viewOf) {
this.viewOf = viewOf;
return this;
}
public MongoDbCollectionInfoResponse build() {
final var _resultValue = new MongoDbCollectionInfoResponse();
_resultValue.averageDocumentSize = averageDocumentSize;
_resultValue.dataSize = dataSize;
_resultValue.databaseName = databaseName;
_resultValue.documentCount = documentCount;
_resultValue.isCapped = isCapped;
_resultValue.isSystemCollection = isSystemCollection;
_resultValue.isView = isView;
_resultValue.name = name;
_resultValue.qualifiedName = qualifiedName;
_resultValue.shardKey = shardKey;
_resultValue.supportsSharding = supportsSharding;
_resultValue.viewOf = viewOf;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy