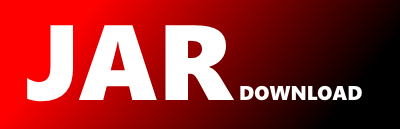
com.pulumi.azurenative.dataprotection.outputs.DatasourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.dataprotection.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DatasourceResponse {
/**
* @return DatasourceType of the resource.
*
*/
private @Nullable String datasourceType;
/**
* @return Type of Datasource object, used to initialize the right inherited type
*
*/
private @Nullable String objectType;
/**
* @return Full ARM ID of the resource. For azure resources, this is ARM ID. For non azure resources, this will be the ID created by backup service via Fabric/Vault.
*
*/
private String resourceID;
/**
* @return Location of datasource.
*
*/
private @Nullable String resourceLocation;
/**
* @return Unique identifier of the resource in the context of parent.
*
*/
private @Nullable String resourceName;
/**
* @return Resource Type of Datasource.
*
*/
private @Nullable String resourceType;
/**
* @return Uri of the resource.
*
*/
private @Nullable String resourceUri;
private DatasourceResponse() {}
/**
* @return DatasourceType of the resource.
*
*/
public Optional datasourceType() {
return Optional.ofNullable(this.datasourceType);
}
/**
* @return Type of Datasource object, used to initialize the right inherited type
*
*/
public Optional objectType() {
return Optional.ofNullable(this.objectType);
}
/**
* @return Full ARM ID of the resource. For azure resources, this is ARM ID. For non azure resources, this will be the ID created by backup service via Fabric/Vault.
*
*/
public String resourceID() {
return this.resourceID;
}
/**
* @return Location of datasource.
*
*/
public Optional resourceLocation() {
return Optional.ofNullable(this.resourceLocation);
}
/**
* @return Unique identifier of the resource in the context of parent.
*
*/
public Optional resourceName() {
return Optional.ofNullable(this.resourceName);
}
/**
* @return Resource Type of Datasource.
*
*/
public Optional resourceType() {
return Optional.ofNullable(this.resourceType);
}
/**
* @return Uri of the resource.
*
*/
public Optional resourceUri() {
return Optional.ofNullable(this.resourceUri);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DatasourceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String datasourceType;
private @Nullable String objectType;
private String resourceID;
private @Nullable String resourceLocation;
private @Nullable String resourceName;
private @Nullable String resourceType;
private @Nullable String resourceUri;
public Builder() {}
public Builder(DatasourceResponse defaults) {
Objects.requireNonNull(defaults);
this.datasourceType = defaults.datasourceType;
this.objectType = defaults.objectType;
this.resourceID = defaults.resourceID;
this.resourceLocation = defaults.resourceLocation;
this.resourceName = defaults.resourceName;
this.resourceType = defaults.resourceType;
this.resourceUri = defaults.resourceUri;
}
@CustomType.Setter
public Builder datasourceType(@Nullable String datasourceType) {
this.datasourceType = datasourceType;
return this;
}
@CustomType.Setter
public Builder objectType(@Nullable String objectType) {
this.objectType = objectType;
return this;
}
@CustomType.Setter
public Builder resourceID(String resourceID) {
if (resourceID == null) {
throw new MissingRequiredPropertyException("DatasourceResponse", "resourceID");
}
this.resourceID = resourceID;
return this;
}
@CustomType.Setter
public Builder resourceLocation(@Nullable String resourceLocation) {
this.resourceLocation = resourceLocation;
return this;
}
@CustomType.Setter
public Builder resourceName(@Nullable String resourceName) {
this.resourceName = resourceName;
return this;
}
@CustomType.Setter
public Builder resourceType(@Nullable String resourceType) {
this.resourceType = resourceType;
return this;
}
@CustomType.Setter
public Builder resourceUri(@Nullable String resourceUri) {
this.resourceUri = resourceUri;
return this;
}
public DatasourceResponse build() {
final var _resultValue = new DatasourceResponse();
_resultValue.datasourceType = datasourceType;
_resultValue.objectType = objectType;
_resultValue.resourceID = resourceID;
_resultValue.resourceLocation = resourceLocation;
_resultValue.resourceName = resourceName;
_resultValue.resourceType = resourceType;
_resultValue.resourceUri = resourceUri;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy