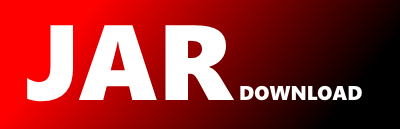
com.pulumi.azurenative.dbformariadb.outputs.GetServerResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.dbformariadb.outputs;
import com.pulumi.azurenative.dbformariadb.outputs.ServerPrivateEndpointConnectionResponse;
import com.pulumi.azurenative.dbformariadb.outputs.SkuResponse;
import com.pulumi.azurenative.dbformariadb.outputs.StorageProfileResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetServerResult {
/**
* @return The administrator's login name of a server. Can only be specified when the server is being created (and is required for creation).
*
*/
private @Nullable String administratorLogin;
/**
* @return Earliest restore point creation time (ISO8601 format)
*
*/
private @Nullable String earliestRestoreDate;
/**
* @return The fully qualified domain name of a server.
*
*/
private @Nullable String fullyQualifiedDomainName;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The master server id of a replica server.
*
*/
private @Nullable String masterServerId;
/**
* @return Enforce a minimal Tls version for the server.
*
*/
private @Nullable String minimalTlsVersion;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return List of private endpoint connections on a server
*
*/
private List privateEndpointConnections;
/**
* @return Whether or not public network access is allowed for this server. Value is optional but if passed in, must be 'Enabled' or 'Disabled'
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return The maximum number of replicas that a master server can have.
*
*/
private @Nullable Integer replicaCapacity;
/**
* @return The replication role of the server.
*
*/
private @Nullable String replicationRole;
/**
* @return The SKU (pricing tier) of the server.
*
*/
private @Nullable SkuResponse sku;
/**
* @return Enable ssl enforcement or not when connect to server.
*
*/
private @Nullable String sslEnforcement;
/**
* @return Storage profile of a server.
*
*/
private @Nullable StorageProfileResponse storageProfile;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return A state of a server that is visible to user.
*
*/
private @Nullable String userVisibleState;
/**
* @return Server version.
*
*/
private @Nullable String version;
private GetServerResult() {}
/**
* @return The administrator's login name of a server. Can only be specified when the server is being created (and is required for creation).
*
*/
public Optional administratorLogin() {
return Optional.ofNullable(this.administratorLogin);
}
/**
* @return Earliest restore point creation time (ISO8601 format)
*
*/
public Optional earliestRestoreDate() {
return Optional.ofNullable(this.earliestRestoreDate);
}
/**
* @return The fully qualified domain name of a server.
*
*/
public Optional fullyQualifiedDomainName() {
return Optional.ofNullable(this.fullyQualifiedDomainName);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The master server id of a replica server.
*
*/
public Optional masterServerId() {
return Optional.ofNullable(this.masterServerId);
}
/**
* @return Enforce a minimal Tls version for the server.
*
*/
public Optional minimalTlsVersion() {
return Optional.ofNullable(this.minimalTlsVersion);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return List of private endpoint connections on a server
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return Whether or not public network access is allowed for this server. Value is optional but if passed in, must be 'Enabled' or 'Disabled'
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return The maximum number of replicas that a master server can have.
*
*/
public Optional replicaCapacity() {
return Optional.ofNullable(this.replicaCapacity);
}
/**
* @return The replication role of the server.
*
*/
public Optional replicationRole() {
return Optional.ofNullable(this.replicationRole);
}
/**
* @return The SKU (pricing tier) of the server.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Enable ssl enforcement or not when connect to server.
*
*/
public Optional sslEnforcement() {
return Optional.ofNullable(this.sslEnforcement);
}
/**
* @return Storage profile of a server.
*
*/
public Optional storageProfile() {
return Optional.ofNullable(this.storageProfile);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return A state of a server that is visible to user.
*
*/
public Optional userVisibleState() {
return Optional.ofNullable(this.userVisibleState);
}
/**
* @return Server version.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String administratorLogin;
private @Nullable String earliestRestoreDate;
private @Nullable String fullyQualifiedDomainName;
private String id;
private String location;
private @Nullable String masterServerId;
private @Nullable String minimalTlsVersion;
private String name;
private List privateEndpointConnections;
private @Nullable String publicNetworkAccess;
private @Nullable Integer replicaCapacity;
private @Nullable String replicationRole;
private @Nullable SkuResponse sku;
private @Nullable String sslEnforcement;
private @Nullable StorageProfileResponse storageProfile;
private @Nullable Map tags;
private String type;
private @Nullable String userVisibleState;
private @Nullable String version;
public Builder() {}
public Builder(GetServerResult defaults) {
Objects.requireNonNull(defaults);
this.administratorLogin = defaults.administratorLogin;
this.earliestRestoreDate = defaults.earliestRestoreDate;
this.fullyQualifiedDomainName = defaults.fullyQualifiedDomainName;
this.id = defaults.id;
this.location = defaults.location;
this.masterServerId = defaults.masterServerId;
this.minimalTlsVersion = defaults.minimalTlsVersion;
this.name = defaults.name;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.replicaCapacity = defaults.replicaCapacity;
this.replicationRole = defaults.replicationRole;
this.sku = defaults.sku;
this.sslEnforcement = defaults.sslEnforcement;
this.storageProfile = defaults.storageProfile;
this.tags = defaults.tags;
this.type = defaults.type;
this.userVisibleState = defaults.userVisibleState;
this.version = defaults.version;
}
@CustomType.Setter
public Builder administratorLogin(@Nullable String administratorLogin) {
this.administratorLogin = administratorLogin;
return this;
}
@CustomType.Setter
public Builder earliestRestoreDate(@Nullable String earliestRestoreDate) {
this.earliestRestoreDate = earliestRestoreDate;
return this;
}
@CustomType.Setter
public Builder fullyQualifiedDomainName(@Nullable String fullyQualifiedDomainName) {
this.fullyQualifiedDomainName = fullyQualifiedDomainName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetServerResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder masterServerId(@Nullable String masterServerId) {
this.masterServerId = masterServerId;
return this;
}
@CustomType.Setter
public Builder minimalTlsVersion(@Nullable String minimalTlsVersion) {
this.minimalTlsVersion = minimalTlsVersion;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServerResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("GetServerResult", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(ServerPrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder replicaCapacity(@Nullable Integer replicaCapacity) {
this.replicaCapacity = replicaCapacity;
return this;
}
@CustomType.Setter
public Builder replicationRole(@Nullable String replicationRole) {
this.replicationRole = replicationRole;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder sslEnforcement(@Nullable String sslEnforcement) {
this.sslEnforcement = sslEnforcement;
return this;
}
@CustomType.Setter
public Builder storageProfile(@Nullable StorageProfileResponse storageProfile) {
this.storageProfile = storageProfile;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetServerResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder userVisibleState(@Nullable String userVisibleState) {
this.userVisibleState = userVisibleState;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public GetServerResult build() {
final var _resultValue = new GetServerResult();
_resultValue.administratorLogin = administratorLogin;
_resultValue.earliestRestoreDate = earliestRestoreDate;
_resultValue.fullyQualifiedDomainName = fullyQualifiedDomainName;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.masterServerId = masterServerId;
_resultValue.minimalTlsVersion = minimalTlsVersion;
_resultValue.name = name;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.replicaCapacity = replicaCapacity;
_resultValue.replicationRole = replicationRole;
_resultValue.sku = sku;
_resultValue.sslEnforcement = sslEnforcement;
_resultValue.storageProfile = storageProfile;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.userVisibleState = userVisibleState;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy