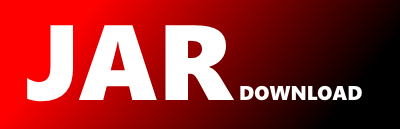
com.pulumi.azurenative.delegatednetwork.OrchestratorInstanceServiceDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.delegatednetwork;
import com.pulumi.azurenative.delegatednetwork.enums.OrchestratorKind;
import com.pulumi.azurenative.delegatednetwork.inputs.ControllerDetailsArgs;
import com.pulumi.azurenative.delegatednetwork.inputs.OrchestratorIdentityArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OrchestratorInstanceServiceDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final OrchestratorInstanceServiceDetailsArgs Empty = new OrchestratorInstanceServiceDetailsArgs();
/**
* K8s APIServer url. Either one of apiServerEndpoint or privateLinkResourceId can be specified
*
*/
@Import(name="apiServerEndpoint")
private @Nullable Output apiServerEndpoint;
/**
* @return K8s APIServer url. Either one of apiServerEndpoint or privateLinkResourceId can be specified
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy