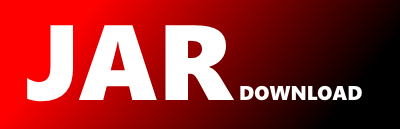
com.pulumi.azurenative.datareplication.outputs.VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datareplication.outputs;
import com.pulumi.azurenative.datareplication.outputs.ProtectedItemDynamicMemoryConfigResponse;
import com.pulumi.azurenative.datareplication.outputs.VMwareToAzStackHCIDiskInputResponse;
import com.pulumi.azurenative.datareplication.outputs.VMwareToAzStackHCINicInputResponse;
import com.pulumi.azurenative.datareplication.outputs.VMwareToAzStackHCIProtectedDiskPropertiesResponse;
import com.pulumi.azurenative.datareplication.outputs.VMwareToAzStackHCIProtectedNicPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse {
/**
* @return Gets or sets the location of the protected item.
*
*/
private String activeLocation;
/**
* @return Gets or sets the location of Azure Arc HCI custom location resource.
*
*/
private String customLocationRegion;
/**
* @return Gets or sets the list of disks to replicate.
*
*/
private List disksToInclude;
/**
* @return Protected item dynamic memory config.
*
*/
private @Nullable ProtectedItemDynamicMemoryConfigResponse dynamicMemoryConfig;
/**
* @return Gets or sets the ARM Id of the discovered machine.
*
*/
private String fabricDiscoveryMachineId;
/**
* @return Gets or sets the recovery point Id to which the VM was failed over.
*
*/
private String failoverRecoveryPointId;
/**
* @return Gets or sets the firmware type.
*
*/
private String firmwareType;
/**
* @return Gets or sets the hypervisor generation of the virtual machine possible values are 1,2.
*
*/
private String hyperVGeneration;
/**
* @return Gets or sets the initial replication progress percentage. This is calculated based on
* total bytes processed for all disks in the source VM.
*
*/
private Integer initialReplicationProgressPercentage;
/**
* @return Gets or sets the instance type.
* Expected value is 'VMwareToAzStackHCI'.
*
*/
private String instanceType;
/**
* @return Gets or sets a value indicating whether memory is dynamical.
*
*/
private @Nullable Boolean isDynamicRam;
/**
* @return Gets or sets the last recovery point Id.
*
*/
private String lastRecoveryPointId;
/**
* @return Gets or sets the last recovery point received time.
*
*/
private String lastRecoveryPointReceived;
/**
* @return Gets or sets the latest timestamp that replication status is updated.
*
*/
private String lastReplicationUpdateTime;
/**
* @return Gets or sets the migration progress percentage.
*
*/
private Integer migrationProgressPercentage;
/**
* @return Gets or sets the list of VM NIC to replicate.
*
*/
private List nicsToInclude;
/**
* @return Gets or sets the name of the OS.
*
*/
private String osName;
/**
* @return Gets or sets the type of the OS.
*
*/
private String osType;
/**
* @return Gets or sets a value indicating whether auto resync is to be done.
*
*/
private @Nullable Boolean performAutoResync;
/**
* @return Gets or sets the list of protected disks.
*
*/
private List protectedDisks;
/**
* @return Gets or sets the VM NIC details.
*
*/
private List protectedNics;
/**
* @return Gets or sets the resume progress percentage.
*
*/
private Integer resumeProgressPercentage;
/**
* @return Gets or sets the resume retry count.
*
*/
private Double resumeRetryCount;
/**
* @return Gets or sets the resync progress percentage. This is calculated based on total bytes
* processed for all disks in the source VM.
*
*/
private Integer resyncProgressPercentage;
/**
* @return Gets or sets a value indicating whether resync is required.
*
*/
private Boolean resyncRequired;
/**
* @return Gets or sets the resync retry count.
*
*/
private Double resyncRetryCount;
/**
* @return Gets or sets the resync state.
*
*/
private String resyncState;
/**
* @return Gets or sets the run as account Id.
*
*/
private String runAsAccountId;
/**
* @return Gets or sets the source appliance name.
*
*/
private String sourceApplianceName;
/**
* @return Gets or sets the source VM CPU cores.
*
*/
private Integer sourceCpuCores;
/**
* @return Gets or sets the source DRA name.
*
*/
private String sourceDraName;
/**
* @return Gets or sets the source VM ram memory size in megabytes.
*
*/
private Double sourceMemoryInMegaBytes;
/**
* @return Gets or sets the source VM display name.
*
*/
private String sourceVmName;
/**
* @return Gets or sets the target storage container ARM Id.
*
*/
private String storageContainerId;
/**
* @return Gets or sets the target appliance name.
*
*/
private String targetApplianceName;
/**
* @return Gets or sets the Target Arc Cluster Custom Location ARM Id.
*
*/
private String targetArcClusterCustomLocationId;
/**
* @return Gets or sets the Target AzStackHCI cluster name.
*
*/
private String targetAzStackHciClusterName;
/**
* @return Gets or sets the target CPU cores.
*
*/
private @Nullable Integer targetCpuCores;
/**
* @return Gets or sets the target DRA name.
*
*/
private String targetDraName;
/**
* @return Gets or sets the Target HCI Cluster ARM Id.
*
*/
private String targetHciClusterId;
/**
* @return Gets or sets the target location.
*
*/
private String targetLocation;
/**
* @return Gets or sets the target memory in mega-bytes.
*
*/
private @Nullable Integer targetMemoryInMegaBytes;
/**
* @return Gets or sets the target network Id within AzStackHCI Cluster.
*
*/
private @Nullable String targetNetworkId;
/**
* @return Gets or sets the target resource group ARM Id.
*
*/
private String targetResourceGroupId;
/**
* @return Gets or sets the BIOS Id of the target AzStackHCI VM.
*
*/
private String targetVmBiosId;
/**
* @return Gets or sets the target VM display name.
*
*/
private @Nullable String targetVmName;
/**
* @return Gets or sets the target test network Id within AzStackHCI Cluster.
*
*/
private @Nullable String testNetworkId;
private VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse() {}
/**
* @return Gets or sets the location of the protected item.
*
*/
public String activeLocation() {
return this.activeLocation;
}
/**
* @return Gets or sets the location of Azure Arc HCI custom location resource.
*
*/
public String customLocationRegion() {
return this.customLocationRegion;
}
/**
* @return Gets or sets the list of disks to replicate.
*
*/
public List disksToInclude() {
return this.disksToInclude;
}
/**
* @return Protected item dynamic memory config.
*
*/
public Optional dynamicMemoryConfig() {
return Optional.ofNullable(this.dynamicMemoryConfig);
}
/**
* @return Gets or sets the ARM Id of the discovered machine.
*
*/
public String fabricDiscoveryMachineId() {
return this.fabricDiscoveryMachineId;
}
/**
* @return Gets or sets the recovery point Id to which the VM was failed over.
*
*/
public String failoverRecoveryPointId() {
return this.failoverRecoveryPointId;
}
/**
* @return Gets or sets the firmware type.
*
*/
public String firmwareType() {
return this.firmwareType;
}
/**
* @return Gets or sets the hypervisor generation of the virtual machine possible values are 1,2.
*
*/
public String hyperVGeneration() {
return this.hyperVGeneration;
}
/**
* @return Gets or sets the initial replication progress percentage. This is calculated based on
* total bytes processed for all disks in the source VM.
*
*/
public Integer initialReplicationProgressPercentage() {
return this.initialReplicationProgressPercentage;
}
/**
* @return Gets or sets the instance type.
* Expected value is 'VMwareToAzStackHCI'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return Gets or sets a value indicating whether memory is dynamical.
*
*/
public Optional isDynamicRam() {
return Optional.ofNullable(this.isDynamicRam);
}
/**
* @return Gets or sets the last recovery point Id.
*
*/
public String lastRecoveryPointId() {
return this.lastRecoveryPointId;
}
/**
* @return Gets or sets the last recovery point received time.
*
*/
public String lastRecoveryPointReceived() {
return this.lastRecoveryPointReceived;
}
/**
* @return Gets or sets the latest timestamp that replication status is updated.
*
*/
public String lastReplicationUpdateTime() {
return this.lastReplicationUpdateTime;
}
/**
* @return Gets or sets the migration progress percentage.
*
*/
public Integer migrationProgressPercentage() {
return this.migrationProgressPercentage;
}
/**
* @return Gets or sets the list of VM NIC to replicate.
*
*/
public List nicsToInclude() {
return this.nicsToInclude;
}
/**
* @return Gets or sets the name of the OS.
*
*/
public String osName() {
return this.osName;
}
/**
* @return Gets or sets the type of the OS.
*
*/
public String osType() {
return this.osType;
}
/**
* @return Gets or sets a value indicating whether auto resync is to be done.
*
*/
public Optional performAutoResync() {
return Optional.ofNullable(this.performAutoResync);
}
/**
* @return Gets or sets the list of protected disks.
*
*/
public List protectedDisks() {
return this.protectedDisks;
}
/**
* @return Gets or sets the VM NIC details.
*
*/
public List protectedNics() {
return this.protectedNics;
}
/**
* @return Gets or sets the resume progress percentage.
*
*/
public Integer resumeProgressPercentage() {
return this.resumeProgressPercentage;
}
/**
* @return Gets or sets the resume retry count.
*
*/
public Double resumeRetryCount() {
return this.resumeRetryCount;
}
/**
* @return Gets or sets the resync progress percentage. This is calculated based on total bytes
* processed for all disks in the source VM.
*
*/
public Integer resyncProgressPercentage() {
return this.resyncProgressPercentage;
}
/**
* @return Gets or sets a value indicating whether resync is required.
*
*/
public Boolean resyncRequired() {
return this.resyncRequired;
}
/**
* @return Gets or sets the resync retry count.
*
*/
public Double resyncRetryCount() {
return this.resyncRetryCount;
}
/**
* @return Gets or sets the resync state.
*
*/
public String resyncState() {
return this.resyncState;
}
/**
* @return Gets or sets the run as account Id.
*
*/
public String runAsAccountId() {
return this.runAsAccountId;
}
/**
* @return Gets or sets the source appliance name.
*
*/
public String sourceApplianceName() {
return this.sourceApplianceName;
}
/**
* @return Gets or sets the source VM CPU cores.
*
*/
public Integer sourceCpuCores() {
return this.sourceCpuCores;
}
/**
* @return Gets or sets the source DRA name.
*
*/
public String sourceDraName() {
return this.sourceDraName;
}
/**
* @return Gets or sets the source VM ram memory size in megabytes.
*
*/
public Double sourceMemoryInMegaBytes() {
return this.sourceMemoryInMegaBytes;
}
/**
* @return Gets or sets the source VM display name.
*
*/
public String sourceVmName() {
return this.sourceVmName;
}
/**
* @return Gets or sets the target storage container ARM Id.
*
*/
public String storageContainerId() {
return this.storageContainerId;
}
/**
* @return Gets or sets the target appliance name.
*
*/
public String targetApplianceName() {
return this.targetApplianceName;
}
/**
* @return Gets or sets the Target Arc Cluster Custom Location ARM Id.
*
*/
public String targetArcClusterCustomLocationId() {
return this.targetArcClusterCustomLocationId;
}
/**
* @return Gets or sets the Target AzStackHCI cluster name.
*
*/
public String targetAzStackHciClusterName() {
return this.targetAzStackHciClusterName;
}
/**
* @return Gets or sets the target CPU cores.
*
*/
public Optional targetCpuCores() {
return Optional.ofNullable(this.targetCpuCores);
}
/**
* @return Gets or sets the target DRA name.
*
*/
public String targetDraName() {
return this.targetDraName;
}
/**
* @return Gets or sets the Target HCI Cluster ARM Id.
*
*/
public String targetHciClusterId() {
return this.targetHciClusterId;
}
/**
* @return Gets or sets the target location.
*
*/
public String targetLocation() {
return this.targetLocation;
}
/**
* @return Gets or sets the target memory in mega-bytes.
*
*/
public Optional targetMemoryInMegaBytes() {
return Optional.ofNullable(this.targetMemoryInMegaBytes);
}
/**
* @return Gets or sets the target network Id within AzStackHCI Cluster.
*
*/
public Optional targetNetworkId() {
return Optional.ofNullable(this.targetNetworkId);
}
/**
* @return Gets or sets the target resource group ARM Id.
*
*/
public String targetResourceGroupId() {
return this.targetResourceGroupId;
}
/**
* @return Gets or sets the BIOS Id of the target AzStackHCI VM.
*
*/
public String targetVmBiosId() {
return this.targetVmBiosId;
}
/**
* @return Gets or sets the target VM display name.
*
*/
public Optional targetVmName() {
return Optional.ofNullable(this.targetVmName);
}
/**
* @return Gets or sets the target test network Id within AzStackHCI Cluster.
*
*/
public Optional testNetworkId() {
return Optional.ofNullable(this.testNetworkId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String activeLocation;
private String customLocationRegion;
private List disksToInclude;
private @Nullable ProtectedItemDynamicMemoryConfigResponse dynamicMemoryConfig;
private String fabricDiscoveryMachineId;
private String failoverRecoveryPointId;
private String firmwareType;
private String hyperVGeneration;
private Integer initialReplicationProgressPercentage;
private String instanceType;
private @Nullable Boolean isDynamicRam;
private String lastRecoveryPointId;
private String lastRecoveryPointReceived;
private String lastReplicationUpdateTime;
private Integer migrationProgressPercentage;
private List nicsToInclude;
private String osName;
private String osType;
private @Nullable Boolean performAutoResync;
private List protectedDisks;
private List protectedNics;
private Integer resumeProgressPercentage;
private Double resumeRetryCount;
private Integer resyncProgressPercentage;
private Boolean resyncRequired;
private Double resyncRetryCount;
private String resyncState;
private String runAsAccountId;
private String sourceApplianceName;
private Integer sourceCpuCores;
private String sourceDraName;
private Double sourceMemoryInMegaBytes;
private String sourceVmName;
private String storageContainerId;
private String targetApplianceName;
private String targetArcClusterCustomLocationId;
private String targetAzStackHciClusterName;
private @Nullable Integer targetCpuCores;
private String targetDraName;
private String targetHciClusterId;
private String targetLocation;
private @Nullable Integer targetMemoryInMegaBytes;
private @Nullable String targetNetworkId;
private String targetResourceGroupId;
private String targetVmBiosId;
private @Nullable String targetVmName;
private @Nullable String testNetworkId;
public Builder() {}
public Builder(VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.activeLocation = defaults.activeLocation;
this.customLocationRegion = defaults.customLocationRegion;
this.disksToInclude = defaults.disksToInclude;
this.dynamicMemoryConfig = defaults.dynamicMemoryConfig;
this.fabricDiscoveryMachineId = defaults.fabricDiscoveryMachineId;
this.failoverRecoveryPointId = defaults.failoverRecoveryPointId;
this.firmwareType = defaults.firmwareType;
this.hyperVGeneration = defaults.hyperVGeneration;
this.initialReplicationProgressPercentage = defaults.initialReplicationProgressPercentage;
this.instanceType = defaults.instanceType;
this.isDynamicRam = defaults.isDynamicRam;
this.lastRecoveryPointId = defaults.lastRecoveryPointId;
this.lastRecoveryPointReceived = defaults.lastRecoveryPointReceived;
this.lastReplicationUpdateTime = defaults.lastReplicationUpdateTime;
this.migrationProgressPercentage = defaults.migrationProgressPercentage;
this.nicsToInclude = defaults.nicsToInclude;
this.osName = defaults.osName;
this.osType = defaults.osType;
this.performAutoResync = defaults.performAutoResync;
this.protectedDisks = defaults.protectedDisks;
this.protectedNics = defaults.protectedNics;
this.resumeProgressPercentage = defaults.resumeProgressPercentage;
this.resumeRetryCount = defaults.resumeRetryCount;
this.resyncProgressPercentage = defaults.resyncProgressPercentage;
this.resyncRequired = defaults.resyncRequired;
this.resyncRetryCount = defaults.resyncRetryCount;
this.resyncState = defaults.resyncState;
this.runAsAccountId = defaults.runAsAccountId;
this.sourceApplianceName = defaults.sourceApplianceName;
this.sourceCpuCores = defaults.sourceCpuCores;
this.sourceDraName = defaults.sourceDraName;
this.sourceMemoryInMegaBytes = defaults.sourceMemoryInMegaBytes;
this.sourceVmName = defaults.sourceVmName;
this.storageContainerId = defaults.storageContainerId;
this.targetApplianceName = defaults.targetApplianceName;
this.targetArcClusterCustomLocationId = defaults.targetArcClusterCustomLocationId;
this.targetAzStackHciClusterName = defaults.targetAzStackHciClusterName;
this.targetCpuCores = defaults.targetCpuCores;
this.targetDraName = defaults.targetDraName;
this.targetHciClusterId = defaults.targetHciClusterId;
this.targetLocation = defaults.targetLocation;
this.targetMemoryInMegaBytes = defaults.targetMemoryInMegaBytes;
this.targetNetworkId = defaults.targetNetworkId;
this.targetResourceGroupId = defaults.targetResourceGroupId;
this.targetVmBiosId = defaults.targetVmBiosId;
this.targetVmName = defaults.targetVmName;
this.testNetworkId = defaults.testNetworkId;
}
@CustomType.Setter
public Builder activeLocation(String activeLocation) {
if (activeLocation == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "activeLocation");
}
this.activeLocation = activeLocation;
return this;
}
@CustomType.Setter
public Builder customLocationRegion(String customLocationRegion) {
if (customLocationRegion == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "customLocationRegion");
}
this.customLocationRegion = customLocationRegion;
return this;
}
@CustomType.Setter
public Builder disksToInclude(List disksToInclude) {
if (disksToInclude == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "disksToInclude");
}
this.disksToInclude = disksToInclude;
return this;
}
public Builder disksToInclude(VMwareToAzStackHCIDiskInputResponse... disksToInclude) {
return disksToInclude(List.of(disksToInclude));
}
@CustomType.Setter
public Builder dynamicMemoryConfig(@Nullable ProtectedItemDynamicMemoryConfigResponse dynamicMemoryConfig) {
this.dynamicMemoryConfig = dynamicMemoryConfig;
return this;
}
@CustomType.Setter
public Builder fabricDiscoveryMachineId(String fabricDiscoveryMachineId) {
if (fabricDiscoveryMachineId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "fabricDiscoveryMachineId");
}
this.fabricDiscoveryMachineId = fabricDiscoveryMachineId;
return this;
}
@CustomType.Setter
public Builder failoverRecoveryPointId(String failoverRecoveryPointId) {
if (failoverRecoveryPointId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "failoverRecoveryPointId");
}
this.failoverRecoveryPointId = failoverRecoveryPointId;
return this;
}
@CustomType.Setter
public Builder firmwareType(String firmwareType) {
if (firmwareType == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "firmwareType");
}
this.firmwareType = firmwareType;
return this;
}
@CustomType.Setter
public Builder hyperVGeneration(String hyperVGeneration) {
if (hyperVGeneration == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "hyperVGeneration");
}
this.hyperVGeneration = hyperVGeneration;
return this;
}
@CustomType.Setter
public Builder initialReplicationProgressPercentage(Integer initialReplicationProgressPercentage) {
if (initialReplicationProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "initialReplicationProgressPercentage");
}
this.initialReplicationProgressPercentage = initialReplicationProgressPercentage;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder isDynamicRam(@Nullable Boolean isDynamicRam) {
this.isDynamicRam = isDynamicRam;
return this;
}
@CustomType.Setter
public Builder lastRecoveryPointId(String lastRecoveryPointId) {
if (lastRecoveryPointId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "lastRecoveryPointId");
}
this.lastRecoveryPointId = lastRecoveryPointId;
return this;
}
@CustomType.Setter
public Builder lastRecoveryPointReceived(String lastRecoveryPointReceived) {
if (lastRecoveryPointReceived == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "lastRecoveryPointReceived");
}
this.lastRecoveryPointReceived = lastRecoveryPointReceived;
return this;
}
@CustomType.Setter
public Builder lastReplicationUpdateTime(String lastReplicationUpdateTime) {
if (lastReplicationUpdateTime == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "lastReplicationUpdateTime");
}
this.lastReplicationUpdateTime = lastReplicationUpdateTime;
return this;
}
@CustomType.Setter
public Builder migrationProgressPercentage(Integer migrationProgressPercentage) {
if (migrationProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "migrationProgressPercentage");
}
this.migrationProgressPercentage = migrationProgressPercentage;
return this;
}
@CustomType.Setter
public Builder nicsToInclude(List nicsToInclude) {
if (nicsToInclude == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "nicsToInclude");
}
this.nicsToInclude = nicsToInclude;
return this;
}
public Builder nicsToInclude(VMwareToAzStackHCINicInputResponse... nicsToInclude) {
return nicsToInclude(List.of(nicsToInclude));
}
@CustomType.Setter
public Builder osName(String osName) {
if (osName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "osName");
}
this.osName = osName;
return this;
}
@CustomType.Setter
public Builder osType(String osType) {
if (osType == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "osType");
}
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder performAutoResync(@Nullable Boolean performAutoResync) {
this.performAutoResync = performAutoResync;
return this;
}
@CustomType.Setter
public Builder protectedDisks(List protectedDisks) {
if (protectedDisks == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "protectedDisks");
}
this.protectedDisks = protectedDisks;
return this;
}
public Builder protectedDisks(VMwareToAzStackHCIProtectedDiskPropertiesResponse... protectedDisks) {
return protectedDisks(List.of(protectedDisks));
}
@CustomType.Setter
public Builder protectedNics(List protectedNics) {
if (protectedNics == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "protectedNics");
}
this.protectedNics = protectedNics;
return this;
}
public Builder protectedNics(VMwareToAzStackHCIProtectedNicPropertiesResponse... protectedNics) {
return protectedNics(List.of(protectedNics));
}
@CustomType.Setter
public Builder resumeProgressPercentage(Integer resumeProgressPercentage) {
if (resumeProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "resumeProgressPercentage");
}
this.resumeProgressPercentage = resumeProgressPercentage;
return this;
}
@CustomType.Setter
public Builder resumeRetryCount(Double resumeRetryCount) {
if (resumeRetryCount == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "resumeRetryCount");
}
this.resumeRetryCount = resumeRetryCount;
return this;
}
@CustomType.Setter
public Builder resyncProgressPercentage(Integer resyncProgressPercentage) {
if (resyncProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "resyncProgressPercentage");
}
this.resyncProgressPercentage = resyncProgressPercentage;
return this;
}
@CustomType.Setter
public Builder resyncRequired(Boolean resyncRequired) {
if (resyncRequired == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "resyncRequired");
}
this.resyncRequired = resyncRequired;
return this;
}
@CustomType.Setter
public Builder resyncRetryCount(Double resyncRetryCount) {
if (resyncRetryCount == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "resyncRetryCount");
}
this.resyncRetryCount = resyncRetryCount;
return this;
}
@CustomType.Setter
public Builder resyncState(String resyncState) {
if (resyncState == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "resyncState");
}
this.resyncState = resyncState;
return this;
}
@CustomType.Setter
public Builder runAsAccountId(String runAsAccountId) {
if (runAsAccountId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "runAsAccountId");
}
this.runAsAccountId = runAsAccountId;
return this;
}
@CustomType.Setter
public Builder sourceApplianceName(String sourceApplianceName) {
if (sourceApplianceName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "sourceApplianceName");
}
this.sourceApplianceName = sourceApplianceName;
return this;
}
@CustomType.Setter
public Builder sourceCpuCores(Integer sourceCpuCores) {
if (sourceCpuCores == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "sourceCpuCores");
}
this.sourceCpuCores = sourceCpuCores;
return this;
}
@CustomType.Setter
public Builder sourceDraName(String sourceDraName) {
if (sourceDraName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "sourceDraName");
}
this.sourceDraName = sourceDraName;
return this;
}
@CustomType.Setter
public Builder sourceMemoryInMegaBytes(Double sourceMemoryInMegaBytes) {
if (sourceMemoryInMegaBytes == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "sourceMemoryInMegaBytes");
}
this.sourceMemoryInMegaBytes = sourceMemoryInMegaBytes;
return this;
}
@CustomType.Setter
public Builder sourceVmName(String sourceVmName) {
if (sourceVmName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "sourceVmName");
}
this.sourceVmName = sourceVmName;
return this;
}
@CustomType.Setter
public Builder storageContainerId(String storageContainerId) {
if (storageContainerId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "storageContainerId");
}
this.storageContainerId = storageContainerId;
return this;
}
@CustomType.Setter
public Builder targetApplianceName(String targetApplianceName) {
if (targetApplianceName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetApplianceName");
}
this.targetApplianceName = targetApplianceName;
return this;
}
@CustomType.Setter
public Builder targetArcClusterCustomLocationId(String targetArcClusterCustomLocationId) {
if (targetArcClusterCustomLocationId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetArcClusterCustomLocationId");
}
this.targetArcClusterCustomLocationId = targetArcClusterCustomLocationId;
return this;
}
@CustomType.Setter
public Builder targetAzStackHciClusterName(String targetAzStackHciClusterName) {
if (targetAzStackHciClusterName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetAzStackHciClusterName");
}
this.targetAzStackHciClusterName = targetAzStackHciClusterName;
return this;
}
@CustomType.Setter
public Builder targetCpuCores(@Nullable Integer targetCpuCores) {
this.targetCpuCores = targetCpuCores;
return this;
}
@CustomType.Setter
public Builder targetDraName(String targetDraName) {
if (targetDraName == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetDraName");
}
this.targetDraName = targetDraName;
return this;
}
@CustomType.Setter
public Builder targetHciClusterId(String targetHciClusterId) {
if (targetHciClusterId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetHciClusterId");
}
this.targetHciClusterId = targetHciClusterId;
return this;
}
@CustomType.Setter
public Builder targetLocation(String targetLocation) {
if (targetLocation == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetLocation");
}
this.targetLocation = targetLocation;
return this;
}
@CustomType.Setter
public Builder targetMemoryInMegaBytes(@Nullable Integer targetMemoryInMegaBytes) {
this.targetMemoryInMegaBytes = targetMemoryInMegaBytes;
return this;
}
@CustomType.Setter
public Builder targetNetworkId(@Nullable String targetNetworkId) {
this.targetNetworkId = targetNetworkId;
return this;
}
@CustomType.Setter
public Builder targetResourceGroupId(String targetResourceGroupId) {
if (targetResourceGroupId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetResourceGroupId");
}
this.targetResourceGroupId = targetResourceGroupId;
return this;
}
@CustomType.Setter
public Builder targetVmBiosId(String targetVmBiosId) {
if (targetVmBiosId == null) {
throw new MissingRequiredPropertyException("VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse", "targetVmBiosId");
}
this.targetVmBiosId = targetVmBiosId;
return this;
}
@CustomType.Setter
public Builder targetVmName(@Nullable String targetVmName) {
this.targetVmName = targetVmName;
return this;
}
@CustomType.Setter
public Builder testNetworkId(@Nullable String testNetworkId) {
this.testNetworkId = testNetworkId;
return this;
}
public VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse build() {
final var _resultValue = new VMwareToAzStackHCIProtectedItemModelCustomPropertiesResponse();
_resultValue.activeLocation = activeLocation;
_resultValue.customLocationRegion = customLocationRegion;
_resultValue.disksToInclude = disksToInclude;
_resultValue.dynamicMemoryConfig = dynamicMemoryConfig;
_resultValue.fabricDiscoveryMachineId = fabricDiscoveryMachineId;
_resultValue.failoverRecoveryPointId = failoverRecoveryPointId;
_resultValue.firmwareType = firmwareType;
_resultValue.hyperVGeneration = hyperVGeneration;
_resultValue.initialReplicationProgressPercentage = initialReplicationProgressPercentage;
_resultValue.instanceType = instanceType;
_resultValue.isDynamicRam = isDynamicRam;
_resultValue.lastRecoveryPointId = lastRecoveryPointId;
_resultValue.lastRecoveryPointReceived = lastRecoveryPointReceived;
_resultValue.lastReplicationUpdateTime = lastReplicationUpdateTime;
_resultValue.migrationProgressPercentage = migrationProgressPercentage;
_resultValue.nicsToInclude = nicsToInclude;
_resultValue.osName = osName;
_resultValue.osType = osType;
_resultValue.performAutoResync = performAutoResync;
_resultValue.protectedDisks = protectedDisks;
_resultValue.protectedNics = protectedNics;
_resultValue.resumeProgressPercentage = resumeProgressPercentage;
_resultValue.resumeRetryCount = resumeRetryCount;
_resultValue.resyncProgressPercentage = resyncProgressPercentage;
_resultValue.resyncRequired = resyncRequired;
_resultValue.resyncRetryCount = resyncRetryCount;
_resultValue.resyncState = resyncState;
_resultValue.runAsAccountId = runAsAccountId;
_resultValue.sourceApplianceName = sourceApplianceName;
_resultValue.sourceCpuCores = sourceCpuCores;
_resultValue.sourceDraName = sourceDraName;
_resultValue.sourceMemoryInMegaBytes = sourceMemoryInMegaBytes;
_resultValue.sourceVmName = sourceVmName;
_resultValue.storageContainerId = storageContainerId;
_resultValue.targetApplianceName = targetApplianceName;
_resultValue.targetArcClusterCustomLocationId = targetArcClusterCustomLocationId;
_resultValue.targetAzStackHciClusterName = targetAzStackHciClusterName;
_resultValue.targetCpuCores = targetCpuCores;
_resultValue.targetDraName = targetDraName;
_resultValue.targetHciClusterId = targetHciClusterId;
_resultValue.targetLocation = targetLocation;
_resultValue.targetMemoryInMegaBytes = targetMemoryInMegaBytes;
_resultValue.targetNetworkId = targetNetworkId;
_resultValue.targetResourceGroupId = targetResourceGroupId;
_resultValue.targetVmBiosId = targetVmBiosId;
_resultValue.targetVmName = targetVmName;
_resultValue.testNetworkId = testNetworkId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy