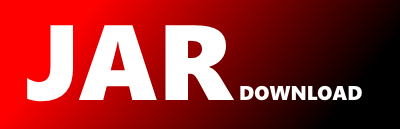
com.pulumi.azurenative.desktopvirtualization.outputs.GetHostPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.desktopvirtualization.outputs;
import com.pulumi.azurenative.desktopvirtualization.outputs.AgentUpdatePropertiesResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.RegistrationInfoResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.ResourceModelWithAllowedPropertySetResponseIdentity;
import com.pulumi.azurenative.desktopvirtualization.outputs.ResourceModelWithAllowedPropertySetResponsePlan;
import com.pulumi.azurenative.desktopvirtualization.outputs.ResourceModelWithAllowedPropertySetResponseSku;
import com.pulumi.azurenative.desktopvirtualization.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetHostPoolResult {
/**
* @return The session host configuration for updating agent, monitoring agent, and stack component.
*
*/
private @Nullable AgentUpdatePropertiesResponse agentUpdate;
/**
* @return List of applicationGroup links.
*
*/
private List applicationGroupReferences;
/**
* @return Is cloud pc resource.
*
*/
private Boolean cloudPcResource;
/**
* @return Custom rdp property of HostPool.
*
*/
private @Nullable String customRdpProperty;
/**
* @return Description of HostPool.
*
*/
private @Nullable String description;
/**
* @return The etag field is *not* required. If it is provided in the response body, it must also be provided as a header per the normal etag convention. Entity tags are used for comparing two or more entities from the same requested resource. HTTP/1.1 uses entity tags in the etag (section 14.19), If-Match (section 14.24), If-None-Match (section 14.26), and If-Range (section 14.27) header fields.
*
*/
private String etag;
/**
* @return Friendly name of HostPool.
*
*/
private @Nullable String friendlyName;
/**
* @return HostPool type for desktop.
*
*/
private String hostPoolType;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
private @Nullable ResourceModelWithAllowedPropertySetResponseIdentity identity;
/**
* @return Metadata used by portal/tooling/etc to render different UX experiences for resources of the same type; e.g. ApiApps are a kind of Microsoft.Web/sites type. If supported, the resource provider must validate and persist this value.
*
*/
private @Nullable String kind;
/**
* @return The type of the load balancer.
*
*/
private String loadBalancerType;
/**
* @return The geo-location where the resource lives
*
*/
private @Nullable String location;
/**
* @return The fully qualified resource ID of the resource that manages this resource. Indicates if this resource is managed by another Azure resource. If this is present, complete mode deployment will not delete the resource if it is removed from the template since it is managed by another resource.
*
*/
private @Nullable String managedBy;
/**
* @return The max session limit of HostPool.
*
*/
private @Nullable Integer maxSessionLimit;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return ObjectId of HostPool. (internal use)
*
*/
private String objectId;
/**
* @return PersonalDesktopAssignment type for HostPool.
*
*/
private @Nullable String personalDesktopAssignmentType;
private @Nullable ResourceModelWithAllowedPropertySetResponsePlan plan;
/**
* @return The type of preferred application group type, default to Desktop Application Group
*
*/
private String preferredAppGroupType;
/**
* @return The registration info of HostPool.
*
*/
private @Nullable RegistrationInfoResponse registrationInfo;
/**
* @return The ring number of HostPool.
*
*/
private @Nullable Integer ring;
private @Nullable ResourceModelWithAllowedPropertySetResponseSku sku;
/**
* @return ClientId for the registered Relying Party used to issue WVD SSO certificates.
*
*/
private @Nullable String ssoClientId;
/**
* @return Path to Azure KeyVault storing the secret used for communication to ADFS.
*
*/
private @Nullable String ssoClientSecretKeyVaultPath;
/**
* @return The type of single sign on Secret Type.
*
*/
private @Nullable String ssoSecretType;
/**
* @return URL to customer ADFS server for signing WVD SSO certificates.
*
*/
private @Nullable String ssoadfsAuthority;
/**
* @return The flag to turn on/off StartVMOnConnect feature.
*
*/
private @Nullable Boolean startVMOnConnect;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Is validation environment.
*
*/
private @Nullable Boolean validationEnvironment;
/**
* @return VM template for sessionhosts configuration within hostpool.
*
*/
private @Nullable String vmTemplate;
private GetHostPoolResult() {}
/**
* @return The session host configuration for updating agent, monitoring agent, and stack component.
*
*/
public Optional agentUpdate() {
return Optional.ofNullable(this.agentUpdate);
}
/**
* @return List of applicationGroup links.
*
*/
public List applicationGroupReferences() {
return this.applicationGroupReferences;
}
/**
* @return Is cloud pc resource.
*
*/
public Boolean cloudPcResource() {
return this.cloudPcResource;
}
/**
* @return Custom rdp property of HostPool.
*
*/
public Optional customRdpProperty() {
return Optional.ofNullable(this.customRdpProperty);
}
/**
* @return Description of HostPool.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The etag field is *not* required. If it is provided in the response body, it must also be provided as a header per the normal etag convention. Entity tags are used for comparing two or more entities from the same requested resource. HTTP/1.1 uses entity tags in the etag (section 14.19), If-Match (section 14.24), If-None-Match (section 14.26), and If-Range (section 14.27) header fields.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Friendly name of HostPool.
*
*/
public Optional friendlyName() {
return Optional.ofNullable(this.friendlyName);
}
/**
* @return HostPool type for desktop.
*
*/
public String hostPoolType() {
return this.hostPoolType;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return Metadata used by portal/tooling/etc to render different UX experiences for resources of the same type; e.g. ApiApps are a kind of Microsoft.Web/sites type. If supported, the resource provider must validate and persist this value.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return The type of the load balancer.
*
*/
public String loadBalancerType() {
return this.loadBalancerType;
}
/**
* @return The geo-location where the resource lives
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The fully qualified resource ID of the resource that manages this resource. Indicates if this resource is managed by another Azure resource. If this is present, complete mode deployment will not delete the resource if it is removed from the template since it is managed by another resource.
*
*/
public Optional managedBy() {
return Optional.ofNullable(this.managedBy);
}
/**
* @return The max session limit of HostPool.
*
*/
public Optional maxSessionLimit() {
return Optional.ofNullable(this.maxSessionLimit);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return ObjectId of HostPool. (internal use)
*
*/
public String objectId() {
return this.objectId;
}
/**
* @return PersonalDesktopAssignment type for HostPool.
*
*/
public Optional personalDesktopAssignmentType() {
return Optional.ofNullable(this.personalDesktopAssignmentType);
}
public Optional plan() {
return Optional.ofNullable(this.plan);
}
/**
* @return The type of preferred application group type, default to Desktop Application Group
*
*/
public String preferredAppGroupType() {
return this.preferredAppGroupType;
}
/**
* @return The registration info of HostPool.
*
*/
public Optional registrationInfo() {
return Optional.ofNullable(this.registrationInfo);
}
/**
* @return The ring number of HostPool.
*
*/
public Optional ring() {
return Optional.ofNullable(this.ring);
}
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return ClientId for the registered Relying Party used to issue WVD SSO certificates.
*
*/
public Optional ssoClientId() {
return Optional.ofNullable(this.ssoClientId);
}
/**
* @return Path to Azure KeyVault storing the secret used for communication to ADFS.
*
*/
public Optional ssoClientSecretKeyVaultPath() {
return Optional.ofNullable(this.ssoClientSecretKeyVaultPath);
}
/**
* @return The type of single sign on Secret Type.
*
*/
public Optional ssoSecretType() {
return Optional.ofNullable(this.ssoSecretType);
}
/**
* @return URL to customer ADFS server for signing WVD SSO certificates.
*
*/
public Optional ssoadfsAuthority() {
return Optional.ofNullable(this.ssoadfsAuthority);
}
/**
* @return The flag to turn on/off StartVMOnConnect feature.
*
*/
public Optional startVMOnConnect() {
return Optional.ofNullable(this.startVMOnConnect);
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Is validation environment.
*
*/
public Optional validationEnvironment() {
return Optional.ofNullable(this.validationEnvironment);
}
/**
* @return VM template for sessionhosts configuration within hostpool.
*
*/
public Optional vmTemplate() {
return Optional.ofNullable(this.vmTemplate);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetHostPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AgentUpdatePropertiesResponse agentUpdate;
private List applicationGroupReferences;
private Boolean cloudPcResource;
private @Nullable String customRdpProperty;
private @Nullable String description;
private String etag;
private @Nullable String friendlyName;
private String hostPoolType;
private String id;
private @Nullable ResourceModelWithAllowedPropertySetResponseIdentity identity;
private @Nullable String kind;
private String loadBalancerType;
private @Nullable String location;
private @Nullable String managedBy;
private @Nullable Integer maxSessionLimit;
private String name;
private String objectId;
private @Nullable String personalDesktopAssignmentType;
private @Nullable ResourceModelWithAllowedPropertySetResponsePlan plan;
private String preferredAppGroupType;
private @Nullable RegistrationInfoResponse registrationInfo;
private @Nullable Integer ring;
private @Nullable ResourceModelWithAllowedPropertySetResponseSku sku;
private @Nullable String ssoClientId;
private @Nullable String ssoClientSecretKeyVaultPath;
private @Nullable String ssoSecretType;
private @Nullable String ssoadfsAuthority;
private @Nullable Boolean startVMOnConnect;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private @Nullable Boolean validationEnvironment;
private @Nullable String vmTemplate;
public Builder() {}
public Builder(GetHostPoolResult defaults) {
Objects.requireNonNull(defaults);
this.agentUpdate = defaults.agentUpdate;
this.applicationGroupReferences = defaults.applicationGroupReferences;
this.cloudPcResource = defaults.cloudPcResource;
this.customRdpProperty = defaults.customRdpProperty;
this.description = defaults.description;
this.etag = defaults.etag;
this.friendlyName = defaults.friendlyName;
this.hostPoolType = defaults.hostPoolType;
this.id = defaults.id;
this.identity = defaults.identity;
this.kind = defaults.kind;
this.loadBalancerType = defaults.loadBalancerType;
this.location = defaults.location;
this.managedBy = defaults.managedBy;
this.maxSessionLimit = defaults.maxSessionLimit;
this.name = defaults.name;
this.objectId = defaults.objectId;
this.personalDesktopAssignmentType = defaults.personalDesktopAssignmentType;
this.plan = defaults.plan;
this.preferredAppGroupType = defaults.preferredAppGroupType;
this.registrationInfo = defaults.registrationInfo;
this.ring = defaults.ring;
this.sku = defaults.sku;
this.ssoClientId = defaults.ssoClientId;
this.ssoClientSecretKeyVaultPath = defaults.ssoClientSecretKeyVaultPath;
this.ssoSecretType = defaults.ssoSecretType;
this.ssoadfsAuthority = defaults.ssoadfsAuthority;
this.startVMOnConnect = defaults.startVMOnConnect;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.validationEnvironment = defaults.validationEnvironment;
this.vmTemplate = defaults.vmTemplate;
}
@CustomType.Setter
public Builder agentUpdate(@Nullable AgentUpdatePropertiesResponse agentUpdate) {
this.agentUpdate = agentUpdate;
return this;
}
@CustomType.Setter
public Builder applicationGroupReferences(List applicationGroupReferences) {
if (applicationGroupReferences == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "applicationGroupReferences");
}
this.applicationGroupReferences = applicationGroupReferences;
return this;
}
public Builder applicationGroupReferences(String... applicationGroupReferences) {
return applicationGroupReferences(List.of(applicationGroupReferences));
}
@CustomType.Setter
public Builder cloudPcResource(Boolean cloudPcResource) {
if (cloudPcResource == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "cloudPcResource");
}
this.cloudPcResource = cloudPcResource;
return this;
}
@CustomType.Setter
public Builder customRdpProperty(@Nullable String customRdpProperty) {
this.customRdpProperty = customRdpProperty;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder friendlyName(@Nullable String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
@CustomType.Setter
public Builder hostPoolType(String hostPoolType) {
if (hostPoolType == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "hostPoolType");
}
this.hostPoolType = hostPoolType;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable ResourceModelWithAllowedPropertySetResponseIdentity identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder loadBalancerType(String loadBalancerType) {
if (loadBalancerType == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "loadBalancerType");
}
this.loadBalancerType = loadBalancerType;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedBy(@Nullable String managedBy) {
this.managedBy = managedBy;
return this;
}
@CustomType.Setter
public Builder maxSessionLimit(@Nullable Integer maxSessionLimit) {
this.maxSessionLimit = maxSessionLimit;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder objectId(String objectId) {
if (objectId == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "objectId");
}
this.objectId = objectId;
return this;
}
@CustomType.Setter
public Builder personalDesktopAssignmentType(@Nullable String personalDesktopAssignmentType) {
this.personalDesktopAssignmentType = personalDesktopAssignmentType;
return this;
}
@CustomType.Setter
public Builder plan(@Nullable ResourceModelWithAllowedPropertySetResponsePlan plan) {
this.plan = plan;
return this;
}
@CustomType.Setter
public Builder preferredAppGroupType(String preferredAppGroupType) {
if (preferredAppGroupType == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "preferredAppGroupType");
}
this.preferredAppGroupType = preferredAppGroupType;
return this;
}
@CustomType.Setter
public Builder registrationInfo(@Nullable RegistrationInfoResponse registrationInfo) {
this.registrationInfo = registrationInfo;
return this;
}
@CustomType.Setter
public Builder ring(@Nullable Integer ring) {
this.ring = ring;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable ResourceModelWithAllowedPropertySetResponseSku sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder ssoClientId(@Nullable String ssoClientId) {
this.ssoClientId = ssoClientId;
return this;
}
@CustomType.Setter
public Builder ssoClientSecretKeyVaultPath(@Nullable String ssoClientSecretKeyVaultPath) {
this.ssoClientSecretKeyVaultPath = ssoClientSecretKeyVaultPath;
return this;
}
@CustomType.Setter
public Builder ssoSecretType(@Nullable String ssoSecretType) {
this.ssoSecretType = ssoSecretType;
return this;
}
@CustomType.Setter
public Builder ssoadfsAuthority(@Nullable String ssoadfsAuthority) {
this.ssoadfsAuthority = ssoadfsAuthority;
return this;
}
@CustomType.Setter
public Builder startVMOnConnect(@Nullable Boolean startVMOnConnect) {
this.startVMOnConnect = startVMOnConnect;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetHostPoolResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder validationEnvironment(@Nullable Boolean validationEnvironment) {
this.validationEnvironment = validationEnvironment;
return this;
}
@CustomType.Setter
public Builder vmTemplate(@Nullable String vmTemplate) {
this.vmTemplate = vmTemplate;
return this;
}
public GetHostPoolResult build() {
final var _resultValue = new GetHostPoolResult();
_resultValue.agentUpdate = agentUpdate;
_resultValue.applicationGroupReferences = applicationGroupReferences;
_resultValue.cloudPcResource = cloudPcResource;
_resultValue.customRdpProperty = customRdpProperty;
_resultValue.description = description;
_resultValue.etag = etag;
_resultValue.friendlyName = friendlyName;
_resultValue.hostPoolType = hostPoolType;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.kind = kind;
_resultValue.loadBalancerType = loadBalancerType;
_resultValue.location = location;
_resultValue.managedBy = managedBy;
_resultValue.maxSessionLimit = maxSessionLimit;
_resultValue.name = name;
_resultValue.objectId = objectId;
_resultValue.personalDesktopAssignmentType = personalDesktopAssignmentType;
_resultValue.plan = plan;
_resultValue.preferredAppGroupType = preferredAppGroupType;
_resultValue.registrationInfo = registrationInfo;
_resultValue.ring = ring;
_resultValue.sku = sku;
_resultValue.ssoClientId = ssoClientId;
_resultValue.ssoClientSecretKeyVaultPath = ssoClientSecretKeyVaultPath;
_resultValue.ssoSecretType = ssoSecretType;
_resultValue.ssoadfsAuthority = ssoadfsAuthority;
_resultValue.startVMOnConnect = startVMOnConnect;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.validationEnvironment = validationEnvironment;
_resultValue.vmTemplate = vmTemplate;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy