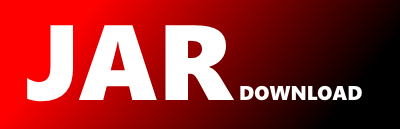
com.pulumi.azurenative.datareplication.outputs.HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datareplication.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse {
/**
* @return Gets or sets the Uri of ASR.
*
*/
private String asrServiceUri;
/**
* @return Gets or sets the ARM Id of the target AzStackHCI fabric.
*
*/
private String azStackHciFabricArmId;
/**
* @return Gets or sets the ARM Id of the AzStackHCI site.
*
*/
private String azStackHciSiteId;
/**
* @return Gets or sets the Uri of Gateway.
*
*/
private String gatewayServiceUri;
/**
* @return Gets or sets the ARM Id of the source HyperV fabric.
*
*/
private String hyperVFabricArmId;
/**
* @return Gets or sets the ARM Id of the HyperV site.
*
*/
private String hyperVSiteId;
/**
* @return Gets or sets the instance type.
* Expected value is 'HyperVToAzStackHCI'.
*
*/
private String instanceType;
/**
* @return Gets or sets the Uri of Rcm.
*
*/
private String rcmServiceUri;
/**
* @return Gets or sets the resource group.
*
*/
private String resourceGroup;
/**
* @return Gets or sets the resource location.
*
*/
private String resourceLocation;
/**
* @return Gets or sets the gateway service Id of source.
*
*/
private String sourceGatewayServiceId;
/**
* @return Gets or sets the source storage container name.
*
*/
private String sourceStorageContainerName;
/**
* @return Gets or sets the storage account Id.
*
*/
private @Nullable String storageAccountId;
/**
* @return Gets or sets the Sas Secret of storage account.
*
*/
private @Nullable String storageAccountSasSecretName;
/**
* @return Gets or sets the subscription.
*
*/
private String subscriptionId;
/**
* @return Gets or sets the gateway service Id of target.
*
*/
private String targetGatewayServiceId;
/**
* @return Gets or sets the target storage container name.
*
*/
private String targetStorageContainerName;
private HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse() {}
/**
* @return Gets or sets the Uri of ASR.
*
*/
public String asrServiceUri() {
return this.asrServiceUri;
}
/**
* @return Gets or sets the ARM Id of the target AzStackHCI fabric.
*
*/
public String azStackHciFabricArmId() {
return this.azStackHciFabricArmId;
}
/**
* @return Gets or sets the ARM Id of the AzStackHCI site.
*
*/
public String azStackHciSiteId() {
return this.azStackHciSiteId;
}
/**
* @return Gets or sets the Uri of Gateway.
*
*/
public String gatewayServiceUri() {
return this.gatewayServiceUri;
}
/**
* @return Gets or sets the ARM Id of the source HyperV fabric.
*
*/
public String hyperVFabricArmId() {
return this.hyperVFabricArmId;
}
/**
* @return Gets or sets the ARM Id of the HyperV site.
*
*/
public String hyperVSiteId() {
return this.hyperVSiteId;
}
/**
* @return Gets or sets the instance type.
* Expected value is 'HyperVToAzStackHCI'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return Gets or sets the Uri of Rcm.
*
*/
public String rcmServiceUri() {
return this.rcmServiceUri;
}
/**
* @return Gets or sets the resource group.
*
*/
public String resourceGroup() {
return this.resourceGroup;
}
/**
* @return Gets or sets the resource location.
*
*/
public String resourceLocation() {
return this.resourceLocation;
}
/**
* @return Gets or sets the gateway service Id of source.
*
*/
public String sourceGatewayServiceId() {
return this.sourceGatewayServiceId;
}
/**
* @return Gets or sets the source storage container name.
*
*/
public String sourceStorageContainerName() {
return this.sourceStorageContainerName;
}
/**
* @return Gets or sets the storage account Id.
*
*/
public Optional storageAccountId() {
return Optional.ofNullable(this.storageAccountId);
}
/**
* @return Gets or sets the Sas Secret of storage account.
*
*/
public Optional storageAccountSasSecretName() {
return Optional.ofNullable(this.storageAccountSasSecretName);
}
/**
* @return Gets or sets the subscription.
*
*/
public String subscriptionId() {
return this.subscriptionId;
}
/**
* @return Gets or sets the gateway service Id of target.
*
*/
public String targetGatewayServiceId() {
return this.targetGatewayServiceId;
}
/**
* @return Gets or sets the target storage container name.
*
*/
public String targetStorageContainerName() {
return this.targetStorageContainerName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String asrServiceUri;
private String azStackHciFabricArmId;
private String azStackHciSiteId;
private String gatewayServiceUri;
private String hyperVFabricArmId;
private String hyperVSiteId;
private String instanceType;
private String rcmServiceUri;
private String resourceGroup;
private String resourceLocation;
private String sourceGatewayServiceId;
private String sourceStorageContainerName;
private @Nullable String storageAccountId;
private @Nullable String storageAccountSasSecretName;
private String subscriptionId;
private String targetGatewayServiceId;
private String targetStorageContainerName;
public Builder() {}
public Builder(HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.asrServiceUri = defaults.asrServiceUri;
this.azStackHciFabricArmId = defaults.azStackHciFabricArmId;
this.azStackHciSiteId = defaults.azStackHciSiteId;
this.gatewayServiceUri = defaults.gatewayServiceUri;
this.hyperVFabricArmId = defaults.hyperVFabricArmId;
this.hyperVSiteId = defaults.hyperVSiteId;
this.instanceType = defaults.instanceType;
this.rcmServiceUri = defaults.rcmServiceUri;
this.resourceGroup = defaults.resourceGroup;
this.resourceLocation = defaults.resourceLocation;
this.sourceGatewayServiceId = defaults.sourceGatewayServiceId;
this.sourceStorageContainerName = defaults.sourceStorageContainerName;
this.storageAccountId = defaults.storageAccountId;
this.storageAccountSasSecretName = defaults.storageAccountSasSecretName;
this.subscriptionId = defaults.subscriptionId;
this.targetGatewayServiceId = defaults.targetGatewayServiceId;
this.targetStorageContainerName = defaults.targetStorageContainerName;
}
@CustomType.Setter
public Builder asrServiceUri(String asrServiceUri) {
if (asrServiceUri == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "asrServiceUri");
}
this.asrServiceUri = asrServiceUri;
return this;
}
@CustomType.Setter
public Builder azStackHciFabricArmId(String azStackHciFabricArmId) {
if (azStackHciFabricArmId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "azStackHciFabricArmId");
}
this.azStackHciFabricArmId = azStackHciFabricArmId;
return this;
}
@CustomType.Setter
public Builder azStackHciSiteId(String azStackHciSiteId) {
if (azStackHciSiteId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "azStackHciSiteId");
}
this.azStackHciSiteId = azStackHciSiteId;
return this;
}
@CustomType.Setter
public Builder gatewayServiceUri(String gatewayServiceUri) {
if (gatewayServiceUri == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "gatewayServiceUri");
}
this.gatewayServiceUri = gatewayServiceUri;
return this;
}
@CustomType.Setter
public Builder hyperVFabricArmId(String hyperVFabricArmId) {
if (hyperVFabricArmId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "hyperVFabricArmId");
}
this.hyperVFabricArmId = hyperVFabricArmId;
return this;
}
@CustomType.Setter
public Builder hyperVSiteId(String hyperVSiteId) {
if (hyperVSiteId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "hyperVSiteId");
}
this.hyperVSiteId = hyperVSiteId;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder rcmServiceUri(String rcmServiceUri) {
if (rcmServiceUri == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "rcmServiceUri");
}
this.rcmServiceUri = rcmServiceUri;
return this;
}
@CustomType.Setter
public Builder resourceGroup(String resourceGroup) {
if (resourceGroup == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "resourceGroup");
}
this.resourceGroup = resourceGroup;
return this;
}
@CustomType.Setter
public Builder resourceLocation(String resourceLocation) {
if (resourceLocation == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "resourceLocation");
}
this.resourceLocation = resourceLocation;
return this;
}
@CustomType.Setter
public Builder sourceGatewayServiceId(String sourceGatewayServiceId) {
if (sourceGatewayServiceId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "sourceGatewayServiceId");
}
this.sourceGatewayServiceId = sourceGatewayServiceId;
return this;
}
@CustomType.Setter
public Builder sourceStorageContainerName(String sourceStorageContainerName) {
if (sourceStorageContainerName == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "sourceStorageContainerName");
}
this.sourceStorageContainerName = sourceStorageContainerName;
return this;
}
@CustomType.Setter
public Builder storageAccountId(@Nullable String storageAccountId) {
this.storageAccountId = storageAccountId;
return this;
}
@CustomType.Setter
public Builder storageAccountSasSecretName(@Nullable String storageAccountSasSecretName) {
this.storageAccountSasSecretName = storageAccountSasSecretName;
return this;
}
@CustomType.Setter
public Builder subscriptionId(String subscriptionId) {
if (subscriptionId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "subscriptionId");
}
this.subscriptionId = subscriptionId;
return this;
}
@CustomType.Setter
public Builder targetGatewayServiceId(String targetGatewayServiceId) {
if (targetGatewayServiceId == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "targetGatewayServiceId");
}
this.targetGatewayServiceId = targetGatewayServiceId;
return this;
}
@CustomType.Setter
public Builder targetStorageContainerName(String targetStorageContainerName) {
if (targetStorageContainerName == null) {
throw new MissingRequiredPropertyException("HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse", "targetStorageContainerName");
}
this.targetStorageContainerName = targetStorageContainerName;
return this;
}
public HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse build() {
final var _resultValue = new HyperVToAzStackHCIReplicationExtensionModelCustomPropertiesResponse();
_resultValue.asrServiceUri = asrServiceUri;
_resultValue.azStackHciFabricArmId = azStackHciFabricArmId;
_resultValue.azStackHciSiteId = azStackHciSiteId;
_resultValue.gatewayServiceUri = gatewayServiceUri;
_resultValue.hyperVFabricArmId = hyperVFabricArmId;
_resultValue.hyperVSiteId = hyperVSiteId;
_resultValue.instanceType = instanceType;
_resultValue.rcmServiceUri = rcmServiceUri;
_resultValue.resourceGroup = resourceGroup;
_resultValue.resourceLocation = resourceLocation;
_resultValue.sourceGatewayServiceId = sourceGatewayServiceId;
_resultValue.sourceStorageContainerName = sourceStorageContainerName;
_resultValue.storageAccountId = storageAccountId;
_resultValue.storageAccountSasSecretName = storageAccountSasSecretName;
_resultValue.subscriptionId = subscriptionId;
_resultValue.targetGatewayServiceId = targetGatewayServiceId;
_resultValue.targetStorageContainerName = targetStorageContainerName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy